mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-07-06 19:26:16 +09:00
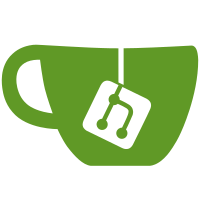
U-Boot has never cared about the type when we get max/min of two values, but Linux Kernel does. This commit gets min, max, min3, max3 macros synced with the kernel introducing type checks. Many of references of those macros must be fixed to suppress warnings. We have two options: - Use min, max, min3, max3 only when the arguments have the same type (or add casts to the arguments) - Use min_t/max_t instead with the appropriate type for the first argument Signed-off-by: Masahiro Yamada <yamada.m@jp.panasonic.com> Acked-by: Pavel Machek <pavel@denx.de> Acked-by: Lukasz Majewski <l.majewski@samsung.com> Tested-by: Lukasz Majewski <l.majewski@samsung.com> [trini: Fixup arch/blackfin/lib/string.c] Signed-off-by: Tom Rini <trini@ti.com>
124 lines
3.0 KiB
C
124 lines
3.0 KiB
C
/*
|
|
* Copyright (c) 2011 The Chromium OS Authors.
|
|
* (C) Copyright 2010,2011
|
|
* Graeme Russ, <graeme.russ@gmail.com>
|
|
*
|
|
* SPDX-License-Identifier: GPL-2.0+
|
|
*/
|
|
|
|
#include <common.h>
|
|
#include <malloc.h>
|
|
#include <asm/e820.h>
|
|
#include <asm/u-boot-x86.h>
|
|
#include <asm/global_data.h>
|
|
#include <asm/processor.h>
|
|
#include <asm/sections.h>
|
|
#include <asm/arch/sysinfo.h>
|
|
#include <asm/arch/tables.h>
|
|
|
|
DECLARE_GLOBAL_DATA_PTR;
|
|
|
|
unsigned install_e820_map(unsigned max_entries, struct e820entry *entries)
|
|
{
|
|
int i;
|
|
|
|
unsigned num_entries = min((unsigned)lib_sysinfo.n_memranges, max_entries);
|
|
if (num_entries < lib_sysinfo.n_memranges) {
|
|
printf("Warning: Limiting e820 map to %d entries.\n",
|
|
num_entries);
|
|
}
|
|
for (i = 0; i < num_entries; i++) {
|
|
struct memrange *memrange = &lib_sysinfo.memrange[i];
|
|
|
|
entries[i].addr = memrange->base;
|
|
entries[i].size = memrange->size;
|
|
entries[i].type = memrange->type;
|
|
}
|
|
return num_entries;
|
|
}
|
|
|
|
/*
|
|
* This function looks for the highest region of memory lower than 4GB which
|
|
* has enough space for U-Boot where U-Boot is aligned on a page boundary. It
|
|
* overrides the default implementation found elsewhere which simply picks the
|
|
* end of ram, wherever that may be. The location of the stack, the relocation
|
|
* address, and how far U-Boot is moved by relocation are set in the global
|
|
* data structure.
|
|
*/
|
|
ulong board_get_usable_ram_top(ulong total_size)
|
|
{
|
|
uintptr_t dest_addr = 0;
|
|
int i;
|
|
|
|
for (i = 0; i < lib_sysinfo.n_memranges; i++) {
|
|
struct memrange *memrange = &lib_sysinfo.memrange[i];
|
|
/* Force U-Boot to relocate to a page aligned address. */
|
|
uint64_t start = roundup(memrange->base, 1 << 12);
|
|
uint64_t end = memrange->base + memrange->size;
|
|
|
|
/* Ignore non-memory regions. */
|
|
if (memrange->type != CB_MEM_RAM)
|
|
continue;
|
|
|
|
/* Filter memory over 4GB. */
|
|
if (end > 0xffffffffULL)
|
|
end = 0x100000000ULL;
|
|
/* Skip this region if it's too small. */
|
|
if (end - start < total_size)
|
|
continue;
|
|
|
|
/* Use this address if it's the largest so far. */
|
|
if (end > dest_addr)
|
|
dest_addr = end;
|
|
}
|
|
|
|
/* If no suitable area was found, return an error. */
|
|
if (!dest_addr)
|
|
panic("No available memory found for relocation");
|
|
|
|
return (ulong)dest_addr;
|
|
}
|
|
|
|
int dram_init_f(void)
|
|
{
|
|
int i;
|
|
phys_size_t ram_size = 0;
|
|
|
|
for (i = 0; i < lib_sysinfo.n_memranges; i++) {
|
|
struct memrange *memrange = &lib_sysinfo.memrange[i];
|
|
unsigned long long end = memrange->base + memrange->size;
|
|
|
|
if (memrange->type == CB_MEM_RAM && end > ram_size)
|
|
ram_size = end;
|
|
}
|
|
gd->ram_size = ram_size;
|
|
if (ram_size == 0)
|
|
return -1;
|
|
return 0;
|
|
}
|
|
|
|
int dram_init_banksize(void)
|
|
{
|
|
int i, j;
|
|
|
|
if (CONFIG_NR_DRAM_BANKS) {
|
|
for (i = 0, j = 0; i < lib_sysinfo.n_memranges; i++) {
|
|
struct memrange *memrange = &lib_sysinfo.memrange[i];
|
|
|
|
if (memrange->type == CB_MEM_RAM) {
|
|
gd->bd->bi_dram[j].start = memrange->base;
|
|
gd->bd->bi_dram[j].size = memrange->size;
|
|
j++;
|
|
if (j >= CONFIG_NR_DRAM_BANKS)
|
|
break;
|
|
}
|
|
}
|
|
}
|
|
return 0;
|
|
}
|
|
|
|
int dram_init(void)
|
|
{
|
|
return dram_init_banksize();
|
|
}
|