mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-07-12 06:06:15 +09:00
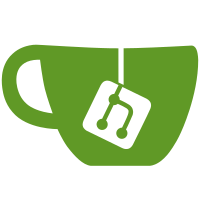
Per PCI spec, VGA device reports its class as standard 030000h in its configuration space, so we can use it to determine if we need run option rom instead of testing the supported vendor/device ids. Signed-off-by: Bin Meng <bmeng.cn@gmail.com> Acked-by: Simon Glass <sjg@chromium.org>
56 lines
1.1 KiB
C
56 lines
1.1 KiB
C
/*
|
|
* VESA frame buffer driver
|
|
*
|
|
* Copyright (C) 2014 Google, Inc
|
|
*
|
|
* SPDX-License-Identifier: GPL-2.0+
|
|
*/
|
|
|
|
#include <common.h>
|
|
#include <pci_rom.h>
|
|
#include <video_fb.h>
|
|
#include <vbe.h>
|
|
|
|
/*
|
|
* The Graphic Device
|
|
*/
|
|
GraphicDevice ctfb;
|
|
|
|
void *video_hw_init(void)
|
|
{
|
|
GraphicDevice *gdev = &ctfb;
|
|
int bits_per_pixel;
|
|
pci_dev_t dev;
|
|
int ret;
|
|
|
|
printf("Video: ");
|
|
if (vbe_get_video_info(gdev)) {
|
|
dev = pci_find_class(PCI_CLASS_DISPLAY_VGA << 8, 0);
|
|
if (dev == -1) {
|
|
printf("no card detected\n");
|
|
return NULL;
|
|
}
|
|
bootstage_start(BOOTSTAGE_ID_ACCUM_LCD, "vesa display");
|
|
ret = pci_run_vga_bios(dev, NULL, PCI_ROM_USE_NATIVE |
|
|
PCI_ROM_ALLOW_FALLBACK);
|
|
bootstage_accum(BOOTSTAGE_ID_ACCUM_LCD);
|
|
if (ret) {
|
|
printf("failed to run video BIOS: %d\n", ret);
|
|
return NULL;
|
|
}
|
|
}
|
|
|
|
if (vbe_get_video_info(gdev)) {
|
|
printf("No video mode configured\n");
|
|
return NULL;
|
|
}
|
|
|
|
bits_per_pixel = gdev->gdfBytesPP * 8;
|
|
sprintf(gdev->modeIdent, "%dx%dx%d", gdev->winSizeX, gdev->winSizeY,
|
|
bits_per_pixel);
|
|
printf("%s\n", gdev->modeIdent);
|
|
debug("Frame buffer at %x\n", gdev->pciBase);
|
|
|
|
return (void *)gdev;
|
|
}
|