mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-07-27 21:33:44 +09:00
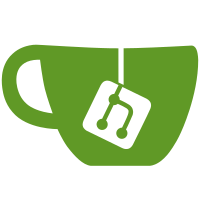
We try hard to make sure that SMBIOS tables live in the lower 32bit. However, when we can not find any space at all there, we should not error out but instead just fall back to map them in the full address space instead. This can for example happen on systems that do not have any RAM mapped in the lower 32bits of address space. In that case having any SMBIOS tables at all is better than having none. Signed-off-by: Alexander Graf <agraf@suse.de>
51 lines
1.2 KiB
C
51 lines
1.2 KiB
C
// SPDX-License-Identifier: GPL-2.0+
|
|
/*
|
|
* EFI application tables support
|
|
*
|
|
* Copyright (c) 2016 Alexander Graf
|
|
*/
|
|
|
|
#include <common.h>
|
|
#include <efi_loader.h>
|
|
#include <inttypes.h>
|
|
#include <smbios.h>
|
|
|
|
static const efi_guid_t smbios_guid = SMBIOS_TABLE_GUID;
|
|
|
|
/*
|
|
* Install the SMBIOS table as a configuration table.
|
|
*
|
|
* @return status code
|
|
*/
|
|
efi_status_t efi_smbios_register(void)
|
|
{
|
|
/* Map within the low 32 bits, to allow for 32bit SMBIOS tables */
|
|
u64 dmi = U32_MAX;
|
|
efi_status_t ret;
|
|
|
|
/* Reserve 4kiB page for SMBIOS */
|
|
ret = efi_allocate_pages(EFI_ALLOCATE_MAX_ADDRESS,
|
|
EFI_RUNTIME_SERVICES_DATA, 1, &dmi);
|
|
|
|
if (ret != EFI_SUCCESS) {
|
|
/* Could not find space in lowmem, use highmem instead */
|
|
ret = efi_allocate_pages(EFI_ALLOCATE_ANY_PAGES,
|
|
EFI_RUNTIME_SERVICES_DATA, 1, &dmi);
|
|
|
|
if (ret != EFI_SUCCESS)
|
|
return ret;
|
|
}
|
|
|
|
/*
|
|
* Generate SMBIOS tables - we know that efi_allocate_pages() returns
|
|
* a 4k-aligned address, so it is safe to assume that
|
|
* write_smbios_table() will write the table at that address.
|
|
*/
|
|
assert(!(dmi & 0xf));
|
|
write_smbios_table(dmi);
|
|
|
|
/* And expose them to our EFI payload */
|
|
return efi_install_configuration_table(&smbios_guid,
|
|
(void *)(uintptr_t)dmi);
|
|
}
|