mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-06-09 23:36:03 +09:00
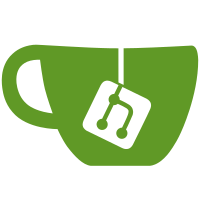
When U-Boot started using SPDX tags we were among the early adopters and there weren't a lot of other examples to borrow from. So we picked the area of the file that usually had a full license text and replaced it with an appropriate SPDX-License-Identifier: entry. Since then, the Linux Kernel has adopted SPDX tags and they place it as the very first line in a file (except where shebangs are used, then it's second line) and with slightly different comment styles than us. In part due to community overlap, in part due to better tag visibility and in part for other minor reasons, switch over to that style. This commit changes all instances where we have a single declared license in the tag as both the before and after are identical in tag contents. There's also a few places where I found we did not have a tag and have introduced one. Signed-off-by: Tom Rini <trini@konsulko.com>
169 lines
3.9 KiB
C
169 lines
3.9 KiB
C
// SPDX-License-Identifier: GPL-2.0
|
|
/*
|
|
* Copyright (C) Marvell International Ltd. and its affiliates
|
|
*/
|
|
|
|
#include <common.h>
|
|
#include <spl.h>
|
|
#include <asm/io.h>
|
|
#include <asm/arch/cpu.h>
|
|
#include <asm/arch/soc.h>
|
|
|
|
#include "seq_exec.h"
|
|
#include "high_speed_env_spec.h"
|
|
|
|
#include "../../../drivers/ddr/marvell/a38x/ddr3_init.h"
|
|
|
|
#if defined(MV_DEBUG_INIT_FULL) || defined(MV_DEBUG)
|
|
#define DB(x) x
|
|
#else
|
|
#define DB(x)
|
|
#endif
|
|
|
|
/* Array for mapping the operation (write, poll or delay) functions */
|
|
op_execute_func_ptr op_execute_func_arr[] = {
|
|
write_op_execute,
|
|
delay_op_execute,
|
|
poll_op_execute
|
|
};
|
|
|
|
int write_op_execute(u32 serdes_num, struct op_params *params, u32 data_arr_idx)
|
|
{
|
|
u32 unit_base_reg, unit_offset, data, mask, reg_data, reg_addr;
|
|
|
|
/* Getting write op params from the input parameter */
|
|
data = params->data[data_arr_idx];
|
|
mask = params->mask;
|
|
|
|
/* an empty operation */
|
|
if (data == NO_DATA)
|
|
return MV_OK;
|
|
|
|
/* get updated base address since it can be different between Serdes */
|
|
CHECK_STATUS(hws_get_ext_base_addr(serdes_num, params->unit_base_reg,
|
|
params->unit_offset,
|
|
&unit_base_reg, &unit_offset));
|
|
|
|
/* Address calculation */
|
|
reg_addr = unit_base_reg + unit_offset * serdes_num;
|
|
|
|
#ifdef SEQ_DEBUG
|
|
printf("Write: 0x%x: 0x%x (mask 0x%x) - ", reg_addr, data, mask);
|
|
#endif
|
|
/* Reading old value */
|
|
reg_data = reg_read(reg_addr);
|
|
reg_data &= (~mask);
|
|
|
|
/* Writing new data */
|
|
data &= mask;
|
|
reg_data |= data;
|
|
reg_write(reg_addr, reg_data);
|
|
|
|
#ifdef SEQ_DEBUG
|
|
printf(" - 0x%x\n", reg_data);
|
|
#endif
|
|
|
|
return MV_OK;
|
|
}
|
|
|
|
int delay_op_execute(u32 serdes_num, struct op_params *params, u32 data_arr_idx)
|
|
{
|
|
u32 delay;
|
|
|
|
/* Getting delay op params from the input parameter */
|
|
delay = params->wait_time;
|
|
#ifdef SEQ_DEBUG
|
|
printf("Delay: %d\n", delay);
|
|
#endif
|
|
mdelay(delay);
|
|
|
|
return MV_OK;
|
|
}
|
|
|
|
int poll_op_execute(u32 serdes_num, struct op_params *params, u32 data_arr_idx)
|
|
{
|
|
u32 unit_base_reg, unit_offset, data, mask, num_of_loops, wait_time;
|
|
u32 poll_counter = 0;
|
|
u32 reg_addr, reg_data;
|
|
|
|
/* Getting poll op params from the input parameter */
|
|
data = params->data[data_arr_idx];
|
|
mask = params->mask;
|
|
num_of_loops = params->num_of_loops;
|
|
wait_time = params->wait_time;
|
|
|
|
/* an empty operation */
|
|
if (data == NO_DATA)
|
|
return MV_OK;
|
|
|
|
/* get updated base address since it can be different between Serdes */
|
|
CHECK_STATUS(hws_get_ext_base_addr(serdes_num, params->unit_base_reg,
|
|
params->unit_offset,
|
|
&unit_base_reg, &unit_offset));
|
|
|
|
/* Address calculation */
|
|
reg_addr = unit_base_reg + unit_offset * serdes_num;
|
|
|
|
/* Polling */
|
|
#ifdef SEQ_DEBUG
|
|
printf("Poll: 0x%x: 0x%x (mask 0x%x)\n", reg_addr, data, mask);
|
|
#endif
|
|
|
|
do {
|
|
reg_data = reg_read(reg_addr) & mask;
|
|
poll_counter++;
|
|
udelay(wait_time);
|
|
} while ((reg_data != data) && (poll_counter < num_of_loops));
|
|
|
|
if ((poll_counter >= num_of_loops) && (reg_data != data)) {
|
|
DEBUG_INIT_S("poll_op_execute: TIMEOUT\n");
|
|
return MV_TIMEOUT;
|
|
}
|
|
|
|
return MV_OK;
|
|
}
|
|
|
|
enum mv_op get_cfg_seq_op(struct op_params *params)
|
|
{
|
|
if (params->wait_time == 0)
|
|
return WRITE_OP;
|
|
else if (params->num_of_loops == 0)
|
|
return DELAY_OP;
|
|
|
|
return POLL_OP;
|
|
}
|
|
|
|
int mv_seq_exec(u32 serdes_num, u32 seq_id)
|
|
{
|
|
u32 seq_idx;
|
|
struct op_params *seq_arr;
|
|
u32 seq_size;
|
|
u32 data_arr_idx;
|
|
enum mv_op curr_op;
|
|
|
|
DB(printf("\n### mv_seq_exec ###\n"));
|
|
DB(printf("seq id: %d\n", seq_id));
|
|
|
|
if (hws_is_serdes_active(serdes_num) != 1) {
|
|
printf("mv_seq_exec_ext:Error: SerDes lane %d is not valid\n",
|
|
serdes_num);
|
|
return MV_BAD_PARAM;
|
|
}
|
|
|
|
seq_arr = serdes_seq_db[seq_id].op_params_ptr;
|
|
seq_size = serdes_seq_db[seq_id].cfg_seq_size;
|
|
data_arr_idx = serdes_seq_db[seq_id].data_arr_idx;
|
|
|
|
DB(printf("seq_size: %d\n", seq_size));
|
|
DB(printf("data_arr_idx: %d\n", data_arr_idx));
|
|
|
|
/* Executing the sequence operations */
|
|
for (seq_idx = 0; seq_idx < seq_size; seq_idx++) {
|
|
curr_op = get_cfg_seq_op(&seq_arr[seq_idx]);
|
|
op_execute_func_arr[curr_op](serdes_num, &seq_arr[seq_idx],
|
|
data_arr_idx);
|
|
}
|
|
|
|
return MV_OK;
|
|
}
|