mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-07-05 02:36:39 +09:00
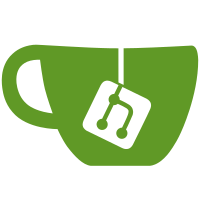
Add a new uclass for TPMs which uses almost the same TIS (TPM Interface Specification) as is currently implemented. Since init() is handled by the normal driver model probe() method, we don't need to implement that. Also rename the transfer method to xfer() which is a less clumbsy name. Once all drivers and users are converted to driver model we can remove the old code. Signed-off-by: Simon Glass <sjg@chromium.org> Acked-by: Christophe Ricard<christophe-h.ricard@st.com> Reviewed-by: Heiko Schocher <hs@denx.de>
165 lines
3.4 KiB
C
165 lines
3.4 KiB
C
/*
|
|
* Copyright (C) 2011 Infineon Technologies
|
|
*
|
|
* Authors:
|
|
* Peter Huewe <huewe.external@infineon.com>
|
|
*
|
|
* Version: 2.1.1
|
|
*
|
|
* Description:
|
|
* Device driver for TCG/TCPA TPM (trusted platform module).
|
|
* Specifications at www.trustedcomputinggroup.org
|
|
*
|
|
* It is based on the Linux kernel driver tpm.c from Leendert van
|
|
* Dorn, Dave Safford, Reiner Sailer, and Kyleen Hall.
|
|
*
|
|
* SPDX-License-Identifier: GPL-2.0
|
|
*/
|
|
|
|
#ifndef _TPM_TIS_I2C_H
|
|
#define _TPM_TIS_I2C_H
|
|
|
|
#include <linux/compiler.h>
|
|
#include <linux/types.h>
|
|
|
|
enum tpm_timeout {
|
|
TPM_TIMEOUT_MS = 5,
|
|
TIS_SHORT_TIMEOUT_MS = 750,
|
|
TIS_LONG_TIMEOUT_MS = 2000,
|
|
SLEEP_DURATION_US = 60,
|
|
SLEEP_DURATION_LONG_US = 210,
|
|
};
|
|
|
|
/* Size of external transmit buffer (used in tpm_transmit)*/
|
|
#define TPM_BUFSIZE 4096
|
|
|
|
/* Index of Count field in TPM response buffer */
|
|
#define TPM_RSP_SIZE_BYTE 2
|
|
#define TPM_RSP_RC_BYTE 6
|
|
|
|
/* Max buffer size supported by our tpm */
|
|
#define TPM_DEV_BUFSIZE 1260
|
|
|
|
enum i2c_chip_type {
|
|
SLB9635,
|
|
SLB9645,
|
|
UNKNOWN,
|
|
};
|
|
|
|
struct tpm_chip {
|
|
bool inited;
|
|
int is_open;
|
|
u8 req_complete_mask;
|
|
u8 req_complete_val;
|
|
u8 req_canceled;
|
|
int irq;
|
|
int locality;
|
|
unsigned long timeout_a, timeout_b, timeout_c, timeout_d; /* msec */
|
|
unsigned long duration[3]; /* msec */
|
|
struct udevice *dev;
|
|
u8 buf[TPM_DEV_BUFSIZE + sizeof(u8)]; /* Max buffer size + addr */
|
|
enum i2c_chip_type chip_type;
|
|
};
|
|
|
|
struct tpm_input_header {
|
|
__be16 tag;
|
|
__be32 length;
|
|
__be32 ordinal;
|
|
} __packed;
|
|
|
|
struct tpm_output_header {
|
|
__be16 tag;
|
|
__be32 length;
|
|
__be32 return_code;
|
|
} __packed;
|
|
|
|
struct timeout_t {
|
|
__be32 a;
|
|
__be32 b;
|
|
__be32 c;
|
|
__be32 d;
|
|
} __packed;
|
|
|
|
struct duration_t {
|
|
__be32 tpm_short;
|
|
__be32 tpm_medium;
|
|
__be32 tpm_long;
|
|
} __packed;
|
|
|
|
union cap_t {
|
|
struct timeout_t timeout;
|
|
struct duration_t duration;
|
|
};
|
|
|
|
struct tpm_getcap_params_in {
|
|
__be32 cap;
|
|
__be32 subcap_size;
|
|
__be32 subcap;
|
|
} __packed;
|
|
|
|
struct tpm_getcap_params_out {
|
|
__be32 cap_size;
|
|
union cap_t cap;
|
|
} __packed;
|
|
|
|
union tpm_cmd_header {
|
|
struct tpm_input_header in;
|
|
struct tpm_output_header out;
|
|
};
|
|
|
|
union tpm_cmd_params {
|
|
struct tpm_getcap_params_out getcap_out;
|
|
struct tpm_getcap_params_in getcap_in;
|
|
};
|
|
|
|
struct tpm_cmd_t {
|
|
union tpm_cmd_header header;
|
|
union tpm_cmd_params params;
|
|
} __packed;
|
|
|
|
/* Max number of iterations after i2c NAK */
|
|
#define MAX_COUNT 3
|
|
|
|
/*
|
|
* Max number of iterations after i2c NAK for 'long' commands
|
|
*
|
|
* We need this especially for sending TPM_READY, since the cleanup after the
|
|
* transtion to the ready state may take some time, but it is unpredictable
|
|
* how long it will take.
|
|
*/
|
|
#define MAX_COUNT_LONG 50
|
|
|
|
#define TPM_HEADER_SIZE 10
|
|
|
|
enum tis_access {
|
|
TPM_ACCESS_VALID = 0x80,
|
|
TPM_ACCESS_ACTIVE_LOCALITY = 0x20,
|
|
TPM_ACCESS_REQUEST_PENDING = 0x04,
|
|
TPM_ACCESS_REQUEST_USE = 0x02,
|
|
};
|
|
|
|
enum tis_status {
|
|
TPM_STS_VALID = 0x80,
|
|
TPM_STS_COMMAND_READY = 0x40,
|
|
TPM_STS_GO = 0x20,
|
|
TPM_STS_DATA_AVAIL = 0x10,
|
|
TPM_STS_DATA_EXPECT = 0x08,
|
|
};
|
|
|
|
/* expected value for DIDVID register */
|
|
#define TPM_TIS_I2C_DID_VID_9635 0x000b15d1L
|
|
#define TPM_TIS_I2C_DID_VID_9645 0x001a15d1L
|
|
|
|
#define TPM_ACCESS(l) (0x0000 | ((l) << 4))
|
|
#define TPM_STS(l) (0x0001 | ((l) << 4))
|
|
#define TPM_DATA_FIFO(l) (0x0005 | ((l) << 4))
|
|
#define TPM_DID_VID(l) (0x0006 | ((l) << 4))
|
|
|
|
/* Extended error numbers from linux (see errno.h) */
|
|
#define ECANCELED 125 /* Operation Canceled */
|
|
|
|
/* Timer frequency. Corresponds to msec timer resolution */
|
|
#define HZ 1000
|
|
|
|
#endif
|