mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-07-24 11:59:46 +09:00
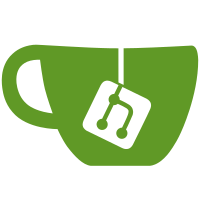
When SoC first boots up, we should invalidate the cache but not flush it. We can use the same function for invalid and flush mostly, with a wrapper. Invalidating large cache can ben slow on emulator, so we postpone doing so until I-cache is enabled, and before enabling D-cache. Signed-off-by: York Sun <yorksun@freescale.com> CC: David Feng <fenghua@phytium.com.cn>
139 lines
2.6 KiB
ArmAsm
139 lines
2.6 KiB
ArmAsm
/*
|
|
* (C) Copyright 2013
|
|
* David Feng <fenghua@phytium.com.cn>
|
|
*
|
|
* SPDX-License-Identifier: GPL-2.0+
|
|
*/
|
|
|
|
#include <asm-offsets.h>
|
|
#include <config.h>
|
|
#include <version.h>
|
|
#include <linux/linkage.h>
|
|
#include <asm/macro.h>
|
|
#include <asm/armv8/mmu.h>
|
|
|
|
/*************************************************************************
|
|
*
|
|
* Startup Code (reset vector)
|
|
*
|
|
*************************************************************************/
|
|
|
|
.globl _start
|
|
_start:
|
|
b reset
|
|
|
|
.align 3
|
|
|
|
.globl _TEXT_BASE
|
|
_TEXT_BASE:
|
|
.quad CONFIG_SYS_TEXT_BASE
|
|
|
|
/*
|
|
* These are defined in the linker script.
|
|
*/
|
|
.globl _end_ofs
|
|
_end_ofs:
|
|
.quad _end - _start
|
|
|
|
.globl _bss_start_ofs
|
|
_bss_start_ofs:
|
|
.quad __bss_start - _start
|
|
|
|
.globl _bss_end_ofs
|
|
_bss_end_ofs:
|
|
.quad __bss_end - _start
|
|
|
|
reset:
|
|
/*
|
|
* Could be EL3/EL2/EL1, Initial State:
|
|
* Little Endian, MMU Disabled, i/dCache Disabled
|
|
*/
|
|
adr x0, vectors
|
|
switch_el x1, 3f, 2f, 1f
|
|
3: msr vbar_el3, x0
|
|
msr cptr_el3, xzr /* Enable FP/SIMD */
|
|
ldr x0, =COUNTER_FREQUENCY
|
|
msr cntfrq_el0, x0 /* Initialize CNTFRQ */
|
|
b 0f
|
|
2: msr vbar_el2, x0
|
|
mov x0, #0x33ff
|
|
msr cptr_el2, x0 /* Enable FP/SIMD */
|
|
b 0f
|
|
1: msr vbar_el1, x0
|
|
mov x0, #3 << 20
|
|
msr cpacr_el1, x0 /* Enable FP/SIMD */
|
|
0:
|
|
|
|
/*
|
|
* Cache/BPB/TLB Invalidate
|
|
* i-cache is invalidated before enabled in icache_enable()
|
|
* tlb is invalidated before mmu is enabled in dcache_enable()
|
|
* d-cache is invalidated before enabled in dcache_enable()
|
|
*/
|
|
|
|
/* Processor specific initialization */
|
|
bl lowlevel_init
|
|
|
|
branch_if_master x0, x1, master_cpu
|
|
|
|
/*
|
|
* Slave CPUs
|
|
*/
|
|
slave_cpu:
|
|
wfe
|
|
ldr x1, =CPU_RELEASE_ADDR
|
|
ldr x0, [x1]
|
|
cbz x0, slave_cpu
|
|
br x0 /* branch to the given address */
|
|
|
|
/*
|
|
* Master CPU
|
|
*/
|
|
master_cpu:
|
|
bl _main
|
|
|
|
/*-----------------------------------------------------------------------*/
|
|
|
|
WEAK(lowlevel_init)
|
|
/* Initialize GIC Secure Bank Status */
|
|
mov x29, lr /* Save LR */
|
|
bl gic_init
|
|
|
|
branch_if_master x0, x1, 1f
|
|
|
|
/*
|
|
* Slave should wait for master clearing spin table.
|
|
* This sync prevent salves observing incorrect
|
|
* value of spin table and jumping to wrong place.
|
|
*/
|
|
bl wait_for_wakeup
|
|
|
|
/*
|
|
* All processors will enter EL2 and optionally EL1.
|
|
*/
|
|
bl armv8_switch_to_el2
|
|
#ifdef CONFIG_ARMV8_SWITCH_TO_EL1
|
|
bl armv8_switch_to_el1
|
|
#endif
|
|
|
|
1:
|
|
mov lr, x29 /* Restore LR */
|
|
ret
|
|
ENDPROC(lowlevel_init)
|
|
|
|
/*-----------------------------------------------------------------------*/
|
|
|
|
ENTRY(c_runtime_cpu_setup)
|
|
/* Relocate vBAR */
|
|
adr x0, vectors
|
|
switch_el x1, 3f, 2f, 1f
|
|
3: msr vbar_el3, x0
|
|
b 0f
|
|
2: msr vbar_el2, x0
|
|
b 0f
|
|
1: msr vbar_el1, x0
|
|
0:
|
|
|
|
ret
|
|
ENDPROC(c_runtime_cpu_setup)
|