mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-06-09 23:36:03 +09:00
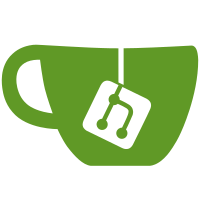
All Calxeda machines are actually a poster book example of device tree usage: the DT is loaded from flash by the management processor into DRAM, the memory node is populated with the detected DRAM size and this DT is then handed over to the kernel. So it's a shame that U-Boot didn't participate in this chain, but fortunately this is easy to fix: Define CONFIG_OF_CONTROL and CONFIG_OF_BOARD, and provide a trivial function to tell U-Boot about the (fixed) location of the DTB in DRAM. Then enable DM_SERIAL, to let the PL011 driver pick up the UART platform data from the DT. Also define AHCI, to bring this driver into the driver model world as well. Signed-off-by: Andre Przywara <andre.przywara@arm.com>
160 lines
3.1 KiB
C
160 lines
3.1 KiB
C
// SPDX-License-Identifier: GPL-2.0+
|
|
/*
|
|
* Copyright 2010-2011 Calxeda, Inc.
|
|
*/
|
|
|
|
#include <common.h>
|
|
#include <ahci.h>
|
|
#include <cpu_func.h>
|
|
#include <env.h>
|
|
#include <fdt_support.h>
|
|
#include <init.h>
|
|
#include <net.h>
|
|
#include <netdev.h>
|
|
#include <scsi.h>
|
|
#include <asm/global_data.h>
|
|
|
|
#include <linux/sizes.h>
|
|
#include <asm/io.h>
|
|
|
|
#define HB_AHCI_BASE 0xffe08000
|
|
|
|
#define HB_SCU_A9_PWR_STATUS 0xfff10008
|
|
#define HB_SREG_A9_PWR_REQ 0xfff3cf00
|
|
#define HB_SREG_A9_BOOT_SRC_STAT 0xfff3cf04
|
|
#define HB_SREG_A9_PWRDOM_STAT 0xfff3cf20
|
|
#define HB_SREG_A15_PWR_CTRL 0xfff3c200
|
|
|
|
#define HB_PWR_SUSPEND 0
|
|
#define HB_PWR_SOFT_RESET 1
|
|
#define HB_PWR_HARD_RESET 2
|
|
#define HB_PWR_SHUTDOWN 3
|
|
|
|
#define PWRDOM_STAT_SATA 0x80000000
|
|
#define PWRDOM_STAT_PCI 0x40000000
|
|
#define PWRDOM_STAT_EMMC 0x20000000
|
|
|
|
#define HB_SCU_A9_PWR_NORMAL 0
|
|
#define HB_SCU_A9_PWR_DORMANT 2
|
|
#define HB_SCU_A9_PWR_OFF 3
|
|
|
|
DECLARE_GLOBAL_DATA_PTR;
|
|
|
|
void cphy_disable_overrides(void);
|
|
|
|
/*
|
|
* Miscellaneous platform dependent initialisations
|
|
*/
|
|
int board_init(void)
|
|
{
|
|
icache_enable();
|
|
|
|
return 0;
|
|
}
|
|
|
|
/* We know all the init functions have been run now */
|
|
int board_eth_init(struct bd_info *bis)
|
|
{
|
|
int rc = 0;
|
|
|
|
#ifdef CONFIG_CALXEDA_XGMAC
|
|
rc += calxedaxgmac_initialize(0, 0xfff50000);
|
|
rc += calxedaxgmac_initialize(1, 0xfff51000);
|
|
#endif
|
|
return rc;
|
|
}
|
|
|
|
#ifdef CONFIG_SCSI_AHCI_PLAT
|
|
void scsi_init(void)
|
|
{
|
|
u32 reg = readl(HB_SREG_A9_PWRDOM_STAT);
|
|
|
|
cphy_disable_overrides();
|
|
if (reg & PWRDOM_STAT_SATA) {
|
|
ahci_init((void __iomem *)HB_AHCI_BASE);
|
|
scsi_scan(true);
|
|
}
|
|
}
|
|
#endif
|
|
|
|
#ifdef CONFIG_MISC_INIT_R
|
|
int misc_init_r(void)
|
|
{
|
|
char envbuffer[16];
|
|
u32 boot_choice;
|
|
|
|
boot_choice = readl(HB_SREG_A9_BOOT_SRC_STAT) & 0xff;
|
|
sprintf(envbuffer, "bootcmd%d", boot_choice);
|
|
if (env_get(envbuffer)) {
|
|
sprintf(envbuffer, "run bootcmd%d", boot_choice);
|
|
env_set("bootcmd", envbuffer);
|
|
} else
|
|
env_set("bootcmd", "");
|
|
|
|
return 0;
|
|
}
|
|
#endif
|
|
|
|
int dram_init(void)
|
|
{
|
|
gd->ram_size = SZ_512M;
|
|
return 0;
|
|
}
|
|
|
|
#if defined(CONFIG_OF_BOARD_SETUP)
|
|
int ft_board_setup(void *fdt, struct bd_info *bd)
|
|
{
|
|
static const char disabled[] = "disabled";
|
|
u32 reg = readl(HB_SREG_A9_PWRDOM_STAT);
|
|
|
|
if (!(reg & PWRDOM_STAT_SATA))
|
|
do_fixup_by_compat(fdt, "calxeda,hb-ahci", "status",
|
|
disabled, sizeof(disabled), 1);
|
|
|
|
if (!(reg & PWRDOM_STAT_EMMC))
|
|
do_fixup_by_compat(fdt, "calxeda,hb-sdhci", "status",
|
|
disabled, sizeof(disabled), 1);
|
|
|
|
return 0;
|
|
}
|
|
#endif
|
|
|
|
void *board_fdt_blob_setup(void)
|
|
{
|
|
/*
|
|
* The ECME management processor loads the DTB from NOR flash
|
|
* into DRAM (at 4KB), where it gets patched to contain the
|
|
* detected memory size.
|
|
*/
|
|
return (void *)0x1000;
|
|
}
|
|
|
|
static int is_highbank(void)
|
|
{
|
|
uint32_t midr;
|
|
|
|
asm volatile ("mrc p15, 0, %0, c0, c0, 0\n" : "=r"(midr));
|
|
|
|
return (midr & 0xfff0) == 0xc090;
|
|
}
|
|
|
|
void reset_cpu(void)
|
|
{
|
|
writel(HB_PWR_HARD_RESET, HB_SREG_A9_PWR_REQ);
|
|
if (is_highbank())
|
|
writeb(HB_SCU_A9_PWR_OFF, HB_SCU_A9_PWR_STATUS);
|
|
else
|
|
writel(0x1, HB_SREG_A15_PWR_CTRL);
|
|
|
|
wfi();
|
|
}
|
|
|
|
/*
|
|
* turn off the override before transferring control to Linux, since Linux
|
|
* may not support spread spectrum.
|
|
*/
|
|
void arch_preboot_os(void)
|
|
{
|
|
cphy_disable_overrides();
|
|
}
|