mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-06-09 23:36:03 +09:00
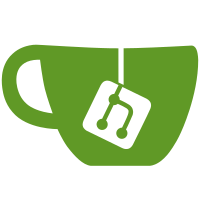
Part of the env cleanup moved this out of the environment code and into the net code. However, this helper is sometimes needed even when the net stack isn't included. Move the helper to lib/net_utils.c like it's similarly-purposed string_to_ip(). Also rename the moved function to similar naming. Signed-off-by: Joe Hershberger <joe.hershberger@ni.com> Reported-by: Ondrej Jirman <megous@megous.com>
59 lines
1.0 KiB
C
59 lines
1.0 KiB
C
// SPDX-License-Identifier: GPL-2.0+
|
|
/*
|
|
* Generic network code. Moved from net.c
|
|
*
|
|
* Copyright 1994 - 2000 Neil Russell.
|
|
* Copyright 2000 Roland Borde
|
|
* Copyright 2000 Paolo Scaffardi
|
|
* Copyright 2000-2002 Wolfgang Denk, wd@denx.de
|
|
* Copyright 2009 Dirk Behme, dirk.behme@googlemail.com
|
|
*/
|
|
|
|
#include <common.h>
|
|
|
|
struct in_addr string_to_ip(const char *s)
|
|
{
|
|
struct in_addr addr;
|
|
char *e;
|
|
int i;
|
|
|
|
addr.s_addr = 0;
|
|
if (s == NULL)
|
|
return addr;
|
|
|
|
for (addr.s_addr = 0, i = 0; i < 4; ++i) {
|
|
ulong val = s ? simple_strtoul(s, &e, 10) : 0;
|
|
if (val > 255) {
|
|
addr.s_addr = 0;
|
|
return addr;
|
|
}
|
|
if (i != 3 && *e != '.') {
|
|
addr.s_addr = 0;
|
|
return addr;
|
|
}
|
|
addr.s_addr <<= 8;
|
|
addr.s_addr |= (val & 0xFF);
|
|
if (s) {
|
|
s = (*e) ? e+1 : e;
|
|
}
|
|
}
|
|
|
|
addr.s_addr = htonl(addr.s_addr);
|
|
return addr;
|
|
}
|
|
|
|
void string_to_enetaddr(const char *addr, uint8_t *enetaddr)
|
|
{
|
|
char *end;
|
|
int i;
|
|
|
|
if (!enetaddr)
|
|
return;
|
|
|
|
for (i = 0; i < 6; ++i) {
|
|
enetaddr[i] = addr ? simple_strtoul(addr, &end, 16) : 0;
|
|
if (addr)
|
|
addr = (*end) ? end + 1 : end;
|
|
}
|
|
}
|