mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-06-09 23:36:03 +09:00
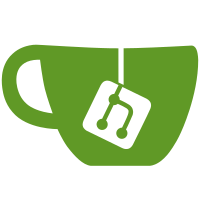
Add another flag to the DM core which could be assigned to drivers and which makes those drivers call their remove callbacks last, just before booting OS and after all the other drivers finished with their remove callbacks. This is necessary for things like clock drivers, where the other drivers might depend on the clock driver in their remove callbacks. Prime example is the mmc subsystem, which can reconfigure a card from HS mode to slower modes in the remove callback and for that it needs to reconfigure the controller clock. Signed-off-by: Marek Vasut <marek.vasut+renesas@gmail.com> Signed-off-by: Simon Glass <sjg@chromium.org>
195 lines
4.1 KiB
C
195 lines
4.1 KiB
C
// SPDX-License-Identifier: GPL-2.0+
|
|
/*
|
|
* Copyright (c) 2013 Google, Inc
|
|
*
|
|
* (C) Copyright 2012
|
|
* Pavel Herrmann <morpheus.ibis@gmail.com>
|
|
*/
|
|
|
|
#include <common.h>
|
|
#include <dm.h>
|
|
#include <errno.h>
|
|
#include <log.h>
|
|
#include <malloc.h>
|
|
#include <asm/io.h>
|
|
#include <dm/device-internal.h>
|
|
#include <dm/test.h>
|
|
#include <test/test.h>
|
|
#include <test/ut.h>
|
|
|
|
int dm_testdrv_op_count[DM_TEST_OP_COUNT];
|
|
static struct unit_test_state *uts = &global_dm_test_state;
|
|
|
|
static int testdrv_ping(struct udevice *dev, int pingval, int *pingret)
|
|
{
|
|
const struct dm_test_pdata *pdata = dev_get_plat(dev);
|
|
struct dm_test_priv *priv = dev_get_priv(dev);
|
|
|
|
*pingret = pingval + pdata->ping_add;
|
|
priv->ping_total += *pingret;
|
|
|
|
return 0;
|
|
}
|
|
|
|
static const struct test_ops test_ops = {
|
|
.ping = testdrv_ping,
|
|
};
|
|
|
|
static int test_bind(struct udevice *dev)
|
|
{
|
|
/* Private data should not be allocated */
|
|
ut_assert(!dev_get_priv(dev));
|
|
|
|
dm_testdrv_op_count[DM_TEST_OP_BIND]++;
|
|
return 0;
|
|
}
|
|
|
|
static int test_probe(struct udevice *dev)
|
|
{
|
|
struct dm_test_priv *priv = dev_get_priv(dev);
|
|
|
|
/* Private data should be allocated */
|
|
ut_assert(priv);
|
|
|
|
dm_testdrv_op_count[DM_TEST_OP_PROBE]++;
|
|
priv->ping_total += DM_TEST_START_TOTAL;
|
|
return 0;
|
|
}
|
|
|
|
static int test_remove(struct udevice *dev)
|
|
{
|
|
/* Private data should still be allocated */
|
|
ut_assert(dev_get_priv(dev));
|
|
|
|
dm_testdrv_op_count[DM_TEST_OP_REMOVE]++;
|
|
return 0;
|
|
}
|
|
|
|
static int test_unbind(struct udevice *dev)
|
|
{
|
|
/* Private data should not be allocated */
|
|
ut_assert(!dev_get_priv(dev));
|
|
|
|
dm_testdrv_op_count[DM_TEST_OP_UNBIND]++;
|
|
return 0;
|
|
}
|
|
|
|
U_BOOT_DRIVER(test_drv) = {
|
|
.name = "test_drv",
|
|
.id = UCLASS_TEST,
|
|
.ops = &test_ops,
|
|
.bind = test_bind,
|
|
.probe = test_probe,
|
|
.remove = test_remove,
|
|
.unbind = test_unbind,
|
|
.priv_auto = sizeof(struct dm_test_priv),
|
|
};
|
|
|
|
U_BOOT_DRIVER(test2_drv) = {
|
|
.name = "test2_drv",
|
|
.id = UCLASS_TEST,
|
|
.ops = &test_ops,
|
|
.bind = test_bind,
|
|
.probe = test_probe,
|
|
.remove = test_remove,
|
|
.unbind = test_unbind,
|
|
.priv_auto = sizeof(struct dm_test_priv),
|
|
};
|
|
|
|
static int test_manual_drv_ping(struct udevice *dev, int pingval, int *pingret)
|
|
{
|
|
*pingret = pingval + 2;
|
|
|
|
return 0;
|
|
}
|
|
|
|
static const struct test_ops test_manual_ops = {
|
|
.ping = test_manual_drv_ping,
|
|
};
|
|
|
|
static int test_manual_bind(struct udevice *dev)
|
|
{
|
|
dm_testdrv_op_count[DM_TEST_OP_BIND]++;
|
|
|
|
return 0;
|
|
}
|
|
|
|
static int test_manual_probe(struct udevice *dev)
|
|
{
|
|
struct dm_test_state *dms = uts->priv;
|
|
|
|
dm_testdrv_op_count[DM_TEST_OP_PROBE]++;
|
|
if (!dms->force_fail_alloc)
|
|
dev_set_priv(dev, calloc(1, sizeof(struct dm_test_priv)));
|
|
if (!dev_get_priv(dev))
|
|
return -ENOMEM;
|
|
|
|
return 0;
|
|
}
|
|
|
|
static int test_manual_remove(struct udevice *dev)
|
|
{
|
|
dm_testdrv_op_count[DM_TEST_OP_REMOVE]++;
|
|
return 0;
|
|
}
|
|
|
|
static int test_manual_unbind(struct udevice *dev)
|
|
{
|
|
dm_testdrv_op_count[DM_TEST_OP_UNBIND]++;
|
|
return 0;
|
|
}
|
|
|
|
U_BOOT_DRIVER(test_manual_drv) = {
|
|
.name = "test_manual_drv",
|
|
.id = UCLASS_TEST,
|
|
.ops = &test_manual_ops,
|
|
.bind = test_manual_bind,
|
|
.probe = test_manual_probe,
|
|
.remove = test_manual_remove,
|
|
.unbind = test_manual_unbind,
|
|
};
|
|
|
|
U_BOOT_DRIVER(test_pre_reloc_drv) = {
|
|
.name = "test_pre_reloc_drv",
|
|
.id = UCLASS_TEST,
|
|
.ops = &test_manual_ops,
|
|
.bind = test_manual_bind,
|
|
.probe = test_manual_probe,
|
|
.remove = test_manual_remove,
|
|
.unbind = test_manual_unbind,
|
|
.flags = DM_FLAG_PRE_RELOC,
|
|
};
|
|
|
|
U_BOOT_DRIVER(test_act_dma_drv) = {
|
|
.name = "test_act_dma_drv",
|
|
.id = UCLASS_TEST,
|
|
.ops = &test_manual_ops,
|
|
.bind = test_manual_bind,
|
|
.probe = test_manual_probe,
|
|
.remove = test_manual_remove,
|
|
.unbind = test_manual_unbind,
|
|
.flags = DM_FLAG_ACTIVE_DMA,
|
|
};
|
|
|
|
U_BOOT_DRIVER(test_vital_clk_drv) = {
|
|
.name = "test_vital_clk_drv",
|
|
.id = UCLASS_TEST,
|
|
.ops = &test_manual_ops,
|
|
.bind = test_manual_bind,
|
|
.probe = test_manual_probe,
|
|
.remove = test_manual_remove,
|
|
.unbind = test_manual_unbind,
|
|
.flags = DM_FLAG_VITAL,
|
|
};
|
|
|
|
U_BOOT_DRIVER(test_act_dma_vital_clk_drv) = {
|
|
.name = "test_act_dma_vital_clk_drv",
|
|
.id = UCLASS_TEST,
|
|
.ops = &test_manual_ops,
|
|
.bind = test_manual_bind,
|
|
.probe = test_manual_probe,
|
|
.remove = test_manual_remove,
|
|
.unbind = test_manual_unbind,
|
|
.flags = DM_FLAG_VITAL | DM_FLAG_ACTIVE_DMA,
|
|
};
|