mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-06-09 23:36:03 +09:00
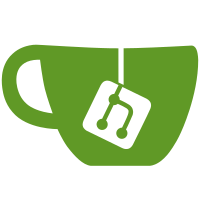
As documented, almost all U-Boot commands expect numbers to be entered in hexadecimal input format. (Exception: for historical reasons, the "sleep" command takes its argument in decimal input format.) This rule was broken for the "load" command; for details please see especially commits045fa1e
"fs: add filesystem switch libary, implement ls and fsload commands" and3f83c87
"fs: fix number base behaviour change in fatload/ext*load". In the result, the load command would always require an explicit "0x" prefix for regular (i. e. base 16 formatted) input. Change this to use the standard notation of base 16 input format. While strictly speaking this is a change of the user interface, we hope that it will not cause trouble. Stephen Warren comments (see [1]): I suppose you can change the behaviour if you want; anyone writing "0x..." for their values presumably won't be affected, and if people really do assume all values in U-Boot are in hex, presumably nobody currently relies upon using non-prefixed values with the generic load command, since it doesn't work like that right now. [1] http://article.gmane.org/gmane.comp.boot-loaders.u-boot/171172 Acked-by: Tom Rini <trini@ti.com> Acked-by: Stephen Warren <swarren@nvidia.com> Signed-off-by: Wolfgang Denk <wd@denx.de>
134 lines
3.2 KiB
C
134 lines
3.2 KiB
C
/*
|
|
* (C) Copyright 2002
|
|
* Richard Jones, rjones@nexus-tech.net
|
|
*
|
|
* SPDX-License-Identifier: GPL-2.0+
|
|
*/
|
|
|
|
/*
|
|
* Boot support
|
|
*/
|
|
#include <common.h>
|
|
#include <command.h>
|
|
#include <s_record.h>
|
|
#include <net.h>
|
|
#include <ata.h>
|
|
#include <part.h>
|
|
#include <fat.h>
|
|
#include <fs.h>
|
|
|
|
int do_fat_fsload (cmd_tbl_t *cmdtp, int flag, int argc, char * const argv[])
|
|
{
|
|
return do_load(cmdtp, flag, argc, argv, FS_TYPE_FAT);
|
|
}
|
|
|
|
|
|
U_BOOT_CMD(
|
|
fatload, 7, 0, do_fat_fsload,
|
|
"load binary file from a dos filesystem",
|
|
"<interface> [<dev[:part]>] <addr> <filename> [bytes [pos]]\n"
|
|
" - Load binary file 'filename' from 'dev' on 'interface'\n"
|
|
" to address 'addr' from dos filesystem.\n"
|
|
" 'pos' gives the file position to start loading from.\n"
|
|
" If 'pos' is omitted, 0 is used. 'pos' requires 'bytes'.\n"
|
|
" 'bytes' gives the size to load. If 'bytes' is 0 or omitted,\n"
|
|
" the load stops on end of file.\n"
|
|
" If either 'pos' or 'bytes' are not aligned to\n"
|
|
" ARCH_DMA_MINALIGN then a misaligned buffer warning will\n"
|
|
" be printed and performance will suffer for the load."
|
|
);
|
|
|
|
static int do_fat_ls(cmd_tbl_t *cmdtp, int flag, int argc, char * const argv[])
|
|
{
|
|
return do_ls(cmdtp, flag, argc, argv, FS_TYPE_FAT);
|
|
}
|
|
|
|
U_BOOT_CMD(
|
|
fatls, 4, 1, do_fat_ls,
|
|
"list files in a directory (default /)",
|
|
"<interface> [<dev[:part]>] [directory]\n"
|
|
" - list files from 'dev' on 'interface' in a 'directory'"
|
|
);
|
|
|
|
static int do_fat_fsinfo(cmd_tbl_t *cmdtp, int flag, int argc,
|
|
char * const argv[])
|
|
{
|
|
int dev, part;
|
|
block_dev_desc_t *dev_desc;
|
|
disk_partition_t info;
|
|
|
|
if (argc < 2) {
|
|
printf("usage: fatinfo <interface> [<dev[:part]>]\n");
|
|
return 0;
|
|
}
|
|
|
|
part = get_device_and_partition(argv[1], argv[2], &dev_desc, &info, 1);
|
|
if (part < 0)
|
|
return 1;
|
|
|
|
dev = dev_desc->dev;
|
|
if (fat_set_blk_dev(dev_desc, &info) != 0) {
|
|
printf("\n** Unable to use %s %d:%d for fatinfo **\n",
|
|
argv[1], dev, part);
|
|
return 1;
|
|
}
|
|
return file_fat_detectfs();
|
|
}
|
|
|
|
U_BOOT_CMD(
|
|
fatinfo, 3, 1, do_fat_fsinfo,
|
|
"print information about filesystem",
|
|
"<interface> [<dev[:part]>]\n"
|
|
" - print information about filesystem from 'dev' on 'interface'"
|
|
);
|
|
|
|
#ifdef CONFIG_FAT_WRITE
|
|
static int do_fat_fswrite(cmd_tbl_t *cmdtp, int flag,
|
|
int argc, char * const argv[])
|
|
{
|
|
long size;
|
|
unsigned long addr;
|
|
unsigned long count;
|
|
block_dev_desc_t *dev_desc = NULL;
|
|
disk_partition_t info;
|
|
int dev = 0;
|
|
int part = 1;
|
|
|
|
if (argc < 5)
|
|
return cmd_usage(cmdtp);
|
|
|
|
part = get_device_and_partition(argv[1], argv[2], &dev_desc, &info, 1);
|
|
if (part < 0)
|
|
return 1;
|
|
|
|
dev = dev_desc->dev;
|
|
|
|
if (fat_set_blk_dev(dev_desc, &info) != 0) {
|
|
printf("\n** Unable to use %s %d:%d for fatwrite **\n",
|
|
argv[1], dev, part);
|
|
return 1;
|
|
}
|
|
addr = simple_strtoul(argv[3], NULL, 16);
|
|
count = simple_strtoul(argv[5], NULL, 16);
|
|
|
|
size = file_fat_write(argv[4], (void *)addr, count);
|
|
if (size == -1) {
|
|
printf("\n** Unable to write \"%s\" from %s %d:%d **\n",
|
|
argv[4], argv[1], dev, part);
|
|
return 1;
|
|
}
|
|
|
|
printf("%ld bytes written\n", size);
|
|
|
|
return 0;
|
|
}
|
|
|
|
U_BOOT_CMD(
|
|
fatwrite, 6, 0, do_fat_fswrite,
|
|
"write file into a dos filesystem",
|
|
"<interface> <dev[:part]> <addr> <filename> <bytes>\n"
|
|
" - write file 'filename' from the address 'addr' in RAM\n"
|
|
" to 'dev' on 'interface'"
|
|
);
|
|
#endif
|