mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-06-09 23:36:03 +09:00
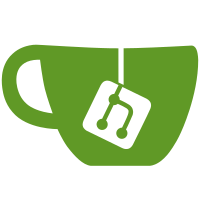
When U-Boot started using SPDX tags we were among the early adopters and there weren't a lot of other examples to borrow from. So we picked the area of the file that usually had a full license text and replaced it with an appropriate SPDX-License-Identifier: entry. Since then, the Linux Kernel has adopted SPDX tags and they place it as the very first line in a file (except where shebangs are used, then it's second line) and with slightly different comment styles than us. In part due to community overlap, in part due to better tag visibility and in part for other minor reasons, switch over to that style. This commit changes all instances where we have a single declared license in the tag as both the before and after are identical in tag contents. There's also a few places where I found we did not have a tag and have introduced one. Signed-off-by: Tom Rini <trini@konsulko.com>
108 lines
3.2 KiB
C
108 lines
3.2 KiB
C
// SPDX-License-Identifier: GPL-2.0+
|
|
/*
|
|
* usb/gadget/config.c -- simplify building config descriptors
|
|
*
|
|
* Copyright (C) 2003 David Brownell
|
|
*
|
|
* Ported to U-Boot by: Thomas Smits <ts.smits@gmail.com> and
|
|
* Remy Bohmer <linux@bohmer.net>
|
|
*/
|
|
|
|
#include <common.h>
|
|
#include <asm/unaligned.h>
|
|
#include <linux/errno.h>
|
|
#include <linux/list.h>
|
|
#include <linux/string.h>
|
|
|
|
#include <linux/usb/ch9.h>
|
|
#include <linux/usb/gadget.h>
|
|
|
|
|
|
/**
|
|
* usb_descriptor_fillbuf - fill buffer with descriptors
|
|
* @buf: Buffer to be filled
|
|
* @buflen: Size of buf
|
|
* @src: Array of descriptor pointers, terminated by null pointer.
|
|
*
|
|
* Copies descriptors into the buffer, returning the length or a
|
|
* negative error code if they can't all be copied. Useful when
|
|
* assembling descriptors for an associated set of interfaces used
|
|
* as part of configuring a composite device; or in other cases where
|
|
* sets of descriptors need to be marshaled.
|
|
*/
|
|
int
|
|
usb_descriptor_fillbuf(void *buf, unsigned buflen,
|
|
const struct usb_descriptor_header **src)
|
|
{
|
|
u8 *dest = buf;
|
|
|
|
if (!src)
|
|
return -EINVAL;
|
|
|
|
/* fill buffer from src[] until null descriptor ptr */
|
|
for (; NULL != *src; src++) {
|
|
unsigned len = (*src)->bLength;
|
|
|
|
if (len > buflen)
|
|
return -EINVAL;
|
|
memcpy(dest, *src, len);
|
|
buflen -= len;
|
|
dest += len;
|
|
}
|
|
return dest - (u8 *)buf;
|
|
}
|
|
|
|
|
|
/**
|
|
* usb_gadget_config_buf - builts a complete configuration descriptor
|
|
* @config: Header for the descriptor, including characteristics such
|
|
* as power requirements and number of interfaces.
|
|
* @desc: Null-terminated vector of pointers to the descriptors (interface,
|
|
* endpoint, etc) defining all functions in this device configuration.
|
|
* @buf: Buffer for the resulting configuration descriptor.
|
|
* @length: Length of buffer. If this is not big enough to hold the
|
|
* entire configuration descriptor, an error code will be returned.
|
|
*
|
|
* This copies descriptors into the response buffer, building a descriptor
|
|
* for that configuration. It returns the buffer length or a negative
|
|
* status code. The config.wTotalLength field is set to match the length
|
|
* of the result, but other descriptor fields (including power usage and
|
|
* interface count) must be set by the caller.
|
|
*
|
|
* Gadget drivers could use this when constructing a config descriptor
|
|
* in response to USB_REQ_GET_DESCRIPTOR. They will need to patch the
|
|
* resulting bDescriptorType value if USB_DT_OTHER_SPEED_CONFIG is needed.
|
|
*/
|
|
int usb_gadget_config_buf(
|
|
const struct usb_config_descriptor *config,
|
|
void *buf,
|
|
unsigned length,
|
|
const struct usb_descriptor_header **desc
|
|
)
|
|
{
|
|
struct usb_config_descriptor *cp = buf;
|
|
int len;
|
|
|
|
/* config descriptor first */
|
|
if (length < USB_DT_CONFIG_SIZE || !desc)
|
|
return -EINVAL;
|
|
/* config need not be aligned */
|
|
memcpy(cp, config, sizeof(*cp));
|
|
|
|
/* then interface/endpoint/class/vendor/... */
|
|
len = usb_descriptor_fillbuf(USB_DT_CONFIG_SIZE + (u8 *)buf,
|
|
length - USB_DT_CONFIG_SIZE, desc);
|
|
if (len < 0)
|
|
return len;
|
|
len += USB_DT_CONFIG_SIZE;
|
|
if (len > 0xffff)
|
|
return -EINVAL;
|
|
|
|
/* patch up the config descriptor */
|
|
cp->bLength = USB_DT_CONFIG_SIZE;
|
|
cp->bDescriptorType = USB_DT_CONFIG;
|
|
put_unaligned_le16(len, &cp->wTotalLength);
|
|
cp->bmAttributes |= USB_CONFIG_ATT_ONE;
|
|
return len;
|
|
}
|