mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-06-09 23:36:03 +09:00
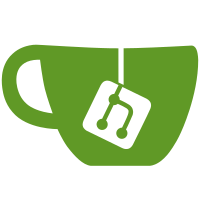
When an operating system started via bootefi tries to reset or power off this is done by calling the EFI runtime ResetSystem(). On most ARMv8 system the actual reset relies on PSCI. Depending on whether the PSCI firmware resides the hypervisor (EL2) or in the secure monitor (EL3) either an HVC or an SMC command has to be issued. The current implementation always uses SMC. This results in crashes on systems where the PSCI firmware is implemented in the hypervisor, e.g. qemu-arm64_defconfig. The logic to decide which call is needed based on the device tree is already implemented in the PSCI firmware driver. During the EFI runtime the device driver model is not available. But we can minimize code duplication by merging the EFI runtime reset and poweroff code with the PSCI firmware driver. As the same HVC/SMC problem is also evident for the ARMv8 do_poweroff and reset_misc routines let's move them into the same code module. Signed-off-by: Heinrich Schuchardt <xypron.glpk@gmx.de> Reviewed-by: Sumit Garg <sumit.garg@linaro.org> Tested-by: Sumit Garg <sumit.garg@linaro.org> Signed-off-by: Alexander Graf <agraf@suse.de>
102 lines
3.4 KiB
C
102 lines
3.4 KiB
C
/*
|
|
* ARM Power State and Coordination Interface (PSCI) header
|
|
*
|
|
* This header holds common PSCI defines and macros shared
|
|
* by: ARM kernel, ARM64 kernel, KVM ARM/ARM64 and user space.
|
|
*
|
|
* Copyright (C) 2014 Linaro Ltd.
|
|
* Author: Anup Patel <anup.patel@linaro.org>
|
|
*/
|
|
|
|
#ifndef _UAPI_LINUX_PSCI_H
|
|
#define _UAPI_LINUX_PSCI_H
|
|
|
|
/*
|
|
* PSCI v0.1 interface
|
|
*
|
|
* The PSCI v0.1 function numbers are implementation defined.
|
|
*
|
|
* Only PSCI return values such as: SUCCESS, NOT_SUPPORTED,
|
|
* INVALID_PARAMS, and DENIED defined below are applicable
|
|
* to PSCI v0.1.
|
|
*/
|
|
|
|
/* PSCI v0.2 interface */
|
|
#define PSCI_0_2_FN_BASE 0x84000000
|
|
#define PSCI_0_2_FN(n) (PSCI_0_2_FN_BASE + (n))
|
|
#define PSCI_0_2_64BIT 0x40000000
|
|
#define PSCI_0_2_FN64_BASE \
|
|
(PSCI_0_2_FN_BASE + PSCI_0_2_64BIT)
|
|
#define PSCI_0_2_FN64(n) (PSCI_0_2_FN64_BASE + (n))
|
|
|
|
#define PSCI_0_2_FN_PSCI_VERSION PSCI_0_2_FN(0)
|
|
#define PSCI_0_2_FN_CPU_SUSPEND PSCI_0_2_FN(1)
|
|
#define PSCI_0_2_FN_CPU_OFF PSCI_0_2_FN(2)
|
|
#define PSCI_0_2_FN_CPU_ON PSCI_0_2_FN(3)
|
|
#define PSCI_0_2_FN_AFFINITY_INFO PSCI_0_2_FN(4)
|
|
#define PSCI_0_2_FN_MIGRATE PSCI_0_2_FN(5)
|
|
#define PSCI_0_2_FN_MIGRATE_INFO_TYPE PSCI_0_2_FN(6)
|
|
#define PSCI_0_2_FN_MIGRATE_INFO_UP_CPU PSCI_0_2_FN(7)
|
|
#define PSCI_0_2_FN_SYSTEM_OFF PSCI_0_2_FN(8)
|
|
#define PSCI_0_2_FN_SYSTEM_RESET PSCI_0_2_FN(9)
|
|
|
|
#define PSCI_0_2_FN64_CPU_SUSPEND PSCI_0_2_FN64(1)
|
|
#define PSCI_0_2_FN64_CPU_ON PSCI_0_2_FN64(3)
|
|
#define PSCI_0_2_FN64_AFFINITY_INFO PSCI_0_2_FN64(4)
|
|
#define PSCI_0_2_FN64_MIGRATE PSCI_0_2_FN64(5)
|
|
#define PSCI_0_2_FN64_MIGRATE_INFO_UP_CPU PSCI_0_2_FN64(7)
|
|
|
|
/* PSCI v0.2 power state encoding for CPU_SUSPEND function */
|
|
#define PSCI_0_2_POWER_STATE_ID_MASK 0xffff
|
|
#define PSCI_0_2_POWER_STATE_ID_SHIFT 0
|
|
#define PSCI_0_2_POWER_STATE_TYPE_SHIFT 16
|
|
#define PSCI_0_2_POWER_STATE_TYPE_MASK \
|
|
(0x1 << PSCI_0_2_POWER_STATE_TYPE_SHIFT)
|
|
#define PSCI_0_2_POWER_STATE_AFFL_SHIFT 24
|
|
#define PSCI_0_2_POWER_STATE_AFFL_MASK \
|
|
(0x3 << PSCI_0_2_POWER_STATE_AFFL_SHIFT)
|
|
|
|
/* PSCI v0.2 affinity level state returned by AFFINITY_INFO */
|
|
#define PSCI_0_2_AFFINITY_LEVEL_ON 0
|
|
#define PSCI_0_2_AFFINITY_LEVEL_OFF 1
|
|
#define PSCI_0_2_AFFINITY_LEVEL_ON_PENDING 2
|
|
|
|
/* PSCI v0.2 multicore support in Trusted OS returned by MIGRATE_INFO_TYPE */
|
|
#define PSCI_0_2_TOS_UP_MIGRATE 0
|
|
#define PSCI_0_2_TOS_UP_NO_MIGRATE 1
|
|
#define PSCI_0_2_TOS_MP 2
|
|
|
|
/* PSCI version decoding (independent of PSCI version) */
|
|
#define PSCI_VERSION_MAJOR_SHIFT 16
|
|
#define PSCI_VERSION_MINOR_MASK \
|
|
((1U << PSCI_VERSION_MAJOR_SHIFT) - 1)
|
|
#define PSCI_VERSION_MAJOR_MASK ~PSCI_VERSION_MINOR_MASK
|
|
#define PSCI_VERSION_MAJOR(ver) \
|
|
(((ver) & PSCI_VERSION_MAJOR_MASK) >> PSCI_VERSION_MAJOR_SHIFT)
|
|
#define PSCI_VERSION_MINOR(ver) \
|
|
((ver) & PSCI_VERSION_MINOR_MASK)
|
|
|
|
/* PSCI return values (inclusive of all PSCI versions) */
|
|
#define PSCI_RET_SUCCESS 0
|
|
#define PSCI_RET_NOT_SUPPORTED -1
|
|
#define PSCI_RET_INVALID_PARAMS -2
|
|
#define PSCI_RET_DENIED -3
|
|
#define PSCI_RET_ALREADY_ON -4
|
|
#define PSCI_RET_ON_PENDING -5
|
|
#define PSCI_RET_INTERNAL_FAILURE -6
|
|
#define PSCI_RET_NOT_PRESENT -7
|
|
#define PSCI_RET_DISABLED -8
|
|
|
|
#ifdef CONFIG_ARM_PSCI_FW
|
|
unsigned long invoke_psci_fn(unsigned long a0, unsigned long a1,
|
|
unsigned long a2, unsigned long a3);
|
|
#else
|
|
unsigned long invoke_psci_fn(unsigned long a0, unsigned long a1,
|
|
unsigned long a2, unsigned long a3)
|
|
{
|
|
return PSCI_RET_DISABLED;
|
|
}
|
|
#endif
|
|
|
|
#endif /* _UAPI_LINUX_PSCI_H */
|