mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-06-09 23:36:03 +09:00
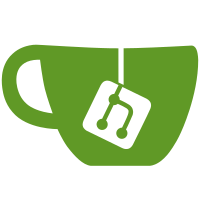
At present each entry has an offset within its parent section. This is useful for figuring out how entries relate to one another. However it is sometimes necessary to locate an entry within an image, regardless of which sections it is nested inside. Add a new 'image-pos' property to provide this information. Also add some documentation for the -u option binman provides, which updates the device tree with final entry information. Since the image position is a better symbol to use for the position of U-Boot as obtained by SPL, update the SPL symbols to use this instead of offset, which might be incorrect if hierarchical sections are used. Signed-off-by: Simon Glass <sjg@chromium.org>
84 lines
2.1 KiB
C
84 lines
2.1 KiB
C
// SPDX-License-Identifier: GPL-2.0+
|
|
/*
|
|
* (C) Copyright 2016
|
|
* Xilinx, Inc.
|
|
*
|
|
* (C) Copyright 2016
|
|
* Toradex AG
|
|
*
|
|
* Michal Simek <michal.simek@xilinx.com>
|
|
* Stefan Agner <stefan.agner@toradex.com>
|
|
*/
|
|
#include <common.h>
|
|
#include <binman_sym.h>
|
|
#include <mapmem.h>
|
|
#include <spl.h>
|
|
#include <linux/libfdt.h>
|
|
|
|
#ifndef CONFIG_SPL_LOAD_FIT_ADDRESS
|
|
# define CONFIG_SPL_LOAD_FIT_ADDRESS 0
|
|
#endif
|
|
|
|
static ulong spl_ram_load_read(struct spl_load_info *load, ulong sector,
|
|
ulong count, void *buf)
|
|
{
|
|
debug("%s: sector %lx, count %lx, buf %lx\n",
|
|
__func__, sector, count, (ulong)buf);
|
|
memcpy(buf, (void *)(CONFIG_SPL_LOAD_FIT_ADDRESS + sector), count);
|
|
return count;
|
|
}
|
|
|
|
static int spl_ram_load_image(struct spl_image_info *spl_image,
|
|
struct spl_boot_device *bootdev)
|
|
{
|
|
struct image_header *header;
|
|
|
|
header = (struct image_header *)CONFIG_SPL_LOAD_FIT_ADDRESS;
|
|
|
|
#if CONFIG_IS_ENABLED(DFU_SUPPORT)
|
|
if (bootdev->boot_device == BOOT_DEVICE_DFU)
|
|
spl_dfu_cmd(0, "dfu_alt_info_ram", "ram", "0");
|
|
#endif
|
|
|
|
if (IS_ENABLED(CONFIG_SPL_LOAD_FIT) &&
|
|
image_get_magic(header) == FDT_MAGIC) {
|
|
struct spl_load_info load;
|
|
|
|
debug("Found FIT\n");
|
|
load.bl_len = 1;
|
|
load.read = spl_ram_load_read;
|
|
spl_load_simple_fit(spl_image, &load, 0, header);
|
|
} else {
|
|
ulong u_boot_pos = binman_sym(ulong, u_boot_any, image_pos);
|
|
|
|
debug("Legacy image\n");
|
|
/*
|
|
* Get the header. It will point to an address defined by
|
|
* handoff which will tell where the image located inside
|
|
* the flash.
|
|
*/
|
|
debug("u_boot_pos = %lx\n", u_boot_pos);
|
|
if (u_boot_pos == BINMAN_SYM_MISSING) {
|
|
/*
|
|
* No binman support or no information. For now, fix it
|
|
* to the address pointed to by U-Boot.
|
|
*/
|
|
u_boot_pos = CONFIG_SYS_TEXT_BASE -
|
|
sizeof(struct image_header);
|
|
}
|
|
header = (struct image_header *)map_sysmem(u_boot_pos, 0);
|
|
|
|
spl_parse_image_header(spl_image, header);
|
|
}
|
|
|
|
return 0;
|
|
}
|
|
#if CONFIG_IS_ENABLED(RAM_DEVICE)
|
|
SPL_LOAD_IMAGE_METHOD("RAM", 0, BOOT_DEVICE_RAM, spl_ram_load_image);
|
|
#endif
|
|
#if CONFIG_IS_ENABLED(DFU_SUPPORT)
|
|
SPL_LOAD_IMAGE_METHOD("DFU", 0, BOOT_DEVICE_DFU, spl_ram_load_image);
|
|
#endif
|
|
|
|
|