mirror of
https://github.com/brain-hackers/linux-brain.git
synced 2024-06-08 23:14:51 +09:00
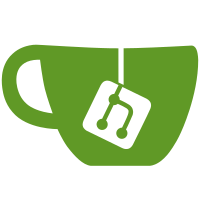
When calling debugfs functions, there is no need to ever check the return value. The function can work or not, but the code logic should never do something different based on this. Also, because there is no need to save the file dentry, remove all of the variables that were being saved, and just recursively delete the whole directory when shutting down, saving a lot of logic and local variables. [gregkh@linuxfoundation.org: v2] Link: http://lkml.kernel.org/r/20190613055455.GE19717@kroah.com Link: http://lkml.kernel.org/r/20190612152912.GA19151@kroah.com Signed-off-by: Greg Kroah-Hartman <gregkh@linuxfoundation.org> Reviewed-by: Joseph Qi <joseph.qi@linux.alibaba.com> Cc: Mark Fasheh <mark@fasheh.com> Cc: Joel Becker <jlbec@evilplan.org> Cc: Joseph Qi <joseph.qi@linux.alibaba.com> Cc: Jia Guo <guojia12@huawei.com> Cc: Junxiao Bi <junxiao.bi@oracle.com> Cc: Changwei Ge <gechangwei@live.cn> Cc: Gang He <ghe@suse.com> Cc: Jun Piao <piaojun@huawei.com> Signed-off-by: Andrew Morton <akpm@linux-foundation.org> Signed-off-by: Linus Torvalds <torvalds@linux-foundation.org>
74 lines
2.0 KiB
C
74 lines
2.0 KiB
C
/* SPDX-License-Identifier: GPL-2.0-or-later */
|
|
/* -*- mode: c; c-basic-offset: 8; -*-
|
|
* vim: noexpandtab sw=8 ts=8 sts=0:
|
|
*
|
|
* heartbeat.h
|
|
*
|
|
* Function prototypes
|
|
*
|
|
* Copyright (C) 2004 Oracle. All rights reserved.
|
|
*/
|
|
|
|
#ifndef O2CLUSTER_HEARTBEAT_H
|
|
#define O2CLUSTER_HEARTBEAT_H
|
|
|
|
#include "ocfs2_heartbeat.h"
|
|
|
|
#define O2HB_REGION_TIMEOUT_MS 2000
|
|
|
|
#define O2HB_MAX_REGION_NAME_LEN 32
|
|
|
|
/* number of changes to be seen as live */
|
|
#define O2HB_LIVE_THRESHOLD 2
|
|
/* number of equal samples to be seen as dead */
|
|
extern unsigned int o2hb_dead_threshold;
|
|
#define O2HB_DEFAULT_DEAD_THRESHOLD 31
|
|
/* Otherwise MAX_WRITE_TIMEOUT will be zero... */
|
|
#define O2HB_MIN_DEAD_THRESHOLD 2
|
|
#define O2HB_MAX_WRITE_TIMEOUT_MS (O2HB_REGION_TIMEOUT_MS * (o2hb_dead_threshold - 1))
|
|
|
|
#define O2HB_CB_MAGIC 0x51d1e4ec
|
|
|
|
/* callback stuff */
|
|
enum o2hb_callback_type {
|
|
O2HB_NODE_DOWN_CB = 0,
|
|
O2HB_NODE_UP_CB,
|
|
O2HB_NUM_CB
|
|
};
|
|
|
|
struct o2nm_node;
|
|
typedef void (o2hb_cb_func)(struct o2nm_node *, int, void *);
|
|
|
|
struct o2hb_callback_func {
|
|
u32 hc_magic;
|
|
struct list_head hc_item;
|
|
o2hb_cb_func *hc_func;
|
|
void *hc_data;
|
|
int hc_priority;
|
|
enum o2hb_callback_type hc_type;
|
|
};
|
|
|
|
struct config_group *o2hb_alloc_hb_set(void);
|
|
void o2hb_free_hb_set(struct config_group *group);
|
|
|
|
void o2hb_setup_callback(struct o2hb_callback_func *hc,
|
|
enum o2hb_callback_type type,
|
|
o2hb_cb_func *func,
|
|
void *data,
|
|
int priority);
|
|
int o2hb_register_callback(const char *region_uuid,
|
|
struct o2hb_callback_func *hc);
|
|
void o2hb_unregister_callback(const char *region_uuid,
|
|
struct o2hb_callback_func *hc);
|
|
void o2hb_fill_node_map(unsigned long *map,
|
|
unsigned bytes);
|
|
void o2hb_exit(void);
|
|
void o2hb_init(void);
|
|
int o2hb_check_node_heartbeating_no_sem(u8 node_num);
|
|
int o2hb_check_node_heartbeating_from_callback(u8 node_num);
|
|
void o2hb_stop_all_regions(void);
|
|
int o2hb_get_all_regions(char *region_uuids, u8 numregions);
|
|
int o2hb_global_heartbeat_active(void);
|
|
|
|
#endif /* O2CLUSTER_HEARTBEAT_H */
|