mirror of
https://github.com/brain-hackers/linux-brain.git
synced 2024-06-09 15:26:21 +09:00
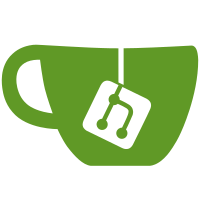
commit 4fdec2034b
upstream.
Processor tracing is already enumerated in word 9 (CPUID[7,0].EBX),
so do not duplicate it in the scattered features word.
Besides being more tidy, this will be useful for KVM when it presents
processor tracing to the guests. KVM selects host features that are
supported by both the host kernel (depending on command line options,
CPU errata, or whatever) and KVM. Whenever a full feature word exists,
KVM's code is written in the expectation that the CPUID bit number
matches the X86_FEATURE_* bit number, but this is not the case for
X86_FEATURE_INTEL_PT.
Signed-off-by: Paolo Bonzini <pbonzini@redhat.com>
Cc: Borislav Petkov <bp@suse.de>
Cc: Linus Torvalds <torvalds@linux-foundation.org>
Cc: Luwei Kang <luwei.kang@intel.com>
Cc: Peter Zijlstra <peterz@infradead.org>
Cc: Radim Krčmář <rkrcmar@redhat.com>
Cc: Thomas Gleixner <tglx@linutronix.de>
Cc: kvm@vger.kernel.org
Link: http://lkml.kernel.org/r/1516117345-34561-1-git-send-email-pbonzini@redhat.com
Signed-off-by: Ingo Molnar <mingo@kernel.org>
Signed-off-by: Greg Kroah-Hartman <gregkh@linuxfoundation.org>
83 lines
2.1 KiB
C
83 lines
2.1 KiB
C
/*
|
|
* Routines to identify additional cpu features that are scattered in
|
|
* cpuid space.
|
|
*/
|
|
#include <linux/cpu.h>
|
|
|
|
#include <asm/pat.h>
|
|
#include <asm/processor.h>
|
|
|
|
#include <asm/apic.h>
|
|
|
|
struct cpuid_bit {
|
|
u16 feature;
|
|
u8 reg;
|
|
u8 bit;
|
|
u32 level;
|
|
u32 sub_leaf;
|
|
};
|
|
|
|
/* Please keep the leaf sorted by cpuid_bit.level for faster search. */
|
|
static const struct cpuid_bit cpuid_bits[] = {
|
|
{ X86_FEATURE_APERFMPERF, CPUID_ECX, 0, 0x00000006, 0 },
|
|
{ X86_FEATURE_EPB, CPUID_ECX, 3, 0x00000006, 0 },
|
|
{ X86_FEATURE_AVX512_4VNNIW, CPUID_EDX, 2, 0x00000007, 0 },
|
|
{ X86_FEATURE_AVX512_4FMAPS, CPUID_EDX, 3, 0x00000007, 0 },
|
|
{ X86_FEATURE_CAT_L3, CPUID_EBX, 1, 0x00000010, 0 },
|
|
{ X86_FEATURE_CAT_L2, CPUID_EBX, 2, 0x00000010, 0 },
|
|
{ X86_FEATURE_CDP_L3, CPUID_ECX, 2, 0x00000010, 1 },
|
|
{ X86_FEATURE_MBA, CPUID_EBX, 3, 0x00000010, 0 },
|
|
{ X86_FEATURE_HW_PSTATE, CPUID_EDX, 7, 0x80000007, 0 },
|
|
{ X86_FEATURE_CPB, CPUID_EDX, 9, 0x80000007, 0 },
|
|
{ X86_FEATURE_PROC_FEEDBACK, CPUID_EDX, 11, 0x80000007, 0 },
|
|
{ X86_FEATURE_SME, CPUID_EAX, 0, 0x8000001f, 0 },
|
|
{ 0, 0, 0, 0, 0 }
|
|
};
|
|
|
|
void init_scattered_cpuid_features(struct cpuinfo_x86 *c)
|
|
{
|
|
u32 max_level;
|
|
u32 regs[4];
|
|
const struct cpuid_bit *cb;
|
|
|
|
for (cb = cpuid_bits; cb->feature; cb++) {
|
|
|
|
/* Verify that the level is valid */
|
|
max_level = cpuid_eax(cb->level & 0xffff0000);
|
|
if (max_level < cb->level ||
|
|
max_level > (cb->level | 0xffff))
|
|
continue;
|
|
|
|
cpuid_count(cb->level, cb->sub_leaf, ®s[CPUID_EAX],
|
|
®s[CPUID_EBX], ®s[CPUID_ECX],
|
|
®s[CPUID_EDX]);
|
|
|
|
if (regs[cb->reg] & (1 << cb->bit))
|
|
set_cpu_cap(c, cb->feature);
|
|
}
|
|
}
|
|
|
|
u32 get_scattered_cpuid_leaf(unsigned int level, unsigned int sub_leaf,
|
|
enum cpuid_regs_idx reg)
|
|
{
|
|
const struct cpuid_bit *cb;
|
|
u32 cpuid_val = 0;
|
|
|
|
for (cb = cpuid_bits; cb->feature; cb++) {
|
|
|
|
if (level > cb->level)
|
|
continue;
|
|
|
|
if (level < cb->level)
|
|
break;
|
|
|
|
if (reg == cb->reg && sub_leaf == cb->sub_leaf) {
|
|
if (cpu_has(&boot_cpu_data, cb->feature))
|
|
cpuid_val |= BIT(cb->bit);
|
|
}
|
|
}
|
|
|
|
return cpuid_val;
|
|
}
|
|
EXPORT_SYMBOL_GPL(get_scattered_cpuid_leaf);
|