mirror of
https://github.com/brain-hackers/linux-brain.git
synced 2024-06-09 23:36:23 +09:00
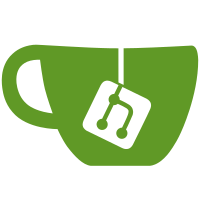
Based on 1 normalized pattern(s): this program is free software you can redistribute it and or modify it under the terms of the gnu general public license version 2 as published by the free software foundation this program is distributed in the hope that it will be useful but without any warranty without even the implied warranty of merchantability or fitness for a particular purpose see the gnu general public license for more details extracted by the scancode license scanner the SPDX license identifier GPL-2.0-only has been chosen to replace the boilerplate/reference in 655 file(s). Signed-off-by: Thomas Gleixner <tglx@linutronix.de> Reviewed-by: Allison Randal <allison@lohutok.net> Reviewed-by: Kate Stewart <kstewart@linuxfoundation.org> Reviewed-by: Richard Fontana <rfontana@redhat.com> Cc: linux-spdx@vger.kernel.org Link: https://lkml.kernel.org/r/20190527070034.575739538@linutronix.de Signed-off-by: Greg Kroah-Hartman <gregkh@linuxfoundation.org>
74 lines
2.0 KiB
C
74 lines
2.0 KiB
C
/* SPDX-License-Identifier: GPL-2.0-only */
|
|
/*
|
|
* fence-chain: chain fences together in a timeline
|
|
*
|
|
* Copyright (C) 2018 Advanced Micro Devices, Inc.
|
|
* Authors:
|
|
* Christian König <christian.koenig@amd.com>
|
|
*/
|
|
|
|
#ifndef __LINUX_DMA_FENCE_CHAIN_H
|
|
#define __LINUX_DMA_FENCE_CHAIN_H
|
|
|
|
#include <linux/dma-fence.h>
|
|
#include <linux/irq_work.h>
|
|
|
|
/**
|
|
* struct dma_fence_chain - fence to represent an node of a fence chain
|
|
* @base: fence base class
|
|
* @lock: spinlock for fence handling
|
|
* @prev: previous fence of the chain
|
|
* @prev_seqno: original previous seqno before garbage collection
|
|
* @fence: encapsulated fence
|
|
* @cb: callback structure for signaling
|
|
* @work: irq work item for signaling
|
|
*/
|
|
struct dma_fence_chain {
|
|
struct dma_fence base;
|
|
spinlock_t lock;
|
|
struct dma_fence __rcu *prev;
|
|
u64 prev_seqno;
|
|
struct dma_fence *fence;
|
|
struct dma_fence_cb cb;
|
|
struct irq_work work;
|
|
};
|
|
|
|
extern const struct dma_fence_ops dma_fence_chain_ops;
|
|
|
|
/**
|
|
* to_dma_fence_chain - cast a fence to a dma_fence_chain
|
|
* @fence: fence to cast to a dma_fence_array
|
|
*
|
|
* Returns NULL if the fence is not a dma_fence_chain,
|
|
* or the dma_fence_chain otherwise.
|
|
*/
|
|
static inline struct dma_fence_chain *
|
|
to_dma_fence_chain(struct dma_fence *fence)
|
|
{
|
|
if (!fence || fence->ops != &dma_fence_chain_ops)
|
|
return NULL;
|
|
|
|
return container_of(fence, struct dma_fence_chain, base);
|
|
}
|
|
|
|
/**
|
|
* dma_fence_chain_for_each - iterate over all fences in chain
|
|
* @iter: current fence
|
|
* @head: starting point
|
|
*
|
|
* Iterate over all fences in the chain. We keep a reference to the current
|
|
* fence while inside the loop which must be dropped when breaking out.
|
|
*/
|
|
#define dma_fence_chain_for_each(iter, head) \
|
|
for (iter = dma_fence_get(head); iter; \
|
|
iter = dma_fence_chain_walk(iter))
|
|
|
|
struct dma_fence *dma_fence_chain_walk(struct dma_fence *fence);
|
|
int dma_fence_chain_find_seqno(struct dma_fence **pfence, uint64_t seqno);
|
|
void dma_fence_chain_init(struct dma_fence_chain *chain,
|
|
struct dma_fence *prev,
|
|
struct dma_fence *fence,
|
|
uint64_t seqno);
|
|
|
|
#endif /* __LINUX_DMA_FENCE_CHAIN_H */
|