mirror of
https://github.com/brain-hackers/linux-brain.git
synced 2024-06-09 15:26:21 +09:00
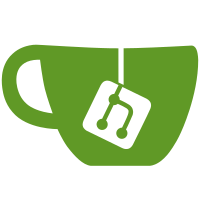
We reserve 128 bytes for struct siginfo but only use about 48 bytes on 64bit and 32 bytes on 32bit. Someday we might use more but it is unlikely to be anytime soon. Userspace seems content with just enough bytes of siginfo to implement sigqueue. Or in the case of checkpoint/restart reinjecting signals the kernel has sent. Reducing the stack footprint and the work to copy siginfo around from 2 cachelines to 1 cachelines seems worth doing even if I don't have benchmarks to show a performance difference. Suggested-by: Linus Torvalds <torvalds@linux-foundation.org> Signed-off-by: "Eric W. Biederman" <ebiederm@xmission.com>
72 lines
1.2 KiB
C
72 lines
1.2 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
#ifndef _LINUX_SIGNAL_TYPES_H
|
|
#define _LINUX_SIGNAL_TYPES_H
|
|
|
|
/*
|
|
* Basic signal handling related data type definitions:
|
|
*/
|
|
|
|
#include <linux/list.h>
|
|
#include <uapi/linux/signal.h>
|
|
|
|
typedef struct kernel_siginfo {
|
|
__SIGINFO;
|
|
} kernel_siginfo_t;
|
|
|
|
/*
|
|
* Real Time signals may be queued.
|
|
*/
|
|
|
|
struct sigqueue {
|
|
struct list_head list;
|
|
int flags;
|
|
kernel_siginfo_t info;
|
|
struct user_struct *user;
|
|
};
|
|
|
|
/* flags values. */
|
|
#define SIGQUEUE_PREALLOC 1
|
|
|
|
struct sigpending {
|
|
struct list_head list;
|
|
sigset_t signal;
|
|
};
|
|
|
|
struct sigaction {
|
|
#ifndef __ARCH_HAS_IRIX_SIGACTION
|
|
__sighandler_t sa_handler;
|
|
unsigned long sa_flags;
|
|
#else
|
|
unsigned int sa_flags;
|
|
__sighandler_t sa_handler;
|
|
#endif
|
|
#ifdef __ARCH_HAS_SA_RESTORER
|
|
__sigrestore_t sa_restorer;
|
|
#endif
|
|
sigset_t sa_mask; /* mask last for extensibility */
|
|
};
|
|
|
|
struct k_sigaction {
|
|
struct sigaction sa;
|
|
#ifdef __ARCH_HAS_KA_RESTORER
|
|
__sigrestore_t ka_restorer;
|
|
#endif
|
|
};
|
|
|
|
#ifdef CONFIG_OLD_SIGACTION
|
|
struct old_sigaction {
|
|
__sighandler_t sa_handler;
|
|
old_sigset_t sa_mask;
|
|
unsigned long sa_flags;
|
|
__sigrestore_t sa_restorer;
|
|
};
|
|
#endif
|
|
|
|
struct ksignal {
|
|
struct k_sigaction ka;
|
|
kernel_siginfo_t info;
|
|
int sig;
|
|
};
|
|
|
|
#endif /* _LINUX_SIGNAL_TYPES_H */
|