mirror of
https://github.com/brain-hackers/linux-brain.git
synced 2024-06-09 23:36:23 +09:00
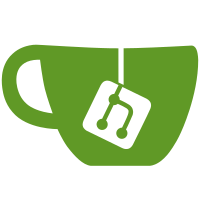
rxkad sometimes triggers a warning about oversized stack frames when
building with clang for a 32-bit architecture:
net/rxrpc/rxkad.c:243:12: error: stack frame size of 1088 bytes in function 'rxkad_secure_packet' [-Werror,-Wframe-larger-than=]
net/rxrpc/rxkad.c:501:12: error: stack frame size of 1088 bytes in function 'rxkad_verify_packet' [-Werror,-Wframe-larger-than=]
The problem is the combination of SYNC_SKCIPHER_REQUEST_ON_STACK() in
rxkad_verify_packet()/rxkad_secure_packet() with the relatively large
scatterlist in rxkad_verify_packet_1()/rxkad_secure_packet_encrypt().
The warning does not show up when using gcc, which does not inline the
functions as aggressively, but the problem is still the same.
Allocate the cipher buffers from the slab instead, caching the allocated
packet crypto request memory used for DATA packet crypto in the rxrpc_call
struct.
Fixes: 17926a7932
("[AF_RXRPC]: Provide secure RxRPC sockets for use by userspace and kernel both")
Reported-by: Arnd Bergmann <arnd@arndb.de>
Signed-off-by: David Howells <dhowells@redhat.com>
Acked-by: Arnd Bergmann <arnd@arndb.de>
cc: Herbert Xu <herbert@gondor.apana.org.au>
Signed-off-by: David S. Miller <davem@davemloft.net>
98 lines
2.2 KiB
C
98 lines
2.2 KiB
C
// SPDX-License-Identifier: GPL-2.0-or-later
|
|
/* Null security operations.
|
|
*
|
|
* Copyright (C) 2016 Red Hat, Inc. All Rights Reserved.
|
|
* Written by David Howells (dhowells@redhat.com)
|
|
*/
|
|
|
|
#include <net/af_rxrpc.h>
|
|
#include "ar-internal.h"
|
|
|
|
static int none_init_connection_security(struct rxrpc_connection *conn)
|
|
{
|
|
return 0;
|
|
}
|
|
|
|
static int none_prime_packet_security(struct rxrpc_connection *conn)
|
|
{
|
|
return 0;
|
|
}
|
|
|
|
static int none_secure_packet(struct rxrpc_call *call,
|
|
struct sk_buff *skb,
|
|
size_t data_size,
|
|
void *sechdr)
|
|
{
|
|
return 0;
|
|
}
|
|
|
|
static int none_verify_packet(struct rxrpc_call *call, struct sk_buff *skb,
|
|
unsigned int offset, unsigned int len,
|
|
rxrpc_seq_t seq, u16 expected_cksum)
|
|
{
|
|
return 0;
|
|
}
|
|
|
|
static void none_free_call_crypto(struct rxrpc_call *call)
|
|
{
|
|
}
|
|
|
|
static void none_locate_data(struct rxrpc_call *call, struct sk_buff *skb,
|
|
unsigned int *_offset, unsigned int *_len)
|
|
{
|
|
}
|
|
|
|
static int none_respond_to_challenge(struct rxrpc_connection *conn,
|
|
struct sk_buff *skb,
|
|
u32 *_abort_code)
|
|
{
|
|
struct rxrpc_skb_priv *sp = rxrpc_skb(skb);
|
|
|
|
trace_rxrpc_rx_eproto(NULL, sp->hdr.serial,
|
|
tracepoint_string("chall_none"));
|
|
return -EPROTO;
|
|
}
|
|
|
|
static int none_verify_response(struct rxrpc_connection *conn,
|
|
struct sk_buff *skb,
|
|
u32 *_abort_code)
|
|
{
|
|
struct rxrpc_skb_priv *sp = rxrpc_skb(skb);
|
|
|
|
trace_rxrpc_rx_eproto(NULL, sp->hdr.serial,
|
|
tracepoint_string("resp_none"));
|
|
return -EPROTO;
|
|
}
|
|
|
|
static void none_clear(struct rxrpc_connection *conn)
|
|
{
|
|
}
|
|
|
|
static int none_init(void)
|
|
{
|
|
return 0;
|
|
}
|
|
|
|
static void none_exit(void)
|
|
{
|
|
}
|
|
|
|
/*
|
|
* RxRPC Kerberos-based security
|
|
*/
|
|
const struct rxrpc_security rxrpc_no_security = {
|
|
.name = "none",
|
|
.security_index = RXRPC_SECURITY_NONE,
|
|
.init = none_init,
|
|
.exit = none_exit,
|
|
.init_connection_security = none_init_connection_security,
|
|
.prime_packet_security = none_prime_packet_security,
|
|
.free_call_crypto = none_free_call_crypto,
|
|
.secure_packet = none_secure_packet,
|
|
.verify_packet = none_verify_packet,
|
|
.locate_data = none_locate_data,
|
|
.respond_to_challenge = none_respond_to_challenge,
|
|
.verify_response = none_verify_response,
|
|
.clear = none_clear,
|
|
};
|