mirror of
https://github.com/brain-hackers/linux-brain.git
synced 2024-06-09 07:16:21 +09:00
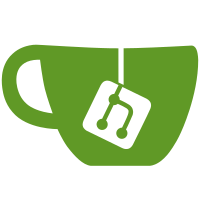
This adds support for partial file caching in Coda. Every read, write and mmap informs the userspace cache manager about what part of a file is about to be accessed so that the cache manager can ensure the relevant parts are available before the operation is allowed to proceed. When a read or write operation completes, this is also reported to allow the cache manager to track when partially cached content can be released. If the cache manager does not support partial file caching, or when the entire file has been fetched into the local cache, the cache manager may return an EOPNOTSUPP error to indicate that intent upcalls are no longer necessary until the file is closed. [akpm@linux-foundation.org: little whitespace fixup] Link: http://lkml.kernel.org/r/20190618181301.6960-1-jaharkes@cs.cmu.edu Signed-off-by: Pedro Cuadra <pjcuadra@gmail.com> Signed-off-by: Jan Harkes <jaharkes@cs.cmu.edu> Signed-off-by: Andrew Morton <akpm@linux-foundation.org> Signed-off-by: Linus Torvalds <torvalds@linux-foundation.org>
60 lines
1.9 KiB
C
60 lines
1.9 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
/*
|
|
* coda_fs_i.h
|
|
*
|
|
* Copyright (C) 1998 Carnegie Mellon University
|
|
*
|
|
*/
|
|
|
|
#ifndef _LINUX_CODA_FS_I
|
|
#define _LINUX_CODA_FS_I
|
|
|
|
#include <linux/types.h>
|
|
#include <linux/list.h>
|
|
#include <linux/spinlock.h>
|
|
#include <linux/coda.h>
|
|
|
|
/*
|
|
* coda fs inode data
|
|
* c_lock protects accesses to c_flags, c_mapcount, c_cached_epoch, c_uid and
|
|
* c_cached_perm.
|
|
* vfs_inode is set only when the inode is created and never changes.
|
|
* c_fid is set when the inode is created and should be considered immutable.
|
|
*/
|
|
struct coda_inode_info {
|
|
struct CodaFid c_fid; /* Coda identifier */
|
|
u_short c_flags; /* flags (see below) */
|
|
unsigned int c_mapcount; /* nr of times this inode is mapped */
|
|
unsigned int c_cached_epoch; /* epoch for cached permissions */
|
|
kuid_t c_uid; /* fsuid for cached permissions */
|
|
unsigned int c_cached_perm; /* cached access permissions */
|
|
spinlock_t c_lock;
|
|
struct inode vfs_inode;
|
|
};
|
|
|
|
/*
|
|
* coda fs file private data
|
|
*/
|
|
#define CODA_MAGIC 0xC0DAC0DA
|
|
struct coda_file_info {
|
|
int cfi_magic; /* magic number */
|
|
struct file *cfi_container; /* container file for this cnode */
|
|
unsigned int cfi_mapcount; /* nr of times this file is mapped */
|
|
bool cfi_access_intent; /* is access intent supported */
|
|
};
|
|
|
|
/* flags */
|
|
#define C_VATTR 0x1 /* Validity of vattr in inode */
|
|
#define C_FLUSH 0x2 /* used after a flush */
|
|
#define C_DYING 0x4 /* from venus (which died) */
|
|
#define C_PURGE 0x8
|
|
|
|
struct inode *coda_cnode_make(struct CodaFid *, struct super_block *);
|
|
struct inode *coda_iget(struct super_block *sb, struct CodaFid *fid, struct coda_vattr *attr);
|
|
struct inode *coda_cnode_makectl(struct super_block *sb);
|
|
struct inode *coda_fid_to_inode(struct CodaFid *fid, struct super_block *sb);
|
|
struct coda_file_info *coda_ftoc(struct file *file);
|
|
void coda_replace_fid(struct inode *, struct CodaFid *, struct CodaFid *);
|
|
|
|
#endif
|