mirror of
https://github.com/brain-hackers/linux-brain.git
synced 2024-06-09 23:36:23 +09:00
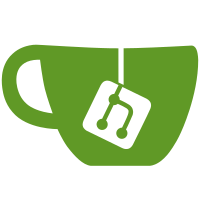
The following kernel panic was observed on ARM64 platform due to a stale TLB entry. 1. ioremap with 4K size, a valid pte page table is set. 2. iounmap it, its pte entry is set to 0. 3. ioremap the same address with 2M size, update its pmd entry with a new value. 4. CPU may hit an exception because the old pmd entry is still in TLB, which leads to a kernel panic. Commitb6bdb7517c
("mm/vmalloc: add interfaces to free unmapped page table") has addressed this panic by falling to pte mappings in the above case on ARM64. To support pmd mappings in all cases, TLB purge needs to be performed in this case on ARM64. Add a new arg, 'addr', to pud_free_pmd_page() and pmd_free_pte_page() so that TLB purge can be added later in seprate patches. [toshi.kani@hpe.com: merge changes, rewrite patch description] Fixes:28ee90fe60
("x86/mm: implement free pmd/pte page interfaces") Signed-off-by: Chintan Pandya <cpandya@codeaurora.org> Signed-off-by: Toshi Kani <toshi.kani@hpe.com> Signed-off-by: Thomas Gleixner <tglx@linutronix.de> Cc: mhocko@suse.com Cc: akpm@linux-foundation.org Cc: hpa@zytor.com Cc: linux-mm@kvack.org Cc: linux-arm-kernel@lists.infradead.org Cc: Will Deacon <will.deacon@arm.com> Cc: Joerg Roedel <joro@8bytes.org> Cc: stable@vger.kernel.org Cc: Andrew Morton <akpm@linux-foundation.org> Cc: Michal Hocko <mhocko@suse.com> Cc: "H. Peter Anvin" <hpa@zytor.com> Cc: <stable@vger.kernel.org> Link: https://lkml.kernel.org/r/20180627141348.21777-3-toshi.kani@hpe.com
184 lines
4.2 KiB
C
184 lines
4.2 KiB
C
// SPDX-License-Identifier: GPL-2.0
|
|
/*
|
|
* Re-map IO memory to kernel address space so that we can access it.
|
|
* This is needed for high PCI addresses that aren't mapped in the
|
|
* 640k-1MB IO memory area on PC's
|
|
*
|
|
* (C) Copyright 1995 1996 Linus Torvalds
|
|
*/
|
|
#include <linux/vmalloc.h>
|
|
#include <linux/mm.h>
|
|
#include <linux/sched.h>
|
|
#include <linux/io.h>
|
|
#include <linux/export.h>
|
|
#include <asm/cacheflush.h>
|
|
#include <asm/pgtable.h>
|
|
|
|
#ifdef CONFIG_HAVE_ARCH_HUGE_VMAP
|
|
static int __read_mostly ioremap_p4d_capable;
|
|
static int __read_mostly ioremap_pud_capable;
|
|
static int __read_mostly ioremap_pmd_capable;
|
|
static int __read_mostly ioremap_huge_disabled;
|
|
|
|
static int __init set_nohugeiomap(char *str)
|
|
{
|
|
ioremap_huge_disabled = 1;
|
|
return 0;
|
|
}
|
|
early_param("nohugeiomap", set_nohugeiomap);
|
|
|
|
void __init ioremap_huge_init(void)
|
|
{
|
|
if (!ioremap_huge_disabled) {
|
|
if (arch_ioremap_pud_supported())
|
|
ioremap_pud_capable = 1;
|
|
if (arch_ioremap_pmd_supported())
|
|
ioremap_pmd_capable = 1;
|
|
}
|
|
}
|
|
|
|
static inline int ioremap_p4d_enabled(void)
|
|
{
|
|
return ioremap_p4d_capable;
|
|
}
|
|
|
|
static inline int ioremap_pud_enabled(void)
|
|
{
|
|
return ioremap_pud_capable;
|
|
}
|
|
|
|
static inline int ioremap_pmd_enabled(void)
|
|
{
|
|
return ioremap_pmd_capable;
|
|
}
|
|
|
|
#else /* !CONFIG_HAVE_ARCH_HUGE_VMAP */
|
|
static inline int ioremap_p4d_enabled(void) { return 0; }
|
|
static inline int ioremap_pud_enabled(void) { return 0; }
|
|
static inline int ioremap_pmd_enabled(void) { return 0; }
|
|
#endif /* CONFIG_HAVE_ARCH_HUGE_VMAP */
|
|
|
|
static int ioremap_pte_range(pmd_t *pmd, unsigned long addr,
|
|
unsigned long end, phys_addr_t phys_addr, pgprot_t prot)
|
|
{
|
|
pte_t *pte;
|
|
u64 pfn;
|
|
|
|
pfn = phys_addr >> PAGE_SHIFT;
|
|
pte = pte_alloc_kernel(pmd, addr);
|
|
if (!pte)
|
|
return -ENOMEM;
|
|
do {
|
|
BUG_ON(!pte_none(*pte));
|
|
set_pte_at(&init_mm, addr, pte, pfn_pte(pfn, prot));
|
|
pfn++;
|
|
} while (pte++, addr += PAGE_SIZE, addr != end);
|
|
return 0;
|
|
}
|
|
|
|
static inline int ioremap_pmd_range(pud_t *pud, unsigned long addr,
|
|
unsigned long end, phys_addr_t phys_addr, pgprot_t prot)
|
|
{
|
|
pmd_t *pmd;
|
|
unsigned long next;
|
|
|
|
phys_addr -= addr;
|
|
pmd = pmd_alloc(&init_mm, pud, addr);
|
|
if (!pmd)
|
|
return -ENOMEM;
|
|
do {
|
|
next = pmd_addr_end(addr, end);
|
|
|
|
if (ioremap_pmd_enabled() &&
|
|
((next - addr) == PMD_SIZE) &&
|
|
IS_ALIGNED(phys_addr + addr, PMD_SIZE) &&
|
|
pmd_free_pte_page(pmd, addr)) {
|
|
if (pmd_set_huge(pmd, phys_addr + addr, prot))
|
|
continue;
|
|
}
|
|
|
|
if (ioremap_pte_range(pmd, addr, next, phys_addr + addr, prot))
|
|
return -ENOMEM;
|
|
} while (pmd++, addr = next, addr != end);
|
|
return 0;
|
|
}
|
|
|
|
static inline int ioremap_pud_range(p4d_t *p4d, unsigned long addr,
|
|
unsigned long end, phys_addr_t phys_addr, pgprot_t prot)
|
|
{
|
|
pud_t *pud;
|
|
unsigned long next;
|
|
|
|
phys_addr -= addr;
|
|
pud = pud_alloc(&init_mm, p4d, addr);
|
|
if (!pud)
|
|
return -ENOMEM;
|
|
do {
|
|
next = pud_addr_end(addr, end);
|
|
|
|
if (ioremap_pud_enabled() &&
|
|
((next - addr) == PUD_SIZE) &&
|
|
IS_ALIGNED(phys_addr + addr, PUD_SIZE) &&
|
|
pud_free_pmd_page(pud, addr)) {
|
|
if (pud_set_huge(pud, phys_addr + addr, prot))
|
|
continue;
|
|
}
|
|
|
|
if (ioremap_pmd_range(pud, addr, next, phys_addr + addr, prot))
|
|
return -ENOMEM;
|
|
} while (pud++, addr = next, addr != end);
|
|
return 0;
|
|
}
|
|
|
|
static inline int ioremap_p4d_range(pgd_t *pgd, unsigned long addr,
|
|
unsigned long end, phys_addr_t phys_addr, pgprot_t prot)
|
|
{
|
|
p4d_t *p4d;
|
|
unsigned long next;
|
|
|
|
phys_addr -= addr;
|
|
p4d = p4d_alloc(&init_mm, pgd, addr);
|
|
if (!p4d)
|
|
return -ENOMEM;
|
|
do {
|
|
next = p4d_addr_end(addr, end);
|
|
|
|
if (ioremap_p4d_enabled() &&
|
|
((next - addr) == P4D_SIZE) &&
|
|
IS_ALIGNED(phys_addr + addr, P4D_SIZE)) {
|
|
if (p4d_set_huge(p4d, phys_addr + addr, prot))
|
|
continue;
|
|
}
|
|
|
|
if (ioremap_pud_range(p4d, addr, next, phys_addr + addr, prot))
|
|
return -ENOMEM;
|
|
} while (p4d++, addr = next, addr != end);
|
|
return 0;
|
|
}
|
|
|
|
int ioremap_page_range(unsigned long addr,
|
|
unsigned long end, phys_addr_t phys_addr, pgprot_t prot)
|
|
{
|
|
pgd_t *pgd;
|
|
unsigned long start;
|
|
unsigned long next;
|
|
int err;
|
|
|
|
might_sleep();
|
|
BUG_ON(addr >= end);
|
|
|
|
start = addr;
|
|
phys_addr -= addr;
|
|
pgd = pgd_offset_k(addr);
|
|
do {
|
|
next = pgd_addr_end(addr, end);
|
|
err = ioremap_p4d_range(pgd, addr, next, phys_addr+addr, prot);
|
|
if (err)
|
|
break;
|
|
} while (pgd++, addr = next, addr != end);
|
|
|
|
flush_cache_vmap(start, end);
|
|
|
|
return err;
|
|
}
|