mirror of
https://github.com/brain-hackers/linux-brain.git
synced 2024-06-09 23:36:23 +09:00
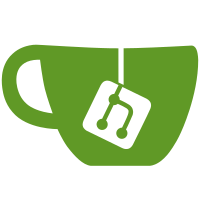
commit 412055398b9e67e07347a936fc4a6adddabe9cf4 upstream.
svcrdma expects that the payload falls precisely into the xdr_buf
page vector. This does not seem to be the case for
nfsd4_encode_readv().
This code is called only when fops->splice_read is missing or when
RQ_SPLICE_OK is clear, so it's not a noticeable problem in many
common cases.
Add new transport method: ->xpo_read_payload so that when a READ
payload does not fit exactly in rq_res's page vector, the XDR
encoder can inform the RPC transport exactly where that payload is,
without the payload's XDR pad.
That way, when a Write chunk is present, the transport knows what
byte range in the Reply message is supposed to be matched with the
chunk.
Note that the Linux NFS server implementation of NFS/RDMA can
currently handle only one Write chunk per RPC-over-RDMA message.
This simplifies the implementation of this fix.
Fixes: b042098063
("nfsd4: allow exotic read compounds")
Buglink: https://bugzilla.kernel.org/show_bug.cgi?id=198053
Signed-off-by: Chuck Lever <chuck.lever@oracle.com>
Cc: Timo Rothenpieler <timo@rothenpieler.org>
Signed-off-by: Greg Kroah-Hartman <gregkh@linuxfoundation.org>
215 lines
7.3 KiB
C
215 lines
7.3 KiB
C
/* SPDX-License-Identifier: GPL-2.0 OR BSD-3-Clause */
|
|
/*
|
|
* Copyright (c) 2005-2006 Network Appliance, Inc. All rights reserved.
|
|
*
|
|
* This software is available to you under a choice of one of two
|
|
* licenses. You may choose to be licensed under the terms of the GNU
|
|
* General Public License (GPL) Version 2, available from the file
|
|
* COPYING in the main directory of this source tree, or the BSD-type
|
|
* license below:
|
|
*
|
|
* Redistribution and use in source and binary forms, with or without
|
|
* modification, are permitted provided that the following conditions
|
|
* are met:
|
|
*
|
|
* Redistributions of source code must retain the above copyright
|
|
* notice, this list of conditions and the following disclaimer.
|
|
*
|
|
* Redistributions in binary form must reproduce the above
|
|
* copyright notice, this list of conditions and the following
|
|
* disclaimer in the documentation and/or other materials provided
|
|
* with the distribution.
|
|
*
|
|
* Neither the name of the Network Appliance, Inc. nor the names of
|
|
* its contributors may be used to endorse or promote products
|
|
* derived from this software without specific prior written
|
|
* permission.
|
|
*
|
|
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
|
|
* "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT
|
|
* LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR
|
|
* A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT
|
|
* OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL,
|
|
* SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT
|
|
* LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE,
|
|
* DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY
|
|
* THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
|
|
* (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
|
|
* OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
|
|
*
|
|
* Author: Tom Tucker <tom@opengridcomputing.com>
|
|
*/
|
|
|
|
#ifndef SVC_RDMA_H
|
|
#define SVC_RDMA_H
|
|
#include <linux/llist.h>
|
|
#include <linux/sunrpc/xdr.h>
|
|
#include <linux/sunrpc/svcsock.h>
|
|
#include <linux/sunrpc/rpc_rdma.h>
|
|
#include <rdma/ib_verbs.h>
|
|
#include <rdma/rdma_cm.h>
|
|
#define SVCRDMA_DEBUG
|
|
|
|
/* Default and maximum inline threshold sizes */
|
|
enum {
|
|
RPCRDMA_DEF_INLINE_THRESH = 4096,
|
|
RPCRDMA_MAX_INLINE_THRESH = 65536
|
|
};
|
|
|
|
/* RPC/RDMA parameters and stats */
|
|
extern unsigned int svcrdma_ord;
|
|
extern unsigned int svcrdma_max_requests;
|
|
extern unsigned int svcrdma_max_bc_requests;
|
|
extern unsigned int svcrdma_max_req_size;
|
|
|
|
extern atomic_t rdma_stat_recv;
|
|
extern atomic_t rdma_stat_read;
|
|
extern atomic_t rdma_stat_write;
|
|
extern atomic_t rdma_stat_sq_starve;
|
|
extern atomic_t rdma_stat_rq_starve;
|
|
extern atomic_t rdma_stat_rq_poll;
|
|
extern atomic_t rdma_stat_rq_prod;
|
|
extern atomic_t rdma_stat_sq_poll;
|
|
extern atomic_t rdma_stat_sq_prod;
|
|
|
|
struct svcxprt_rdma {
|
|
struct svc_xprt sc_xprt; /* SVC transport structure */
|
|
struct rdma_cm_id *sc_cm_id; /* RDMA connection id */
|
|
struct list_head sc_accept_q; /* Conn. waiting accept */
|
|
int sc_ord; /* RDMA read limit */
|
|
int sc_max_send_sges;
|
|
bool sc_snd_w_inv; /* OK to use Send With Invalidate */
|
|
|
|
atomic_t sc_sq_avail; /* SQEs ready to be consumed */
|
|
unsigned int sc_sq_depth; /* Depth of SQ */
|
|
__be32 sc_fc_credits; /* Forward credits */
|
|
u32 sc_max_requests; /* Max requests */
|
|
u32 sc_max_bc_requests;/* Backward credits */
|
|
int sc_max_req_size; /* Size of each RQ WR buf */
|
|
u8 sc_port_num;
|
|
|
|
struct ib_pd *sc_pd;
|
|
|
|
spinlock_t sc_send_lock;
|
|
struct list_head sc_send_ctxts;
|
|
spinlock_t sc_rw_ctxt_lock;
|
|
struct list_head sc_rw_ctxts;
|
|
|
|
struct list_head sc_rq_dto_q;
|
|
spinlock_t sc_rq_dto_lock;
|
|
struct ib_qp *sc_qp;
|
|
struct ib_cq *sc_rq_cq;
|
|
struct ib_cq *sc_sq_cq;
|
|
|
|
spinlock_t sc_lock; /* transport lock */
|
|
|
|
wait_queue_head_t sc_send_wait; /* SQ exhaustion waitlist */
|
|
unsigned long sc_flags;
|
|
struct list_head sc_read_complete_q;
|
|
struct work_struct sc_work;
|
|
|
|
struct llist_head sc_recv_ctxts;
|
|
};
|
|
/* sc_flags */
|
|
#define RDMAXPRT_CONN_PENDING 3
|
|
|
|
/*
|
|
* Default connection parameters
|
|
*/
|
|
enum {
|
|
RPCRDMA_LISTEN_BACKLOG = 10,
|
|
RPCRDMA_MAX_REQUESTS = 64,
|
|
RPCRDMA_MAX_BC_REQUESTS = 2,
|
|
};
|
|
|
|
#define RPCSVC_MAXPAYLOAD_RDMA RPCSVC_MAXPAYLOAD
|
|
|
|
struct svc_rdma_recv_ctxt {
|
|
struct llist_node rc_node;
|
|
struct list_head rc_list;
|
|
struct ib_recv_wr rc_recv_wr;
|
|
struct ib_cqe rc_cqe;
|
|
struct ib_sge rc_recv_sge;
|
|
void *rc_recv_buf;
|
|
struct xdr_buf rc_arg;
|
|
bool rc_temp;
|
|
u32 rc_byte_len;
|
|
unsigned int rc_page_count;
|
|
unsigned int rc_hdr_count;
|
|
u32 rc_inv_rkey;
|
|
unsigned int rc_read_payload_offset;
|
|
unsigned int rc_read_payload_length;
|
|
struct page *rc_pages[RPCSVC_MAXPAGES];
|
|
};
|
|
|
|
struct svc_rdma_send_ctxt {
|
|
struct list_head sc_list;
|
|
struct ib_send_wr sc_send_wr;
|
|
struct ib_cqe sc_cqe;
|
|
void *sc_xprt_buf;
|
|
int sc_page_count;
|
|
int sc_cur_sge_no;
|
|
struct page *sc_pages[RPCSVC_MAXPAGES];
|
|
struct ib_sge sc_sges[];
|
|
};
|
|
|
|
/* svc_rdma_backchannel.c */
|
|
extern int svc_rdma_handle_bc_reply(struct rpc_xprt *xprt,
|
|
__be32 *rdma_resp,
|
|
struct xdr_buf *rcvbuf);
|
|
|
|
/* svc_rdma_recvfrom.c */
|
|
extern void svc_rdma_recv_ctxts_destroy(struct svcxprt_rdma *rdma);
|
|
extern bool svc_rdma_post_recvs(struct svcxprt_rdma *rdma);
|
|
extern void svc_rdma_recv_ctxt_put(struct svcxprt_rdma *rdma,
|
|
struct svc_rdma_recv_ctxt *ctxt);
|
|
extern void svc_rdma_flush_recv_queues(struct svcxprt_rdma *rdma);
|
|
extern void svc_rdma_release_rqst(struct svc_rqst *rqstp);
|
|
extern int svc_rdma_recvfrom(struct svc_rqst *);
|
|
|
|
/* svc_rdma_rw.c */
|
|
extern void svc_rdma_destroy_rw_ctxts(struct svcxprt_rdma *rdma);
|
|
extern int svc_rdma_recv_read_chunk(struct svcxprt_rdma *rdma,
|
|
struct svc_rqst *rqstp,
|
|
struct svc_rdma_recv_ctxt *head, __be32 *p);
|
|
extern int svc_rdma_send_write_chunk(struct svcxprt_rdma *rdma,
|
|
__be32 *wr_ch, struct xdr_buf *xdr,
|
|
unsigned int offset,
|
|
unsigned long length);
|
|
extern int svc_rdma_send_reply_chunk(struct svcxprt_rdma *rdma,
|
|
__be32 *rp_ch, bool writelist,
|
|
struct xdr_buf *xdr);
|
|
|
|
/* svc_rdma_sendto.c */
|
|
extern void svc_rdma_send_ctxts_destroy(struct svcxprt_rdma *rdma);
|
|
extern struct svc_rdma_send_ctxt *
|
|
svc_rdma_send_ctxt_get(struct svcxprt_rdma *rdma);
|
|
extern void svc_rdma_send_ctxt_put(struct svcxprt_rdma *rdma,
|
|
struct svc_rdma_send_ctxt *ctxt);
|
|
extern int svc_rdma_send(struct svcxprt_rdma *rdma, struct ib_send_wr *wr);
|
|
extern void svc_rdma_sync_reply_hdr(struct svcxprt_rdma *rdma,
|
|
struct svc_rdma_send_ctxt *ctxt,
|
|
unsigned int len);
|
|
extern int svc_rdma_map_reply_msg(struct svcxprt_rdma *rdma,
|
|
struct svc_rdma_send_ctxt *ctxt,
|
|
struct xdr_buf *xdr, __be32 *wr_lst);
|
|
extern int svc_rdma_sendto(struct svc_rqst *);
|
|
extern int svc_rdma_read_payload(struct svc_rqst *rqstp, unsigned int offset,
|
|
unsigned int length);
|
|
|
|
/* svc_rdma_transport.c */
|
|
extern int svc_rdma_create_listen(struct svc_serv *, int, struct sockaddr *);
|
|
extern void svc_sq_reap(struct svcxprt_rdma *);
|
|
extern void svc_rq_reap(struct svcxprt_rdma *);
|
|
|
|
extern struct svc_xprt_class svc_rdma_class;
|
|
#ifdef CONFIG_SUNRPC_BACKCHANNEL
|
|
extern struct svc_xprt_class svc_rdma_bc_class;
|
|
#endif
|
|
|
|
/* svc_rdma.c */
|
|
extern int svc_rdma_init(void);
|
|
extern void svc_rdma_cleanup(void);
|
|
|
|
#endif
|