mirror of
https://github.com/brain-hackers/linux-brain.git
synced 2024-06-09 23:36:23 +09:00
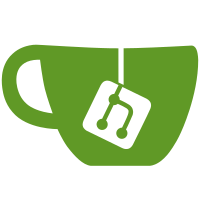
Update the time(r) core files files with the correct SPDX license identifier based on the license text in the file itself. The SPDX identifier is a legally binding shorthand, which can be used instead of the full boiler plate text. This work is based on a script and data from Philippe Ombredanne, Kate Stewart and myself. The data has been created with two independent license scanners and manual inspection. The following files do not contain any direct license information and have been omitted from the big initial SPDX changes: timeconst.bc: The .bc files were not touched time.c, timer.c, timekeeping.c: Licence was deduced from EXPORT_SYMBOL_GPL As those files do not contain direct license references they fall under the project license, i.e. GPL V2 only. Signed-off-by: Thomas Gleixner <tglx@linutronix.de> Acked-by: Kees Cook <keescook@chromium.org> Acked-by: Ingo Molnar <mingo@kernel.org> Acked-by: John Stultz <john.stultz@linaro.org> Acked-by: Corey Minyard <cminyard@mvista.com> Cc: Peter Zijlstra <peterz@infradead.org> Cc: Kate Stewart <kstewart@linuxfoundation.org> Cc: Philippe Ombredanne <pombredanne@nexb.com> Cc: Russell King <rmk+kernel@armlinux.org.uk> Cc: Richard Cochran <richardcochran@gmail.com> Cc: Nicolas Pitre <nicolas.pitre@linaro.org> Cc: David Riley <davidriley@chromium.org> Cc: Colin Cross <ccross@android.com> Cc: Mark Brown <broonie@kernel.org> Cc: H. Peter Anvin <hpa@zytor.com> Cc: Paul E. McKenney <paulmck@linux.vnet.ibm.com> Link: https://lkml.kernel.org/r/20181031182252.879109557@linutronix.de
84 lines
2.2 KiB
C
84 lines
2.2 KiB
C
// SPDX-License-Identifier: GPL-2.0+
|
|
/*
|
|
* debugfs file to track time spent in suspend
|
|
*
|
|
* Copyright (c) 2011, Google, Inc.
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful, but WITHOUT
|
|
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
|
|
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License for
|
|
* more details.
|
|
*/
|
|
|
|
#include <linux/debugfs.h>
|
|
#include <linux/err.h>
|
|
#include <linux/init.h>
|
|
#include <linux/kernel.h>
|
|
#include <linux/seq_file.h>
|
|
#include <linux/suspend.h>
|
|
#include <linux/time.h>
|
|
|
|
#include "timekeeping_internal.h"
|
|
|
|
#define NUM_BINS 32
|
|
|
|
static unsigned int sleep_time_bin[NUM_BINS] = {0};
|
|
|
|
static int tk_debug_show_sleep_time(struct seq_file *s, void *data)
|
|
{
|
|
unsigned int bin;
|
|
seq_puts(s, " time (secs) count\n");
|
|
seq_puts(s, "------------------------------\n");
|
|
for (bin = 0; bin < 32; bin++) {
|
|
if (sleep_time_bin[bin] == 0)
|
|
continue;
|
|
seq_printf(s, "%10u - %-10u %4u\n",
|
|
bin ? 1 << (bin - 1) : 0, 1 << bin,
|
|
sleep_time_bin[bin]);
|
|
}
|
|
return 0;
|
|
}
|
|
|
|
static int tk_debug_sleep_time_open(struct inode *inode, struct file *file)
|
|
{
|
|
return single_open(file, tk_debug_show_sleep_time, NULL);
|
|
}
|
|
|
|
static const struct file_operations tk_debug_sleep_time_fops = {
|
|
.open = tk_debug_sleep_time_open,
|
|
.read = seq_read,
|
|
.llseek = seq_lseek,
|
|
.release = single_release,
|
|
};
|
|
|
|
static int __init tk_debug_sleep_time_init(void)
|
|
{
|
|
struct dentry *d;
|
|
|
|
d = debugfs_create_file("sleep_time", 0444, NULL, NULL,
|
|
&tk_debug_sleep_time_fops);
|
|
if (!d) {
|
|
pr_err("Failed to create sleep_time debug file\n");
|
|
return -ENOMEM;
|
|
}
|
|
|
|
return 0;
|
|
}
|
|
late_initcall(tk_debug_sleep_time_init);
|
|
|
|
void tk_debug_account_sleep_time(const struct timespec64 *t)
|
|
{
|
|
/* Cap bin index so we don't overflow the array */
|
|
int bin = min(fls(t->tv_sec), NUM_BINS-1);
|
|
|
|
sleep_time_bin[bin]++;
|
|
pm_deferred_pr_dbg("Timekeeping suspended for %lld.%03lu seconds\n",
|
|
(s64)t->tv_sec, t->tv_nsec / NSEC_PER_MSEC);
|
|
}
|
|
|