mirror of
https://github.com/brain-hackers/linux-brain.git
synced 2024-06-09 23:36:23 +09:00
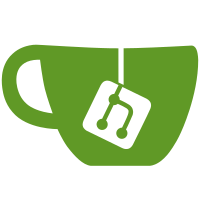
Based on 1 normalized pattern(s): this program is free software you can redistribute it and or modify it under the terms of the gnu general public license version 2 as published by the free software foundation this program is distributed in the hope that it will be useful but without any warranty without even the implied warranty of merchantability or fitness for a particular purpose see the gnu general public license for more details you should have received a copy of the gnu general public license along with this program if not write to the free software foundation inc 51 franklin st fifth floor boston ma 02110 1301 usa extracted by the scancode license scanner the SPDX license identifier GPL-2.0-only has been chosen to replace the boilerplate/reference in 246 file(s). Signed-off-by: Thomas Gleixner <tglx@linutronix.de> Reviewed-by: Alexios Zavras <alexios.zavras@intel.com> Reviewed-by: Allison Randal <allison@lohutok.net> Cc: linux-spdx@vger.kernel.org Link: https://lkml.kernel.org/r/20190530000436.674189849@linutronix.de Signed-off-by: Greg Kroah-Hartman <gregkh@linuxfoundation.org>
76 lines
1.9 KiB
C
76 lines
1.9 KiB
C
// SPDX-License-Identifier: GPL-2.0-only
|
|
/*
|
|
* MSDI IP block reset
|
|
*
|
|
* Copyright (C) 2012 Texas Instruments, Inc.
|
|
* Paul Walmsley
|
|
*
|
|
* XXX What about pad muxing?
|
|
*/
|
|
|
|
#include <linux/kernel.h>
|
|
#include <linux/err.h>
|
|
|
|
#include "prm.h"
|
|
#include "common.h"
|
|
#include "control.h"
|
|
#include "omap_hwmod.h"
|
|
#include "omap_device.h"
|
|
#include "mmc.h"
|
|
|
|
/*
|
|
* MSDI_CON_OFFSET: offset in bytes of the MSDI IP block's CON register
|
|
* from the IP block's base address
|
|
*/
|
|
#define MSDI_CON_OFFSET 0x0c
|
|
|
|
/* Register bitfields in the CON register */
|
|
#define MSDI_CON_POW_MASK BIT(11)
|
|
#define MSDI_CON_CLKD_MASK (0x3f << 0)
|
|
#define MSDI_CON_CLKD_SHIFT 0
|
|
|
|
/* MSDI_TARGET_RESET_CLKD: clock divisor to use throughout the reset */
|
|
#define MSDI_TARGET_RESET_CLKD 0x3ff
|
|
|
|
/**
|
|
* omap_msdi_reset - reset the MSDI IP block
|
|
* @oh: struct omap_hwmod *
|
|
*
|
|
* The MSDI IP block on OMAP2420 has to have both the POW and CLKD
|
|
* fields set inside its CON register for a reset to complete
|
|
* successfully. This is not documented in the TRM. For CLKD, we use
|
|
* the value that results in the lowest possible clock rate, to attempt
|
|
* to avoid disturbing any cards.
|
|
*/
|
|
int omap_msdi_reset(struct omap_hwmod *oh)
|
|
{
|
|
u16 v = 0;
|
|
int c = 0;
|
|
|
|
/* Write to the SOFTRESET bit */
|
|
omap_hwmod_softreset(oh);
|
|
|
|
/* Enable the MSDI core and internal clock */
|
|
v |= MSDI_CON_POW_MASK;
|
|
v |= MSDI_TARGET_RESET_CLKD << MSDI_CON_CLKD_SHIFT;
|
|
omap_hwmod_write(v, oh, MSDI_CON_OFFSET);
|
|
|
|
/* Poll on RESETDONE bit */
|
|
omap_test_timeout((omap_hwmod_read(oh, oh->class->sysc->syss_offs)
|
|
& SYSS_RESETDONE_MASK),
|
|
MAX_MODULE_SOFTRESET_WAIT, c);
|
|
|
|
if (c == MAX_MODULE_SOFTRESET_WAIT)
|
|
pr_warn("%s: %s: softreset failed (waited %d usec)\n",
|
|
__func__, oh->name, MAX_MODULE_SOFTRESET_WAIT);
|
|
else
|
|
pr_debug("%s: %s: softreset in %d usec\n", __func__,
|
|
oh->name, c);
|
|
|
|
/* Disable the MSDI internal clock */
|
|
v &= ~MSDI_CON_CLKD_MASK;
|
|
omap_hwmod_write(v, oh, MSDI_CON_OFFSET);
|
|
|
|
return 0;
|
|
}
|