mirror of
https://github.com/brain-hackers/linux-brain.git
synced 2024-06-09 23:36:23 +09:00
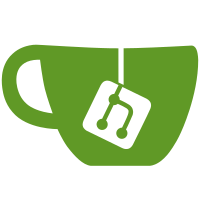
Remove the verbose license text from XFS files and replace them with SPDX tags. This does not change the license of any of the code, merely refers to the common, up-to-date license files in LICENSES/ This change was mostly scripted. fs/xfs/Makefile and fs/xfs/libxfs/xfs_fs.h were modified by hand, the rest were detected and modified by the following command: for f in `git grep -l "GNU General" fs/xfs/` ; do echo $f cat $f | awk -f hdr.awk > $f.new mv -f $f.new $f done And the hdr.awk script that did the modification (including detecting the difference between GPL-2.0 and GPL-2.0+ licenses) is as follows: $ cat hdr.awk BEGIN { hdr = 1.0 tag = "GPL-2.0" str = "" } /^ \* This program is free software/ { hdr = 2.0; next } /any later version./ { tag = "GPL-2.0+" next } /^ \*\// { if (hdr > 0.0) { print "// SPDX-License-Identifier: " tag print str print $0 str="" hdr = 0.0 next } print $0 next } /^ \* / { if (hdr > 1.0) next if (hdr > 0.0) { if (str != "") str = str "\n" str = str $0 next } print $0 next } /^ \*/ { if (hdr > 0.0) next print $0 next } // { if (hdr > 0.0) { if (str != "") str = str "\n" str = str $0 next } print $0 } END { } $ Signed-off-by: Dave Chinner <dchinner@redhat.com> Reviewed-by: Darrick J. Wong <darrick.wong@oracle.com> Signed-off-by: Darrick J. Wong <darrick.wong@oracle.com>
126 lines
4.4 KiB
C
126 lines
4.4 KiB
C
// SPDX-License-Identifier: GPL-2.0
|
|
/*
|
|
* Copyright (c) 2000-2005 Silicon Graphics, Inc.
|
|
* Copyright (c) 2013 Red Hat, Inc.
|
|
* All Rights Reserved.
|
|
*/
|
|
#ifndef __XFS_SHARED_H__
|
|
#define __XFS_SHARED_H__
|
|
|
|
/*
|
|
* Definitions shared between kernel and userspace that don't fit into any other
|
|
* header file that is shared with userspace.
|
|
*/
|
|
struct xfs_ifork;
|
|
struct xfs_buf;
|
|
struct xfs_buf_ops;
|
|
struct xfs_mount;
|
|
struct xfs_trans;
|
|
struct xfs_inode;
|
|
|
|
/*
|
|
* Buffer verifier operations are widely used, including userspace tools
|
|
*/
|
|
extern const struct xfs_buf_ops xfs_agf_buf_ops;
|
|
extern const struct xfs_buf_ops xfs_agi_buf_ops;
|
|
extern const struct xfs_buf_ops xfs_agf_buf_ops;
|
|
extern const struct xfs_buf_ops xfs_agfl_buf_ops;
|
|
extern const struct xfs_buf_ops xfs_allocbt_buf_ops;
|
|
extern const struct xfs_buf_ops xfs_rmapbt_buf_ops;
|
|
extern const struct xfs_buf_ops xfs_refcountbt_buf_ops;
|
|
extern const struct xfs_buf_ops xfs_attr3_leaf_buf_ops;
|
|
extern const struct xfs_buf_ops xfs_attr3_rmt_buf_ops;
|
|
extern const struct xfs_buf_ops xfs_bmbt_buf_ops;
|
|
extern const struct xfs_buf_ops xfs_da3_node_buf_ops;
|
|
extern const struct xfs_buf_ops xfs_dquot_buf_ops;
|
|
extern const struct xfs_buf_ops xfs_symlink_buf_ops;
|
|
extern const struct xfs_buf_ops xfs_agi_buf_ops;
|
|
extern const struct xfs_buf_ops xfs_inobt_buf_ops;
|
|
extern const struct xfs_buf_ops xfs_inode_buf_ops;
|
|
extern const struct xfs_buf_ops xfs_inode_buf_ra_ops;
|
|
extern const struct xfs_buf_ops xfs_dquot_buf_ops;
|
|
extern const struct xfs_buf_ops xfs_dquot_buf_ra_ops;
|
|
extern const struct xfs_buf_ops xfs_sb_buf_ops;
|
|
extern const struct xfs_buf_ops xfs_sb_quiet_buf_ops;
|
|
extern const struct xfs_buf_ops xfs_symlink_buf_ops;
|
|
extern const struct xfs_buf_ops xfs_rtbuf_ops;
|
|
|
|
/* log size calculation functions */
|
|
int xfs_log_calc_unit_res(struct xfs_mount *mp, int unit_bytes);
|
|
int xfs_log_calc_minimum_size(struct xfs_mount *);
|
|
|
|
struct xfs_trans_res;
|
|
void xfs_log_get_max_trans_res(struct xfs_mount *mp,
|
|
struct xfs_trans_res *max_resp);
|
|
|
|
/*
|
|
* Values for t_flags.
|
|
*/
|
|
#define XFS_TRANS_DIRTY 0x01 /* something needs to be logged */
|
|
#define XFS_TRANS_SB_DIRTY 0x02 /* superblock is modified */
|
|
#define XFS_TRANS_PERM_LOG_RES 0x04 /* xact took a permanent log res */
|
|
#define XFS_TRANS_SYNC 0x08 /* make commit synchronous */
|
|
#define XFS_TRANS_DQ_DIRTY 0x10 /* at least one dquot in trx dirty */
|
|
#define XFS_TRANS_RESERVE 0x20 /* OK to use reserved data blocks */
|
|
#define XFS_TRANS_NO_WRITECOUNT 0x40 /* do not elevate SB writecount */
|
|
#define XFS_TRANS_NOFS 0x80 /* pass KM_NOFS to kmem_alloc */
|
|
|
|
/*
|
|
* Field values for xfs_trans_mod_sb.
|
|
*/
|
|
#define XFS_TRANS_SB_ICOUNT 0x00000001
|
|
#define XFS_TRANS_SB_IFREE 0x00000002
|
|
#define XFS_TRANS_SB_FDBLOCKS 0x00000004
|
|
#define XFS_TRANS_SB_RES_FDBLOCKS 0x00000008
|
|
#define XFS_TRANS_SB_FREXTENTS 0x00000010
|
|
#define XFS_TRANS_SB_RES_FREXTENTS 0x00000020
|
|
#define XFS_TRANS_SB_DBLOCKS 0x00000040
|
|
#define XFS_TRANS_SB_AGCOUNT 0x00000080
|
|
#define XFS_TRANS_SB_IMAXPCT 0x00000100
|
|
#define XFS_TRANS_SB_REXTSIZE 0x00000200
|
|
#define XFS_TRANS_SB_RBMBLOCKS 0x00000400
|
|
#define XFS_TRANS_SB_RBLOCKS 0x00000800
|
|
#define XFS_TRANS_SB_REXTENTS 0x00001000
|
|
#define XFS_TRANS_SB_REXTSLOG 0x00002000
|
|
|
|
/*
|
|
* Here we centralize the specification of XFS meta-data buffer reference count
|
|
* values. This determines how hard the buffer cache tries to hold onto the
|
|
* buffer.
|
|
*/
|
|
#define XFS_AGF_REF 4
|
|
#define XFS_AGI_REF 4
|
|
#define XFS_AGFL_REF 3
|
|
#define XFS_INO_BTREE_REF 3
|
|
#define XFS_ALLOC_BTREE_REF 2
|
|
#define XFS_BMAP_BTREE_REF 2
|
|
#define XFS_RMAP_BTREE_REF 2
|
|
#define XFS_DIR_BTREE_REF 2
|
|
#define XFS_INO_REF 2
|
|
#define XFS_ATTR_BTREE_REF 1
|
|
#define XFS_DQUOT_REF 1
|
|
#define XFS_REFC_BTREE_REF 1
|
|
#define XFS_SSB_REF 0
|
|
|
|
/*
|
|
* Flags for xfs_trans_ichgtime().
|
|
*/
|
|
#define XFS_ICHGTIME_MOD 0x1 /* data fork modification timestamp */
|
|
#define XFS_ICHGTIME_CHG 0x2 /* inode field change timestamp */
|
|
#define XFS_ICHGTIME_CREATE 0x4 /* inode create timestamp */
|
|
|
|
|
|
/*
|
|
* Symlink decoding/encoding functions
|
|
*/
|
|
int xfs_symlink_blocks(struct xfs_mount *mp, int pathlen);
|
|
int xfs_symlink_hdr_set(struct xfs_mount *mp, xfs_ino_t ino, uint32_t offset,
|
|
uint32_t size, struct xfs_buf *bp);
|
|
bool xfs_symlink_hdr_ok(xfs_ino_t ino, uint32_t offset,
|
|
uint32_t size, struct xfs_buf *bp);
|
|
void xfs_symlink_local_to_remote(struct xfs_trans *tp, struct xfs_buf *bp,
|
|
struct xfs_inode *ip, struct xfs_ifork *ifp);
|
|
xfs_failaddr_t xfs_symlink_shortform_verify(struct xfs_inode *ip);
|
|
|
|
#endif /* __XFS_SHARED_H__ */
|