mirror of
https://github.com/brain-hackers/linux-brain.git
synced 2024-06-09 15:26:21 +09:00
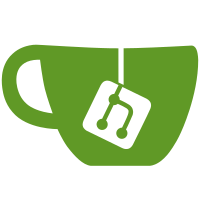
Remove the verbose license text from XFS files and replace them with SPDX tags. This does not change the license of any of the code, merely refers to the common, up-to-date license files in LICENSES/ This change was mostly scripted. fs/xfs/Makefile and fs/xfs/libxfs/xfs_fs.h were modified by hand, the rest were detected and modified by the following command: for f in `git grep -l "GNU General" fs/xfs/` ; do echo $f cat $f | awk -f hdr.awk > $f.new mv -f $f.new $f done And the hdr.awk script that did the modification (including detecting the difference between GPL-2.0 and GPL-2.0+ licenses) is as follows: $ cat hdr.awk BEGIN { hdr = 1.0 tag = "GPL-2.0" str = "" } /^ \* This program is free software/ { hdr = 2.0; next } /any later version./ { tag = "GPL-2.0+" next } /^ \*\// { if (hdr > 0.0) { print "// SPDX-License-Identifier: " tag print str print $0 str="" hdr = 0.0 next } print $0 next } /^ \* / { if (hdr > 1.0) next if (hdr > 0.0) { if (str != "") str = str "\n" str = str $0 next } print $0 next } /^ \*/ { if (hdr > 0.0) next print $0 next } // { if (hdr > 0.0) { if (str != "") str = str "\n" str = str $0 next } print $0 } END { } $ Signed-off-by: Dave Chinner <dchinner@redhat.com> Reviewed-by: Darrick J. Wong <darrick.wong@oracle.com> Signed-off-by: Darrick J. Wong <darrick.wong@oracle.com>
653 lines
16 KiB
C
653 lines
16 KiB
C
// SPDX-License-Identifier: GPL-2.0
|
|
/*
|
|
* Copyright (c) 2000-2005 Silicon Graphics, Inc.
|
|
* Copyright (c) 2013 Red Hat, Inc.
|
|
* All Rights Reserved.
|
|
*/
|
|
#include "xfs.h"
|
|
#include "xfs_fs.h"
|
|
#include "xfs_shared.h"
|
|
#include "xfs_format.h"
|
|
#include "xfs_log_format.h"
|
|
#include "xfs_trans_resv.h"
|
|
#include "xfs_bit.h"
|
|
#include "xfs_mount.h"
|
|
#include "xfs_defer.h"
|
|
#include "xfs_da_format.h"
|
|
#include "xfs_da_btree.h"
|
|
#include "xfs_inode.h"
|
|
#include "xfs_alloc.h"
|
|
#include "xfs_trans.h"
|
|
#include "xfs_inode_item.h"
|
|
#include "xfs_bmap.h"
|
|
#include "xfs_bmap_util.h"
|
|
#include "xfs_attr.h"
|
|
#include "xfs_attr_leaf.h"
|
|
#include "xfs_attr_remote.h"
|
|
#include "xfs_trans_space.h"
|
|
#include "xfs_trace.h"
|
|
#include "xfs_cksum.h"
|
|
#include "xfs_buf_item.h"
|
|
#include "xfs_error.h"
|
|
|
|
#define ATTR_RMTVALUE_MAPSIZE 1 /* # of map entries at once */
|
|
|
|
/*
|
|
* Each contiguous block has a header, so it is not just a simple attribute
|
|
* length to FSB conversion.
|
|
*/
|
|
int
|
|
xfs_attr3_rmt_blocks(
|
|
struct xfs_mount *mp,
|
|
int attrlen)
|
|
{
|
|
if (xfs_sb_version_hascrc(&mp->m_sb)) {
|
|
int buflen = XFS_ATTR3_RMT_BUF_SPACE(mp, mp->m_sb.sb_blocksize);
|
|
return (attrlen + buflen - 1) / buflen;
|
|
}
|
|
return XFS_B_TO_FSB(mp, attrlen);
|
|
}
|
|
|
|
/*
|
|
* Checking of the remote attribute header is split into two parts. The verifier
|
|
* does CRC, location and bounds checking, the unpacking function checks the
|
|
* attribute parameters and owner.
|
|
*/
|
|
static xfs_failaddr_t
|
|
xfs_attr3_rmt_hdr_ok(
|
|
void *ptr,
|
|
xfs_ino_t ino,
|
|
uint32_t offset,
|
|
uint32_t size,
|
|
xfs_daddr_t bno)
|
|
{
|
|
struct xfs_attr3_rmt_hdr *rmt = ptr;
|
|
|
|
if (bno != be64_to_cpu(rmt->rm_blkno))
|
|
return __this_address;
|
|
if (offset != be32_to_cpu(rmt->rm_offset))
|
|
return __this_address;
|
|
if (size != be32_to_cpu(rmt->rm_bytes))
|
|
return __this_address;
|
|
if (ino != be64_to_cpu(rmt->rm_owner))
|
|
return __this_address;
|
|
|
|
/* ok */
|
|
return NULL;
|
|
}
|
|
|
|
static xfs_failaddr_t
|
|
xfs_attr3_rmt_verify(
|
|
struct xfs_mount *mp,
|
|
void *ptr,
|
|
int fsbsize,
|
|
xfs_daddr_t bno)
|
|
{
|
|
struct xfs_attr3_rmt_hdr *rmt = ptr;
|
|
|
|
if (!xfs_sb_version_hascrc(&mp->m_sb))
|
|
return __this_address;
|
|
if (rmt->rm_magic != cpu_to_be32(XFS_ATTR3_RMT_MAGIC))
|
|
return __this_address;
|
|
if (!uuid_equal(&rmt->rm_uuid, &mp->m_sb.sb_meta_uuid))
|
|
return __this_address;
|
|
if (be64_to_cpu(rmt->rm_blkno) != bno)
|
|
return __this_address;
|
|
if (be32_to_cpu(rmt->rm_bytes) > fsbsize - sizeof(*rmt))
|
|
return __this_address;
|
|
if (be32_to_cpu(rmt->rm_offset) +
|
|
be32_to_cpu(rmt->rm_bytes) > XFS_XATTR_SIZE_MAX)
|
|
return __this_address;
|
|
if (rmt->rm_owner == 0)
|
|
return __this_address;
|
|
|
|
return NULL;
|
|
}
|
|
|
|
static int
|
|
__xfs_attr3_rmt_read_verify(
|
|
struct xfs_buf *bp,
|
|
bool check_crc,
|
|
xfs_failaddr_t *failaddr)
|
|
{
|
|
struct xfs_mount *mp = bp->b_target->bt_mount;
|
|
char *ptr;
|
|
int len;
|
|
xfs_daddr_t bno;
|
|
int blksize = mp->m_attr_geo->blksize;
|
|
|
|
/* no verification of non-crc buffers */
|
|
if (!xfs_sb_version_hascrc(&mp->m_sb))
|
|
return 0;
|
|
|
|
ptr = bp->b_addr;
|
|
bno = bp->b_bn;
|
|
len = BBTOB(bp->b_length);
|
|
ASSERT(len >= blksize);
|
|
|
|
while (len > 0) {
|
|
if (check_crc &&
|
|
!xfs_verify_cksum(ptr, blksize, XFS_ATTR3_RMT_CRC_OFF)) {
|
|
*failaddr = __this_address;
|
|
return -EFSBADCRC;
|
|
}
|
|
*failaddr = xfs_attr3_rmt_verify(mp, ptr, blksize, bno);
|
|
if (*failaddr)
|
|
return -EFSCORRUPTED;
|
|
len -= blksize;
|
|
ptr += blksize;
|
|
bno += BTOBB(blksize);
|
|
}
|
|
|
|
if (len != 0) {
|
|
*failaddr = __this_address;
|
|
return -EFSCORRUPTED;
|
|
}
|
|
|
|
return 0;
|
|
}
|
|
|
|
static void
|
|
xfs_attr3_rmt_read_verify(
|
|
struct xfs_buf *bp)
|
|
{
|
|
xfs_failaddr_t fa;
|
|
int error;
|
|
|
|
error = __xfs_attr3_rmt_read_verify(bp, true, &fa);
|
|
if (error)
|
|
xfs_verifier_error(bp, error, fa);
|
|
}
|
|
|
|
static xfs_failaddr_t
|
|
xfs_attr3_rmt_verify_struct(
|
|
struct xfs_buf *bp)
|
|
{
|
|
xfs_failaddr_t fa;
|
|
int error;
|
|
|
|
error = __xfs_attr3_rmt_read_verify(bp, false, &fa);
|
|
return error ? fa : NULL;
|
|
}
|
|
|
|
static void
|
|
xfs_attr3_rmt_write_verify(
|
|
struct xfs_buf *bp)
|
|
{
|
|
struct xfs_mount *mp = bp->b_target->bt_mount;
|
|
xfs_failaddr_t fa;
|
|
int blksize = mp->m_attr_geo->blksize;
|
|
char *ptr;
|
|
int len;
|
|
xfs_daddr_t bno;
|
|
|
|
/* no verification of non-crc buffers */
|
|
if (!xfs_sb_version_hascrc(&mp->m_sb))
|
|
return;
|
|
|
|
ptr = bp->b_addr;
|
|
bno = bp->b_bn;
|
|
len = BBTOB(bp->b_length);
|
|
ASSERT(len >= blksize);
|
|
|
|
while (len > 0) {
|
|
struct xfs_attr3_rmt_hdr *rmt = (struct xfs_attr3_rmt_hdr *)ptr;
|
|
|
|
fa = xfs_attr3_rmt_verify(mp, ptr, blksize, bno);
|
|
if (fa) {
|
|
xfs_verifier_error(bp, -EFSCORRUPTED, fa);
|
|
return;
|
|
}
|
|
|
|
/*
|
|
* Ensure we aren't writing bogus LSNs to disk. See
|
|
* xfs_attr3_rmt_hdr_set() for the explanation.
|
|
*/
|
|
if (rmt->rm_lsn != cpu_to_be64(NULLCOMMITLSN)) {
|
|
xfs_verifier_error(bp, -EFSCORRUPTED, __this_address);
|
|
return;
|
|
}
|
|
xfs_update_cksum(ptr, blksize, XFS_ATTR3_RMT_CRC_OFF);
|
|
|
|
len -= blksize;
|
|
ptr += blksize;
|
|
bno += BTOBB(blksize);
|
|
}
|
|
|
|
if (len != 0)
|
|
xfs_verifier_error(bp, -EFSCORRUPTED, __this_address);
|
|
}
|
|
|
|
const struct xfs_buf_ops xfs_attr3_rmt_buf_ops = {
|
|
.name = "xfs_attr3_rmt",
|
|
.verify_read = xfs_attr3_rmt_read_verify,
|
|
.verify_write = xfs_attr3_rmt_write_verify,
|
|
.verify_struct = xfs_attr3_rmt_verify_struct,
|
|
};
|
|
|
|
STATIC int
|
|
xfs_attr3_rmt_hdr_set(
|
|
struct xfs_mount *mp,
|
|
void *ptr,
|
|
xfs_ino_t ino,
|
|
uint32_t offset,
|
|
uint32_t size,
|
|
xfs_daddr_t bno)
|
|
{
|
|
struct xfs_attr3_rmt_hdr *rmt = ptr;
|
|
|
|
if (!xfs_sb_version_hascrc(&mp->m_sb))
|
|
return 0;
|
|
|
|
rmt->rm_magic = cpu_to_be32(XFS_ATTR3_RMT_MAGIC);
|
|
rmt->rm_offset = cpu_to_be32(offset);
|
|
rmt->rm_bytes = cpu_to_be32(size);
|
|
uuid_copy(&rmt->rm_uuid, &mp->m_sb.sb_meta_uuid);
|
|
rmt->rm_owner = cpu_to_be64(ino);
|
|
rmt->rm_blkno = cpu_to_be64(bno);
|
|
|
|
/*
|
|
* Remote attribute blocks are written synchronously, so we don't
|
|
* have an LSN that we can stamp in them that makes any sense to log
|
|
* recovery. To ensure that log recovery handles overwrites of these
|
|
* blocks sanely (i.e. once they've been freed and reallocated as some
|
|
* other type of metadata) we need to ensure that the LSN has a value
|
|
* that tells log recovery to ignore the LSN and overwrite the buffer
|
|
* with whatever is in it's log. To do this, we use the magic
|
|
* NULLCOMMITLSN to indicate that the LSN is invalid.
|
|
*/
|
|
rmt->rm_lsn = cpu_to_be64(NULLCOMMITLSN);
|
|
|
|
return sizeof(struct xfs_attr3_rmt_hdr);
|
|
}
|
|
|
|
/*
|
|
* Helper functions to copy attribute data in and out of the one disk extents
|
|
*/
|
|
STATIC int
|
|
xfs_attr_rmtval_copyout(
|
|
struct xfs_mount *mp,
|
|
struct xfs_buf *bp,
|
|
xfs_ino_t ino,
|
|
int *offset,
|
|
int *valuelen,
|
|
uint8_t **dst)
|
|
{
|
|
char *src = bp->b_addr;
|
|
xfs_daddr_t bno = bp->b_bn;
|
|
int len = BBTOB(bp->b_length);
|
|
int blksize = mp->m_attr_geo->blksize;
|
|
|
|
ASSERT(len >= blksize);
|
|
|
|
while (len > 0 && *valuelen > 0) {
|
|
int hdr_size = 0;
|
|
int byte_cnt = XFS_ATTR3_RMT_BUF_SPACE(mp, blksize);
|
|
|
|
byte_cnt = min(*valuelen, byte_cnt);
|
|
|
|
if (xfs_sb_version_hascrc(&mp->m_sb)) {
|
|
if (xfs_attr3_rmt_hdr_ok(src, ino, *offset,
|
|
byte_cnt, bno)) {
|
|
xfs_alert(mp,
|
|
"remote attribute header mismatch bno/off/len/owner (0x%llx/0x%x/Ox%x/0x%llx)",
|
|
bno, *offset, byte_cnt, ino);
|
|
return -EFSCORRUPTED;
|
|
}
|
|
hdr_size = sizeof(struct xfs_attr3_rmt_hdr);
|
|
}
|
|
|
|
memcpy(*dst, src + hdr_size, byte_cnt);
|
|
|
|
/* roll buffer forwards */
|
|
len -= blksize;
|
|
src += blksize;
|
|
bno += BTOBB(blksize);
|
|
|
|
/* roll attribute data forwards */
|
|
*valuelen -= byte_cnt;
|
|
*dst += byte_cnt;
|
|
*offset += byte_cnt;
|
|
}
|
|
return 0;
|
|
}
|
|
|
|
STATIC void
|
|
xfs_attr_rmtval_copyin(
|
|
struct xfs_mount *mp,
|
|
struct xfs_buf *bp,
|
|
xfs_ino_t ino,
|
|
int *offset,
|
|
int *valuelen,
|
|
uint8_t **src)
|
|
{
|
|
char *dst = bp->b_addr;
|
|
xfs_daddr_t bno = bp->b_bn;
|
|
int len = BBTOB(bp->b_length);
|
|
int blksize = mp->m_attr_geo->blksize;
|
|
|
|
ASSERT(len >= blksize);
|
|
|
|
while (len > 0 && *valuelen > 0) {
|
|
int hdr_size;
|
|
int byte_cnt = XFS_ATTR3_RMT_BUF_SPACE(mp, blksize);
|
|
|
|
byte_cnt = min(*valuelen, byte_cnt);
|
|
hdr_size = xfs_attr3_rmt_hdr_set(mp, dst, ino, *offset,
|
|
byte_cnt, bno);
|
|
|
|
memcpy(dst + hdr_size, *src, byte_cnt);
|
|
|
|
/*
|
|
* If this is the last block, zero the remainder of it.
|
|
* Check that we are actually the last block, too.
|
|
*/
|
|
if (byte_cnt + hdr_size < blksize) {
|
|
ASSERT(*valuelen - byte_cnt == 0);
|
|
ASSERT(len == blksize);
|
|
memset(dst + hdr_size + byte_cnt, 0,
|
|
blksize - hdr_size - byte_cnt);
|
|
}
|
|
|
|
/* roll buffer forwards */
|
|
len -= blksize;
|
|
dst += blksize;
|
|
bno += BTOBB(blksize);
|
|
|
|
/* roll attribute data forwards */
|
|
*valuelen -= byte_cnt;
|
|
*src += byte_cnt;
|
|
*offset += byte_cnt;
|
|
}
|
|
}
|
|
|
|
/*
|
|
* Read the value associated with an attribute from the out-of-line buffer
|
|
* that we stored it in.
|
|
*/
|
|
int
|
|
xfs_attr_rmtval_get(
|
|
struct xfs_da_args *args)
|
|
{
|
|
struct xfs_bmbt_irec map[ATTR_RMTVALUE_MAPSIZE];
|
|
struct xfs_mount *mp = args->dp->i_mount;
|
|
struct xfs_buf *bp;
|
|
xfs_dablk_t lblkno = args->rmtblkno;
|
|
uint8_t *dst = args->value;
|
|
int valuelen;
|
|
int nmap;
|
|
int error;
|
|
int blkcnt = args->rmtblkcnt;
|
|
int i;
|
|
int offset = 0;
|
|
|
|
trace_xfs_attr_rmtval_get(args);
|
|
|
|
ASSERT(!(args->flags & ATTR_KERNOVAL));
|
|
ASSERT(args->rmtvaluelen == args->valuelen);
|
|
|
|
valuelen = args->rmtvaluelen;
|
|
while (valuelen > 0) {
|
|
nmap = ATTR_RMTVALUE_MAPSIZE;
|
|
error = xfs_bmapi_read(args->dp, (xfs_fileoff_t)lblkno,
|
|
blkcnt, map, &nmap,
|
|
XFS_BMAPI_ATTRFORK);
|
|
if (error)
|
|
return error;
|
|
ASSERT(nmap >= 1);
|
|
|
|
for (i = 0; (i < nmap) && (valuelen > 0); i++) {
|
|
xfs_daddr_t dblkno;
|
|
int dblkcnt;
|
|
|
|
ASSERT((map[i].br_startblock != DELAYSTARTBLOCK) &&
|
|
(map[i].br_startblock != HOLESTARTBLOCK));
|
|
dblkno = XFS_FSB_TO_DADDR(mp, map[i].br_startblock);
|
|
dblkcnt = XFS_FSB_TO_BB(mp, map[i].br_blockcount);
|
|
error = xfs_trans_read_buf(mp, args->trans,
|
|
mp->m_ddev_targp,
|
|
dblkno, dblkcnt, 0, &bp,
|
|
&xfs_attr3_rmt_buf_ops);
|
|
if (error)
|
|
return error;
|
|
|
|
error = xfs_attr_rmtval_copyout(mp, bp, args->dp->i_ino,
|
|
&offset, &valuelen,
|
|
&dst);
|
|
xfs_trans_brelse(args->trans, bp);
|
|
if (error)
|
|
return error;
|
|
|
|
/* roll attribute extent map forwards */
|
|
lblkno += map[i].br_blockcount;
|
|
blkcnt -= map[i].br_blockcount;
|
|
}
|
|
}
|
|
ASSERT(valuelen == 0);
|
|
return 0;
|
|
}
|
|
|
|
/*
|
|
* Write the value associated with an attribute into the out-of-line buffer
|
|
* that we have defined for it.
|
|
*/
|
|
int
|
|
xfs_attr_rmtval_set(
|
|
struct xfs_da_args *args)
|
|
{
|
|
struct xfs_inode *dp = args->dp;
|
|
struct xfs_mount *mp = dp->i_mount;
|
|
struct xfs_bmbt_irec map;
|
|
xfs_dablk_t lblkno;
|
|
xfs_fileoff_t lfileoff = 0;
|
|
uint8_t *src = args->value;
|
|
int blkcnt;
|
|
int valuelen;
|
|
int nmap;
|
|
int error;
|
|
int offset = 0;
|
|
|
|
trace_xfs_attr_rmtval_set(args);
|
|
|
|
/*
|
|
* Find a "hole" in the attribute address space large enough for
|
|
* us to drop the new attribute's value into. Because CRC enable
|
|
* attributes have headers, we can't just do a straight byte to FSB
|
|
* conversion and have to take the header space into account.
|
|
*/
|
|
blkcnt = xfs_attr3_rmt_blocks(mp, args->rmtvaluelen);
|
|
error = xfs_bmap_first_unused(args->trans, args->dp, blkcnt, &lfileoff,
|
|
XFS_ATTR_FORK);
|
|
if (error)
|
|
return error;
|
|
|
|
args->rmtblkno = lblkno = (xfs_dablk_t)lfileoff;
|
|
args->rmtblkcnt = blkcnt;
|
|
|
|
/*
|
|
* Roll through the "value", allocating blocks on disk as required.
|
|
*/
|
|
while (blkcnt > 0) {
|
|
/*
|
|
* Allocate a single extent, up to the size of the value.
|
|
*
|
|
* Note that we have to consider this a data allocation as we
|
|
* write the remote attribute without logging the contents.
|
|
* Hence we must ensure that we aren't using blocks that are on
|
|
* the busy list so that we don't overwrite blocks which have
|
|
* recently been freed but their transactions are not yet
|
|
* committed to disk. If we overwrite the contents of a busy
|
|
* extent and then crash then the block may not contain the
|
|
* correct metadata after log recovery occurs.
|
|
*/
|
|
xfs_defer_init(args->dfops, args->firstblock);
|
|
nmap = 1;
|
|
error = xfs_bmapi_write(args->trans, dp, (xfs_fileoff_t)lblkno,
|
|
blkcnt, XFS_BMAPI_ATTRFORK, args->firstblock,
|
|
args->total, &map, &nmap, args->dfops);
|
|
if (error)
|
|
goto out_defer_cancel;
|
|
xfs_defer_ijoin(args->dfops, dp);
|
|
error = xfs_defer_finish(&args->trans, args->dfops);
|
|
if (error)
|
|
goto out_defer_cancel;
|
|
|
|
ASSERT(nmap == 1);
|
|
ASSERT((map.br_startblock != DELAYSTARTBLOCK) &&
|
|
(map.br_startblock != HOLESTARTBLOCK));
|
|
lblkno += map.br_blockcount;
|
|
blkcnt -= map.br_blockcount;
|
|
|
|
/*
|
|
* Start the next trans in the chain.
|
|
*/
|
|
error = xfs_trans_roll_inode(&args->trans, dp);
|
|
if (error)
|
|
return error;
|
|
}
|
|
|
|
/*
|
|
* Roll through the "value", copying the attribute value to the
|
|
* already-allocated blocks. Blocks are written synchronously
|
|
* so that we can know they are all on disk before we turn off
|
|
* the INCOMPLETE flag.
|
|
*/
|
|
lblkno = args->rmtblkno;
|
|
blkcnt = args->rmtblkcnt;
|
|
valuelen = args->rmtvaluelen;
|
|
while (valuelen > 0) {
|
|
struct xfs_buf *bp;
|
|
xfs_daddr_t dblkno;
|
|
int dblkcnt;
|
|
|
|
ASSERT(blkcnt > 0);
|
|
|
|
xfs_defer_init(args->dfops, args->firstblock);
|
|
nmap = 1;
|
|
error = xfs_bmapi_read(dp, (xfs_fileoff_t)lblkno,
|
|
blkcnt, &map, &nmap,
|
|
XFS_BMAPI_ATTRFORK);
|
|
if (error)
|
|
return error;
|
|
ASSERT(nmap == 1);
|
|
ASSERT((map.br_startblock != DELAYSTARTBLOCK) &&
|
|
(map.br_startblock != HOLESTARTBLOCK));
|
|
|
|
dblkno = XFS_FSB_TO_DADDR(mp, map.br_startblock),
|
|
dblkcnt = XFS_FSB_TO_BB(mp, map.br_blockcount);
|
|
|
|
bp = xfs_buf_get(mp->m_ddev_targp, dblkno, dblkcnt, 0);
|
|
if (!bp)
|
|
return -ENOMEM;
|
|
bp->b_ops = &xfs_attr3_rmt_buf_ops;
|
|
|
|
xfs_attr_rmtval_copyin(mp, bp, args->dp->i_ino, &offset,
|
|
&valuelen, &src);
|
|
|
|
error = xfs_bwrite(bp); /* GROT: NOTE: synchronous write */
|
|
xfs_buf_relse(bp);
|
|
if (error)
|
|
return error;
|
|
|
|
|
|
/* roll attribute extent map forwards */
|
|
lblkno += map.br_blockcount;
|
|
blkcnt -= map.br_blockcount;
|
|
}
|
|
ASSERT(valuelen == 0);
|
|
return 0;
|
|
out_defer_cancel:
|
|
xfs_defer_cancel(args->dfops);
|
|
args->trans = NULL;
|
|
return error;
|
|
}
|
|
|
|
/*
|
|
* Remove the value associated with an attribute by deleting the
|
|
* out-of-line buffer that it is stored on.
|
|
*/
|
|
int
|
|
xfs_attr_rmtval_remove(
|
|
struct xfs_da_args *args)
|
|
{
|
|
struct xfs_mount *mp = args->dp->i_mount;
|
|
xfs_dablk_t lblkno;
|
|
int blkcnt;
|
|
int error;
|
|
int done;
|
|
|
|
trace_xfs_attr_rmtval_remove(args);
|
|
|
|
/*
|
|
* Roll through the "value", invalidating the attribute value's blocks.
|
|
*/
|
|
lblkno = args->rmtblkno;
|
|
blkcnt = args->rmtblkcnt;
|
|
while (blkcnt > 0) {
|
|
struct xfs_bmbt_irec map;
|
|
struct xfs_buf *bp;
|
|
xfs_daddr_t dblkno;
|
|
int dblkcnt;
|
|
int nmap;
|
|
|
|
/*
|
|
* Try to remember where we decided to put the value.
|
|
*/
|
|
nmap = 1;
|
|
error = xfs_bmapi_read(args->dp, (xfs_fileoff_t)lblkno,
|
|
blkcnt, &map, &nmap, XFS_BMAPI_ATTRFORK);
|
|
if (error)
|
|
return error;
|
|
ASSERT(nmap == 1);
|
|
ASSERT((map.br_startblock != DELAYSTARTBLOCK) &&
|
|
(map.br_startblock != HOLESTARTBLOCK));
|
|
|
|
dblkno = XFS_FSB_TO_DADDR(mp, map.br_startblock),
|
|
dblkcnt = XFS_FSB_TO_BB(mp, map.br_blockcount);
|
|
|
|
/*
|
|
* If the "remote" value is in the cache, remove it.
|
|
*/
|
|
bp = xfs_buf_incore(mp->m_ddev_targp, dblkno, dblkcnt, XBF_TRYLOCK);
|
|
if (bp) {
|
|
xfs_buf_stale(bp);
|
|
xfs_buf_relse(bp);
|
|
bp = NULL;
|
|
}
|
|
|
|
lblkno += map.br_blockcount;
|
|
blkcnt -= map.br_blockcount;
|
|
}
|
|
|
|
/*
|
|
* Keep de-allocating extents until the remote-value region is gone.
|
|
*/
|
|
lblkno = args->rmtblkno;
|
|
blkcnt = args->rmtblkcnt;
|
|
done = 0;
|
|
while (!done) {
|
|
xfs_defer_init(args->dfops, args->firstblock);
|
|
error = xfs_bunmapi(args->trans, args->dp, lblkno, blkcnt,
|
|
XFS_BMAPI_ATTRFORK, 1, args->firstblock,
|
|
args->dfops, &done);
|
|
if (error)
|
|
goto out_defer_cancel;
|
|
xfs_defer_ijoin(args->dfops, args->dp);
|
|
error = xfs_defer_finish(&args->trans, args->dfops);
|
|
if (error)
|
|
goto out_defer_cancel;
|
|
|
|
/*
|
|
* Close out trans and start the next one in the chain.
|
|
*/
|
|
error = xfs_trans_roll_inode(&args->trans, args->dp);
|
|
if (error)
|
|
return error;
|
|
}
|
|
return 0;
|
|
out_defer_cancel:
|
|
xfs_defer_cancel(args->dfops);
|
|
args->trans = NULL;
|
|
return error;
|
|
}
|