mirror of
https://github.com/brain-hackers/linux-brain.git
synced 2024-06-09 07:16:21 +09:00
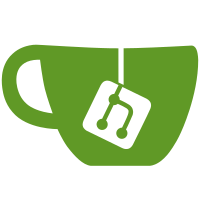
zstd_init(, comp_level = 0) initializes decompression part of API only hat now consists of zstd_decompress_stream() function. The perf.data PERF_RECORD_COMPRESSED records are decompressed using zstd_decompress_stream() function into a linked list of mmaped memory regions of mmap_comp_len size (struct decomp). After decompression of one COMPRESSED record its content is iterated and fetched for usual processing. The mmaped memory regions with decompressed events are kept in the linked list till the tool process termination. When dumping raw records (e.g., perf report -D --header) file offsets of events from compressed records are printed as zero. Committer notes: Since now we have support for processing PERF_RECORD_COMPRESSED, we see none, in raw form, like we saw in the previous patch commiter notes, they were decompressed into the usual PERF_RECORD_{FORK,MMAP,COMM,etc} records, we only see the stats for those PERF_RECORD_COMPRESSED events, and since I used the file generated in the commiter notes for the previous patch, there they are, 2 compressed records: $ perf report --header-only | grep cmdline # cmdline : /home/acme/bin/perf record -z2 sleep 1 $ perf report -D | grep COMPRESS COMPRESSED events: 2 COMPRESSED events: 0 $ perf report --stdio # To display the perf.data header info, please use --header/--header-only options. # # # Total Lost Samples: 0 # # Samples: 15 of event 'cycles:u' # Event count (approx.): 962227 # # Overhead Command Shared Object Symbol # ........ ....... ................ ........................... # 46.99% sleep libc-2.28.so [.] _dl_addr 29.24% sleep [unknown] [k] 0xffffffffaea00a67 16.45% sleep libc-2.28.so [.] __GI__IO_un_link.part.1 5.92% sleep ld-2.28.so [.] _dl_setup_hash 1.40% sleep libc-2.28.so [.] __nanosleep 0.00% sleep [unknown] [k] 0xffffffffaea00163 # # (Tip: To see callchains in a more compact form: perf report -g folded) # $ Signed-off-by: Alexey Budankov <alexey.budankov@linux.intel.com> Reviewed-by: Jiri Olsa <jolsa@kernel.org> Tested-by: Arnaldo Carvalho de Melo <acme@redhat.com> Cc: Alexander Shishkin <alexander.shishkin@linux.intel.com> Cc: Andi Kleen <ak@linux.intel.com> Cc: Namhyung Kim <namhyung@kernel.org> Cc: Peter Zijlstra <peterz@infradead.org> Link: http://lkml.kernel.org/r/304b0a59-942c-3fe1-da02-aa749f87108b@linux.intel.com Signed-off-by: Arnaldo Carvalho de Melo <acme@redhat.com>
69 lines
1.8 KiB
C
69 lines
1.8 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
#ifndef PERF_COMPRESS_H
|
|
#define PERF_COMPRESS_H
|
|
|
|
#include <stdbool.h>
|
|
#ifdef HAVE_ZSTD_SUPPORT
|
|
#include <zstd.h>
|
|
#endif
|
|
|
|
#ifdef HAVE_ZLIB_SUPPORT
|
|
int gzip_decompress_to_file(const char *input, int output_fd);
|
|
bool gzip_is_compressed(const char *input);
|
|
#endif
|
|
|
|
#ifdef HAVE_LZMA_SUPPORT
|
|
int lzma_decompress_to_file(const char *input, int output_fd);
|
|
bool lzma_is_compressed(const char *input);
|
|
#endif
|
|
|
|
struct zstd_data {
|
|
#ifdef HAVE_ZSTD_SUPPORT
|
|
ZSTD_CStream *cstream;
|
|
ZSTD_DStream *dstream;
|
|
#endif
|
|
};
|
|
|
|
#ifdef HAVE_ZSTD_SUPPORT
|
|
|
|
int zstd_init(struct zstd_data *data, int level);
|
|
int zstd_fini(struct zstd_data *data);
|
|
|
|
size_t zstd_compress_stream_to_records(struct zstd_data *data, void *dst, size_t dst_size,
|
|
void *src, size_t src_size, size_t max_record_size,
|
|
size_t process_header(void *record, size_t increment));
|
|
|
|
size_t zstd_decompress_stream(struct zstd_data *data, void *src, size_t src_size,
|
|
void *dst, size_t dst_size);
|
|
#else /* !HAVE_ZSTD_SUPPORT */
|
|
|
|
static inline int zstd_init(struct zstd_data *data __maybe_unused, int level __maybe_unused)
|
|
{
|
|
return 0;
|
|
}
|
|
|
|
static inline int zstd_fini(struct zstd_data *data __maybe_unused)
|
|
{
|
|
return 0;
|
|
}
|
|
|
|
static inline
|
|
size_t zstd_compress_stream_to_records(struct zstd_data *data __maybe_unused,
|
|
void *dst __maybe_unused, size_t dst_size __maybe_unused,
|
|
void *src __maybe_unused, size_t src_size __maybe_unused,
|
|
size_t max_record_size __maybe_unused,
|
|
size_t process_header(void *record, size_t increment) __maybe_unused)
|
|
{
|
|
return 0;
|
|
}
|
|
|
|
static inline size_t zstd_decompress_stream(struct zstd_data *data __maybe_unused, void *src __maybe_unused,
|
|
size_t src_size __maybe_unused, void *dst __maybe_unused,
|
|
size_t dst_size __maybe_unused)
|
|
{
|
|
return 0;
|
|
}
|
|
#endif
|
|
|
|
#endif /* PERF_COMPRESS_H */
|