mirror of
https://github.com/brain-hackers/linux-brain.git
synced 2024-06-09 15:26:21 +09:00
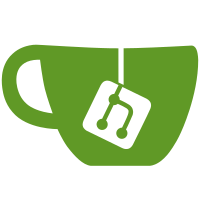
commite562a5f13c
("ASoC: sirf: sirf-audio: don't select unnecessary Platform") Current ALSA SoC avoid to add duplicate component to rtd, and this driver was selecting CPU component as Platform component. Thus, above patch removed Platform settings from this driver, because it assumed these are same component. But, some CPU driver is using generic DMAEngine, in such case, both CPU component and Platform component will have same of_node/name. In other words, there are some components which are different but have same of_node/name. In such case, Card driver definitely need to select Platform even though it is same as CPU. It is depends on CPU driver, but is difficult to know it from Card driver. This patch reverts above patch. Fixes: commite562a5f13c
("ASoC: sirf: sirf-audio: don't select unnecessary Platform") Signed-off-by: Kuninori Morimoto <kuninori.morimoto.gx@renesas.com> Signed-off-by: Mark Brown <broonie@kernel.org>
161 lines
4.6 KiB
C
161 lines
4.6 KiB
C
// SPDX-License-Identifier: GPL-2.0-or-later
|
|
/*
|
|
* SiRF audio card driver
|
|
*
|
|
* Copyright (c) 2011 Cambridge Silicon Radio Limited, a CSR plc group company.
|
|
*/
|
|
|
|
#include <linux/platform_device.h>
|
|
#include <linux/module.h>
|
|
#include <linux/of.h>
|
|
#include <linux/gpio.h>
|
|
#include <linux/of_gpio.h>
|
|
#include <sound/core.h>
|
|
#include <sound/pcm.h>
|
|
#include <sound/soc.h>
|
|
|
|
struct sirf_audio_card {
|
|
unsigned int gpio_hp_pa;
|
|
unsigned int gpio_spk_pa;
|
|
};
|
|
|
|
static int sirf_audio_hp_event(struct snd_soc_dapm_widget *w,
|
|
struct snd_kcontrol *ctrl, int event)
|
|
{
|
|
struct snd_soc_dapm_context *dapm = w->dapm;
|
|
struct snd_soc_card *card = dapm->card;
|
|
struct sirf_audio_card *sirf_audio_card = snd_soc_card_get_drvdata(card);
|
|
int on = !SND_SOC_DAPM_EVENT_OFF(event);
|
|
|
|
if (gpio_is_valid(sirf_audio_card->gpio_hp_pa))
|
|
gpio_set_value(sirf_audio_card->gpio_hp_pa, on);
|
|
return 0;
|
|
}
|
|
|
|
static int sirf_audio_spk_event(struct snd_soc_dapm_widget *w,
|
|
struct snd_kcontrol *ctrl, int event)
|
|
{
|
|
struct snd_soc_dapm_context *dapm = w->dapm;
|
|
struct snd_soc_card *card = dapm->card;
|
|
struct sirf_audio_card *sirf_audio_card = snd_soc_card_get_drvdata(card);
|
|
int on = !SND_SOC_DAPM_EVENT_OFF(event);
|
|
|
|
if (gpio_is_valid(sirf_audio_card->gpio_spk_pa))
|
|
gpio_set_value(sirf_audio_card->gpio_spk_pa, on);
|
|
|
|
return 0;
|
|
}
|
|
static const struct snd_soc_dapm_widget sirf_audio_dapm_widgets[] = {
|
|
SND_SOC_DAPM_HP("Hp", sirf_audio_hp_event),
|
|
SND_SOC_DAPM_SPK("Ext Spk", sirf_audio_spk_event),
|
|
SND_SOC_DAPM_MIC("Ext Mic", NULL),
|
|
};
|
|
|
|
static const struct snd_soc_dapm_route intercon[] = {
|
|
{"Hp", NULL, "HPOUTL"},
|
|
{"Hp", NULL, "HPOUTR"},
|
|
{"Ext Spk", NULL, "SPKOUT"},
|
|
{"MICIN1", NULL, "Mic Bias"},
|
|
{"Mic Bias", NULL, "Ext Mic"},
|
|
};
|
|
|
|
/* Digital audio interface glue - connects codec <--> CPU */
|
|
SND_SOC_DAILINK_DEFS(sirf,
|
|
DAILINK_COMP_ARRAY(COMP_EMPTY()),
|
|
DAILINK_COMP_ARRAY(COMP_CODEC(NULL, "sirf-audio-codec")),
|
|
DAILINK_COMP_ARRAY(COMP_EMPTY()));
|
|
|
|
static struct snd_soc_dai_link sirf_audio_dai_link[] = {
|
|
{
|
|
.name = "SiRF audio card",
|
|
.stream_name = "SiRF audio HiFi",
|
|
SND_SOC_DAILINK_REG(sirf),
|
|
},
|
|
};
|
|
|
|
/* Audio machine driver */
|
|
static struct snd_soc_card snd_soc_sirf_audio_card = {
|
|
.name = "SiRF audio card",
|
|
.owner = THIS_MODULE,
|
|
.dai_link = sirf_audio_dai_link,
|
|
.num_links = ARRAY_SIZE(sirf_audio_dai_link),
|
|
.dapm_widgets = sirf_audio_dapm_widgets,
|
|
.num_dapm_widgets = ARRAY_SIZE(sirf_audio_dapm_widgets),
|
|
.dapm_routes = intercon,
|
|
.num_dapm_routes = ARRAY_SIZE(intercon),
|
|
};
|
|
|
|
static int sirf_audio_probe(struct platform_device *pdev)
|
|
{
|
|
struct snd_soc_card *card = &snd_soc_sirf_audio_card;
|
|
struct sirf_audio_card *sirf_audio_card;
|
|
int ret;
|
|
|
|
sirf_audio_card = devm_kzalloc(&pdev->dev, sizeof(struct sirf_audio_card),
|
|
GFP_KERNEL);
|
|
if (sirf_audio_card == NULL)
|
|
return -ENOMEM;
|
|
|
|
sirf_audio_dai_link[0].cpus->of_node =
|
|
of_parse_phandle(pdev->dev.of_node, "sirf,audio-platform", 0);
|
|
sirf_audio_dai_link[0].platforms->of_node =
|
|
of_parse_phandle(pdev->dev.of_node, "sirf,audio-platform", 0);
|
|
sirf_audio_dai_link[0].codecs->of_node =
|
|
of_parse_phandle(pdev->dev.of_node, "sirf,audio-codec", 0);
|
|
sirf_audio_card->gpio_spk_pa = of_get_named_gpio(pdev->dev.of_node,
|
|
"spk-pa-gpios", 0);
|
|
sirf_audio_card->gpio_hp_pa = of_get_named_gpio(pdev->dev.of_node,
|
|
"hp-pa-gpios", 0);
|
|
if (gpio_is_valid(sirf_audio_card->gpio_spk_pa)) {
|
|
ret = devm_gpio_request_one(&pdev->dev,
|
|
sirf_audio_card->gpio_spk_pa,
|
|
GPIOF_OUT_INIT_LOW, "SPA_PA_SD");
|
|
if (ret) {
|
|
dev_err(&pdev->dev,
|
|
"Failed to request GPIO_%d for reset: %d\n",
|
|
sirf_audio_card->gpio_spk_pa, ret);
|
|
return ret;
|
|
}
|
|
}
|
|
if (gpio_is_valid(sirf_audio_card->gpio_hp_pa)) {
|
|
ret = devm_gpio_request_one(&pdev->dev,
|
|
sirf_audio_card->gpio_hp_pa,
|
|
GPIOF_OUT_INIT_LOW, "HP_PA_SD");
|
|
if (ret) {
|
|
dev_err(&pdev->dev,
|
|
"Failed to request GPIO_%d for reset: %d\n",
|
|
sirf_audio_card->gpio_hp_pa, ret);
|
|
return ret;
|
|
}
|
|
}
|
|
|
|
card->dev = &pdev->dev;
|
|
snd_soc_card_set_drvdata(card, sirf_audio_card);
|
|
|
|
ret = devm_snd_soc_register_card(&pdev->dev, card);
|
|
if (ret)
|
|
dev_err(&pdev->dev, "snd_soc_register_card() failed:%d\n", ret);
|
|
|
|
return ret;
|
|
}
|
|
|
|
static const struct of_device_id sirf_audio_of_match[] = {
|
|
{.compatible = "sirf,sirf-audio-card", },
|
|
{ },
|
|
};
|
|
MODULE_DEVICE_TABLE(of, sirf_audio_of_match);
|
|
|
|
static struct platform_driver sirf_audio_driver = {
|
|
.driver = {
|
|
.name = "sirf-audio-card",
|
|
.pm = &snd_soc_pm_ops,
|
|
.of_match_table = sirf_audio_of_match,
|
|
},
|
|
.probe = sirf_audio_probe,
|
|
};
|
|
module_platform_driver(sirf_audio_driver);
|
|
|
|
MODULE_AUTHOR("RongJun Ying <RongJun.Ying@csr.com>");
|
|
MODULE_DESCRIPTION("ALSA SoC SIRF audio card driver");
|
|
MODULE_LICENSE("GPL v2");
|