mirror of
https://github.com/brain-hackers/linux-brain.git
synced 2024-06-09 15:26:21 +09:00
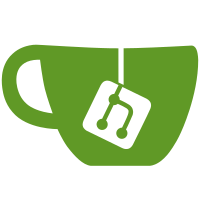
commit 864cee90d4bd870e5d5e5a0b1a6f055f4f951350 upstream.
On TODDR sm1, the fifo threshold register field is slightly different
compared to the other SoCs. This leads to the fifo A being flushed to
memory every 8kB. If the period is smaller than that, several periods
are pushed to memory and notified at once. This is not ideal.
Fix the register field update. With this, the fifos are flushed every
128B. We could still do better, like adapt the threshold depending on
the period size, but at least it consistent across the different
SoC/fifos
Fixes: 5ac825c3d8
("ASoC: meson: axg-toddr: add sm1 support")
Reported-by: Alden DSouza <aldend@google.com>
Signed-off-by: Jerome Brunet <jbrunet@baylibre.com>
Link: https://lore.kernel.org/r/20191218172420.1199117-2-jbrunet@baylibre.com
Signed-off-by: Mark Brown <broonie@kernel.org>
Signed-off-by: Greg Kroah-Hartman <gregkh@linuxfoundation.org>
87 lines
2.3 KiB
C
87 lines
2.3 KiB
C
/* SPDX-License-Identifier: (GPL-2.0 OR MIT) */
|
|
/*
|
|
* Copyright (c) 2018 BayLibre, SAS.
|
|
* Author: Jerome Brunet <jbrunet@baylibre.com>
|
|
*/
|
|
|
|
#ifndef _MESON_AXG_FIFO_H
|
|
#define _MESON_AXG_FIFO_H
|
|
|
|
struct clk;
|
|
struct platform_device;
|
|
struct reg_field;
|
|
struct regmap;
|
|
struct regmap_field;
|
|
struct reset_control;
|
|
|
|
struct snd_soc_component_driver;
|
|
struct snd_soc_dai;
|
|
struct snd_soc_dai_driver;
|
|
struct snd_pcm_ops;
|
|
struct snd_soc_pcm_runtime;
|
|
|
|
#define AXG_FIFO_CH_MAX 128
|
|
#define AXG_FIFO_RATES (SNDRV_PCM_RATE_5512 | \
|
|
SNDRV_PCM_RATE_8000_192000)
|
|
#define AXG_FIFO_FORMATS (SNDRV_PCM_FMTBIT_S8 | \
|
|
SNDRV_PCM_FMTBIT_S16_LE | \
|
|
SNDRV_PCM_FMTBIT_S20_LE | \
|
|
SNDRV_PCM_FMTBIT_S24_LE | \
|
|
SNDRV_PCM_FMTBIT_S32_LE | \
|
|
SNDRV_PCM_FMTBIT_IEC958_SUBFRAME_LE)
|
|
|
|
#define AXG_FIFO_BURST 8
|
|
#define AXG_FIFO_MIN_CNT 64
|
|
#define AXG_FIFO_MIN_DEPTH (AXG_FIFO_BURST * AXG_FIFO_MIN_CNT)
|
|
|
|
#define FIFO_INT_ADDR_FINISH BIT(0)
|
|
#define FIFO_INT_ADDR_INT BIT(1)
|
|
#define FIFO_INT_COUNT_REPEAT BIT(2)
|
|
#define FIFO_INT_COUNT_ONCE BIT(3)
|
|
#define FIFO_INT_FIFO_ZERO BIT(4)
|
|
#define FIFO_INT_FIFO_DEPTH BIT(5)
|
|
#define FIFO_INT_MASK GENMASK(7, 0)
|
|
|
|
#define FIFO_CTRL0 0x00
|
|
#define CTRL0_DMA_EN BIT(31)
|
|
#define CTRL0_INT_EN(x) ((x) << 16)
|
|
#define CTRL0_SEL_MASK GENMASK(2, 0)
|
|
#define CTRL0_SEL_SHIFT 0
|
|
#define FIFO_CTRL1 0x04
|
|
#define CTRL1_INT_CLR(x) ((x) << 0)
|
|
#define CTRL1_STATUS2_SEL_MASK GENMASK(11, 8)
|
|
#define CTRL1_STATUS2_SEL(x) ((x) << 8)
|
|
#define STATUS2_SEL_DDR_READ 0
|
|
#define CTRL1_FRDDR_DEPTH_MASK GENMASK(31, 24)
|
|
#define CTRL1_FRDDR_DEPTH(x) ((x) << 24)
|
|
#define FIFO_START_ADDR 0x08
|
|
#define FIFO_FINISH_ADDR 0x0c
|
|
#define FIFO_INT_ADDR 0x10
|
|
#define FIFO_STATUS1 0x14
|
|
#define STATUS1_INT_STS(x) ((x) << 0)
|
|
#define FIFO_STATUS2 0x18
|
|
#define FIFO_INIT_ADDR 0x24
|
|
#define FIFO_CTRL2 0x28
|
|
|
|
struct axg_fifo {
|
|
struct regmap *map;
|
|
struct clk *pclk;
|
|
struct reset_control *arb;
|
|
struct regmap_field *field_threshold;
|
|
int irq;
|
|
};
|
|
|
|
struct axg_fifo_match_data {
|
|
const struct snd_soc_component_driver *component_drv;
|
|
struct snd_soc_dai_driver *dai_drv;
|
|
struct reg_field field_threshold;
|
|
};
|
|
|
|
extern const struct snd_pcm_ops axg_fifo_pcm_ops;
|
|
extern const struct snd_pcm_ops g12a_fifo_pcm_ops;
|
|
|
|
int axg_fifo_pcm_new(struct snd_soc_pcm_runtime *rtd, unsigned int type);
|
|
int axg_fifo_probe(struct platform_device *pdev);
|
|
|
|
#endif /* _MESON_AXG_FIFO_H */
|