mirror of
https://github.com/brain-hackers/linux-brain.git
synced 2024-06-09 23:36:23 +09:00
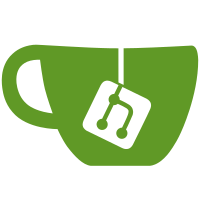
Boris suggests to make a local label (prepend ".L") to these functions to eliminate them from the symbol table. These are functions with very local names and really should not be visible anywhere. Note that objtool won't see these functions anymore (to generate ORC debug info). But all the functions are not annotated with ENDPROC, so they won't have objtool's attention anyway. Signed-off-by: Jiri Slaby <jslaby@suse.cz> Signed-off-by: Borislav Petkov <bp@suse.de> Cc: Andy Lutomirski <luto@kernel.org> Cc: Cao jin <caoj.fnst@cn.fujitsu.com> Cc: Greg Kroah-Hartman <gregkh@linuxfoundation.org> Cc: "H. Peter Anvin" <hpa@zytor.com> Cc: Ingo Molnar <mingo@redhat.com> Cc: Josh Poimboeuf <jpoimboe@redhat.com> Cc: "Kirill A. Shutemov" <kirill.shutemov@linux.intel.com> Cc: Peter Zijlstra <peterz@infradead.org> Cc: Steve Winslow <swinslow@gmail.com> Cc: Thomas Gleixner <tglx@linutronix.de> Cc: Wei Huang <wei@redhat.com> Cc: x86-ml <x86@kernel.org> Cc: Xiaoyao Li <xiaoyao.li@linux.intel.com> Link: https://lkml.kernel.org/r/20190906075550.23435-2-jslaby@suse.cz
107 lines
2.1 KiB
ArmAsm
107 lines
2.1 KiB
ArmAsm
/* SPDX-License-Identifier: GPL-2.0 */
|
|
/*
|
|
* __put_user functions.
|
|
*
|
|
* (C) Copyright 2005 Linus Torvalds
|
|
* (C) Copyright 2005 Andi Kleen
|
|
* (C) Copyright 2008 Glauber Costa
|
|
*
|
|
* These functions have a non-standard call interface
|
|
* to make them more efficient, especially as they
|
|
* return an error value in addition to the "real"
|
|
* return value.
|
|
*/
|
|
#include <linux/linkage.h>
|
|
#include <asm/thread_info.h>
|
|
#include <asm/errno.h>
|
|
#include <asm/asm.h>
|
|
#include <asm/smap.h>
|
|
#include <asm/export.h>
|
|
|
|
|
|
/*
|
|
* __put_user_X
|
|
*
|
|
* Inputs: %eax[:%edx] contains the data
|
|
* %ecx contains the address
|
|
*
|
|
* Outputs: %eax is error code (0 or -EFAULT)
|
|
*
|
|
* These functions should not modify any other registers,
|
|
* as they get called from within inline assembly.
|
|
*/
|
|
|
|
#define ENTER mov PER_CPU_VAR(current_task), %_ASM_BX
|
|
|
|
.text
|
|
ENTRY(__put_user_1)
|
|
ENTER
|
|
cmp TASK_addr_limit(%_ASM_BX),%_ASM_CX
|
|
jae .Lbad_put_user
|
|
ASM_STAC
|
|
1: movb %al,(%_ASM_CX)
|
|
xor %eax,%eax
|
|
ASM_CLAC
|
|
ret
|
|
ENDPROC(__put_user_1)
|
|
EXPORT_SYMBOL(__put_user_1)
|
|
|
|
ENTRY(__put_user_2)
|
|
ENTER
|
|
mov TASK_addr_limit(%_ASM_BX),%_ASM_BX
|
|
sub $1,%_ASM_BX
|
|
cmp %_ASM_BX,%_ASM_CX
|
|
jae .Lbad_put_user
|
|
ASM_STAC
|
|
2: movw %ax,(%_ASM_CX)
|
|
xor %eax,%eax
|
|
ASM_CLAC
|
|
ret
|
|
ENDPROC(__put_user_2)
|
|
EXPORT_SYMBOL(__put_user_2)
|
|
|
|
ENTRY(__put_user_4)
|
|
ENTER
|
|
mov TASK_addr_limit(%_ASM_BX),%_ASM_BX
|
|
sub $3,%_ASM_BX
|
|
cmp %_ASM_BX,%_ASM_CX
|
|
jae .Lbad_put_user
|
|
ASM_STAC
|
|
3: movl %eax,(%_ASM_CX)
|
|
xor %eax,%eax
|
|
ASM_CLAC
|
|
ret
|
|
ENDPROC(__put_user_4)
|
|
EXPORT_SYMBOL(__put_user_4)
|
|
|
|
ENTRY(__put_user_8)
|
|
ENTER
|
|
mov TASK_addr_limit(%_ASM_BX),%_ASM_BX
|
|
sub $7,%_ASM_BX
|
|
cmp %_ASM_BX,%_ASM_CX
|
|
jae .Lbad_put_user
|
|
ASM_STAC
|
|
4: mov %_ASM_AX,(%_ASM_CX)
|
|
#ifdef CONFIG_X86_32
|
|
5: movl %edx,4(%_ASM_CX)
|
|
#endif
|
|
xor %eax,%eax
|
|
ASM_CLAC
|
|
RET
|
|
ENDPROC(__put_user_8)
|
|
EXPORT_SYMBOL(__put_user_8)
|
|
|
|
.Lbad_put_user_clac:
|
|
ASM_CLAC
|
|
.Lbad_put_user:
|
|
movl $-EFAULT,%eax
|
|
RET
|
|
|
|
_ASM_EXTABLE_UA(1b, .Lbad_put_user_clac)
|
|
_ASM_EXTABLE_UA(2b, .Lbad_put_user_clac)
|
|
_ASM_EXTABLE_UA(3b, .Lbad_put_user_clac)
|
|
_ASM_EXTABLE_UA(4b, .Lbad_put_user_clac)
|
|
#ifdef CONFIG_X86_32
|
|
_ASM_EXTABLE_UA(5b, .Lbad_put_user_clac)
|
|
#endif
|