mirror of
https://github.com/brain-hackers/linux-brain.git
synced 2024-06-09 23:36:23 +09:00
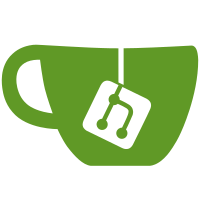
Boris suggests to make a local label (prepend ".L") to these functions to eliminate them from the symbol table. These are functions with very local names and really should not be visible anywhere. Note that objtool won't see these functions anymore (to generate ORC debug info). But all the functions are not annotated with ENDPROC, so they won't have objtool's attention anyway. Signed-off-by: Jiri Slaby <jslaby@suse.cz> Signed-off-by: Borislav Petkov <bp@suse.de> Cc: Andy Lutomirski <luto@kernel.org> Cc: Cao jin <caoj.fnst@cn.fujitsu.com> Cc: Greg Kroah-Hartman <gregkh@linuxfoundation.org> Cc: "H. Peter Anvin" <hpa@zytor.com> Cc: Ingo Molnar <mingo@redhat.com> Cc: Josh Poimboeuf <jpoimboe@redhat.com> Cc: "Kirill A. Shutemov" <kirill.shutemov@linux.intel.com> Cc: Peter Zijlstra <peterz@infradead.org> Cc: Steve Winslow <swinslow@gmail.com> Cc: Thomas Gleixner <tglx@linutronix.de> Cc: Wei Huang <wei@redhat.com> Cc: x86-ml <x86@kernel.org> Cc: Xiaoyao Li <xiaoyao.li@linux.intel.com> Link: https://lkml.kernel.org/r/20190906075550.23435-2-jslaby@suse.cz
144 lines
3.1 KiB
ArmAsm
144 lines
3.1 KiB
ArmAsm
/* SPDX-License-Identifier: GPL-2.0 */
|
|
/*
|
|
* __get_user functions.
|
|
*
|
|
* (C) Copyright 1998 Linus Torvalds
|
|
* (C) Copyright 2005 Andi Kleen
|
|
* (C) Copyright 2008 Glauber Costa
|
|
*
|
|
* These functions have a non-standard call interface
|
|
* to make them more efficient, especially as they
|
|
* return an error value in addition to the "real"
|
|
* return value.
|
|
*/
|
|
|
|
/*
|
|
* __get_user_X
|
|
*
|
|
* Inputs: %[r|e]ax contains the address.
|
|
*
|
|
* Outputs: %[r|e]ax is error code (0 or -EFAULT)
|
|
* %[r|e]dx contains zero-extended value
|
|
* %ecx contains the high half for 32-bit __get_user_8
|
|
*
|
|
*
|
|
* These functions should not modify any other registers,
|
|
* as they get called from within inline assembly.
|
|
*/
|
|
|
|
#include <linux/linkage.h>
|
|
#include <asm/page_types.h>
|
|
#include <asm/errno.h>
|
|
#include <asm/asm-offsets.h>
|
|
#include <asm/thread_info.h>
|
|
#include <asm/asm.h>
|
|
#include <asm/smap.h>
|
|
#include <asm/export.h>
|
|
|
|
.text
|
|
ENTRY(__get_user_1)
|
|
mov PER_CPU_VAR(current_task), %_ASM_DX
|
|
cmp TASK_addr_limit(%_ASM_DX),%_ASM_AX
|
|
jae bad_get_user
|
|
sbb %_ASM_DX, %_ASM_DX /* array_index_mask_nospec() */
|
|
and %_ASM_DX, %_ASM_AX
|
|
ASM_STAC
|
|
1: movzbl (%_ASM_AX),%edx
|
|
xor %eax,%eax
|
|
ASM_CLAC
|
|
ret
|
|
ENDPROC(__get_user_1)
|
|
EXPORT_SYMBOL(__get_user_1)
|
|
|
|
ENTRY(__get_user_2)
|
|
add $1,%_ASM_AX
|
|
jc bad_get_user
|
|
mov PER_CPU_VAR(current_task), %_ASM_DX
|
|
cmp TASK_addr_limit(%_ASM_DX),%_ASM_AX
|
|
jae bad_get_user
|
|
sbb %_ASM_DX, %_ASM_DX /* array_index_mask_nospec() */
|
|
and %_ASM_DX, %_ASM_AX
|
|
ASM_STAC
|
|
2: movzwl -1(%_ASM_AX),%edx
|
|
xor %eax,%eax
|
|
ASM_CLAC
|
|
ret
|
|
ENDPROC(__get_user_2)
|
|
EXPORT_SYMBOL(__get_user_2)
|
|
|
|
ENTRY(__get_user_4)
|
|
add $3,%_ASM_AX
|
|
jc bad_get_user
|
|
mov PER_CPU_VAR(current_task), %_ASM_DX
|
|
cmp TASK_addr_limit(%_ASM_DX),%_ASM_AX
|
|
jae bad_get_user
|
|
sbb %_ASM_DX, %_ASM_DX /* array_index_mask_nospec() */
|
|
and %_ASM_DX, %_ASM_AX
|
|
ASM_STAC
|
|
3: movl -3(%_ASM_AX),%edx
|
|
xor %eax,%eax
|
|
ASM_CLAC
|
|
ret
|
|
ENDPROC(__get_user_4)
|
|
EXPORT_SYMBOL(__get_user_4)
|
|
|
|
ENTRY(__get_user_8)
|
|
#ifdef CONFIG_X86_64
|
|
add $7,%_ASM_AX
|
|
jc bad_get_user
|
|
mov PER_CPU_VAR(current_task), %_ASM_DX
|
|
cmp TASK_addr_limit(%_ASM_DX),%_ASM_AX
|
|
jae bad_get_user
|
|
sbb %_ASM_DX, %_ASM_DX /* array_index_mask_nospec() */
|
|
and %_ASM_DX, %_ASM_AX
|
|
ASM_STAC
|
|
4: movq -7(%_ASM_AX),%rdx
|
|
xor %eax,%eax
|
|
ASM_CLAC
|
|
ret
|
|
#else
|
|
add $7,%_ASM_AX
|
|
jc bad_get_user_8
|
|
mov PER_CPU_VAR(current_task), %_ASM_DX
|
|
cmp TASK_addr_limit(%_ASM_DX),%_ASM_AX
|
|
jae bad_get_user_8
|
|
sbb %_ASM_DX, %_ASM_DX /* array_index_mask_nospec() */
|
|
and %_ASM_DX, %_ASM_AX
|
|
ASM_STAC
|
|
4: movl -7(%_ASM_AX),%edx
|
|
5: movl -3(%_ASM_AX),%ecx
|
|
xor %eax,%eax
|
|
ASM_CLAC
|
|
ret
|
|
#endif
|
|
ENDPROC(__get_user_8)
|
|
EXPORT_SYMBOL(__get_user_8)
|
|
|
|
|
|
.Lbad_get_user_clac:
|
|
ASM_CLAC
|
|
bad_get_user:
|
|
xor %edx,%edx
|
|
mov $(-EFAULT),%_ASM_AX
|
|
ret
|
|
|
|
#ifdef CONFIG_X86_32
|
|
.Lbad_get_user_8_clac:
|
|
ASM_CLAC
|
|
bad_get_user_8:
|
|
xor %edx,%edx
|
|
xor %ecx,%ecx
|
|
mov $(-EFAULT),%_ASM_AX
|
|
ret
|
|
#endif
|
|
|
|
_ASM_EXTABLE_UA(1b, .Lbad_get_user_clac)
|
|
_ASM_EXTABLE_UA(2b, .Lbad_get_user_clac)
|
|
_ASM_EXTABLE_UA(3b, .Lbad_get_user_clac)
|
|
#ifdef CONFIG_X86_64
|
|
_ASM_EXTABLE_UA(4b, .Lbad_get_user_clac)
|
|
#else
|
|
_ASM_EXTABLE_UA(4b, .Lbad_get_user_8_clac)
|
|
_ASM_EXTABLE_UA(5b, .Lbad_get_user_8_clac)
|
|
#endif
|