mirror of
https://github.com/brain-hackers/linux-brain.git
synced 2024-06-09 15:26:21 +09:00
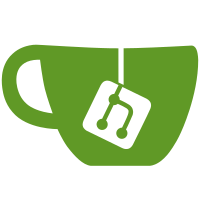
Some platforms execute their timer handler with the interrupt priority level set below 6. That means the handler could be interrupted by another driver and this could lead to re-entry of the timer core. Avoid this by use of local_irq_save/restore for timer interrupt dispatch. This provides mutual exclusion around the timer interrupt flag access which is needed later in this series for the clocksource conversion. Reported-by: Thomas Gleixner <tglx@linutronix.de> Link: http://lkml.kernel.org/r/alpine.DEB.2.21.1811131407120.2697@nanos.tec.linutronix.de Signed-off-by: Finn Thain <fthain@telegraphics.com.au> Signed-off-by: Geert Uytterhoeven <geert@linux-m68k.org>
103 lines
2.2 KiB
C
103 lines
2.2 KiB
C
/*
|
|
* linux/arch/m68k/sun3/sun3ints.c -- Sun-3(x) Linux interrupt handling code
|
|
*
|
|
* This file is subject to the terms and conditions of the GNU General Public
|
|
* License. See the file COPYING in the main directory of this archive
|
|
* for more details.
|
|
*/
|
|
|
|
#include <linux/types.h>
|
|
#include <linux/kernel.h>
|
|
#include <linux/sched.h>
|
|
#include <linux/kernel_stat.h>
|
|
#include <linux/interrupt.h>
|
|
#include <asm/segment.h>
|
|
#include <asm/intersil.h>
|
|
#include <asm/oplib.h>
|
|
#include <asm/sun3ints.h>
|
|
#include <asm/irq_regs.h>
|
|
#include <linux/seq_file.h>
|
|
|
|
extern void sun3_leds (unsigned char);
|
|
|
|
void sun3_disable_interrupts(void)
|
|
{
|
|
sun3_disable_irq(0);
|
|
}
|
|
|
|
void sun3_enable_interrupts(void)
|
|
{
|
|
sun3_enable_irq(0);
|
|
}
|
|
|
|
static int led_pattern[8] = {
|
|
~(0x80), ~(0x01),
|
|
~(0x40), ~(0x02),
|
|
~(0x20), ~(0x04),
|
|
~(0x10), ~(0x08)
|
|
};
|
|
|
|
volatile unsigned char* sun3_intreg;
|
|
|
|
void sun3_enable_irq(unsigned int irq)
|
|
{
|
|
*sun3_intreg |= (1 << irq);
|
|
}
|
|
|
|
void sun3_disable_irq(unsigned int irq)
|
|
{
|
|
*sun3_intreg &= ~(1 << irq);
|
|
}
|
|
|
|
static irqreturn_t sun3_int7(int irq, void *dev_id)
|
|
{
|
|
unsigned int cnt;
|
|
|
|
cnt = kstat_irqs_cpu(irq, 0);
|
|
if (!(cnt % 2000))
|
|
sun3_leds(led_pattern[cnt % 16000 / 2000]);
|
|
return IRQ_HANDLED;
|
|
}
|
|
|
|
static irqreturn_t sun3_int5(int irq, void *dev_id)
|
|
{
|
|
unsigned long flags;
|
|
unsigned int cnt;
|
|
|
|
local_irq_save(flags);
|
|
#ifdef CONFIG_SUN3
|
|
intersil_clear();
|
|
#endif
|
|
sun3_disable_irq(5);
|
|
sun3_enable_irq(5);
|
|
#ifdef CONFIG_SUN3
|
|
intersil_clear();
|
|
#endif
|
|
xtime_update(1);
|
|
update_process_times(user_mode(get_irq_regs()));
|
|
cnt = kstat_irqs_cpu(irq, 0);
|
|
if (!(cnt % 20))
|
|
sun3_leds(led_pattern[cnt % 160 / 20]);
|
|
local_irq_restore(flags);
|
|
return IRQ_HANDLED;
|
|
}
|
|
|
|
static irqreturn_t sun3_vec255(int irq, void *dev_id)
|
|
{
|
|
return IRQ_HANDLED;
|
|
}
|
|
|
|
void __init sun3_init_IRQ(void)
|
|
{
|
|
*sun3_intreg = 1;
|
|
|
|
m68k_setup_user_interrupt(VEC_USER, 128);
|
|
|
|
if (request_irq(IRQ_AUTO_5, sun3_int5, 0, "clock", NULL))
|
|
pr_err("Couldn't register %s interrupt\n", "int5");
|
|
if (request_irq(IRQ_AUTO_7, sun3_int7, 0, "nmi", NULL))
|
|
pr_err("Couldn't register %s interrupt\n", "int7");
|
|
if (request_irq(IRQ_USER+127, sun3_vec255, 0, "vec255", NULL))
|
|
pr_err("Couldn't register %s interrupt\n", "vec255");
|
|
}
|