mirror of
https://github.com/brain-hackers/linux-brain.git
synced 2024-06-09 23:36:23 +09:00
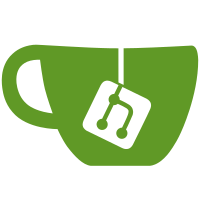
[ Upstream commit 141f8202cfa4192c3af79b6cbd68e7760bb01b5a ]
The ppos points to a position in the old kernel memory (and in case of
arm64 in the crash kernel since elfcorehdr is passed as a segment). The
function should update the ppos by the amount that was read. This bug is
not exposed by accident, but other platforms update this value properly.
So, fix it in ARM64 version of elfcorehdr_read() as well.
Signed-off-by: Pavel Tatashin <pasha.tatashin@soleen.com>
Fixes: e62aaeac42
("arm64: kdump: provide /proc/vmcore file")
Reviewed-by: Tyler Hicks <tyhicks@linux.microsoft.com>
Link: https://lore.kernel.org/r/20210319205054.743368-1-pasha.tatashin@soleen.com
Signed-off-by: Will Deacon <will@kernel.org>
Signed-off-by: Sasha Levin <sashal@kernel.org>
71 lines
1.7 KiB
C
71 lines
1.7 KiB
C
// SPDX-License-Identifier: GPL-2.0-only
|
|
/*
|
|
* Routines for doing kexec-based kdump
|
|
*
|
|
* Copyright (C) 2017 Linaro Limited
|
|
* Author: AKASHI Takahiro <takahiro.akashi@linaro.org>
|
|
*/
|
|
|
|
#include <linux/crash_dump.h>
|
|
#include <linux/errno.h>
|
|
#include <linux/io.h>
|
|
#include <linux/memblock.h>
|
|
#include <linux/uaccess.h>
|
|
#include <asm/memory.h>
|
|
|
|
/**
|
|
* copy_oldmem_page() - copy one page from old kernel memory
|
|
* @pfn: page frame number to be copied
|
|
* @buf: buffer where the copied page is placed
|
|
* @csize: number of bytes to copy
|
|
* @offset: offset in bytes into the page
|
|
* @userbuf: if set, @buf is in a user address space
|
|
*
|
|
* This function copies one page from old kernel memory into buffer pointed by
|
|
* @buf. If @buf is in userspace, set @userbuf to %1. Returns number of bytes
|
|
* copied or negative error in case of failure.
|
|
*/
|
|
ssize_t copy_oldmem_page(unsigned long pfn, char *buf,
|
|
size_t csize, unsigned long offset,
|
|
int userbuf)
|
|
{
|
|
void *vaddr;
|
|
|
|
if (!csize)
|
|
return 0;
|
|
|
|
vaddr = memremap(__pfn_to_phys(pfn), PAGE_SIZE, MEMREMAP_WB);
|
|
if (!vaddr)
|
|
return -ENOMEM;
|
|
|
|
if (userbuf) {
|
|
if (copy_to_user((char __user *)buf, vaddr + offset, csize)) {
|
|
memunmap(vaddr);
|
|
return -EFAULT;
|
|
}
|
|
} else {
|
|
memcpy(buf, vaddr + offset, csize);
|
|
}
|
|
|
|
memunmap(vaddr);
|
|
|
|
return csize;
|
|
}
|
|
|
|
/**
|
|
* elfcorehdr_read - read from ELF core header
|
|
* @buf: buffer where the data is placed
|
|
* @count: number of bytes to read
|
|
* @ppos: address in the memory
|
|
*
|
|
* This function reads @count bytes from elf core header which exists
|
|
* on crash dump kernel's memory.
|
|
*/
|
|
ssize_t elfcorehdr_read(char *buf, size_t count, u64 *ppos)
|
|
{
|
|
memcpy(buf, phys_to_virt((phys_addr_t)*ppos), count);
|
|
*ppos += count;
|
|
|
|
return count;
|
|
}
|