mirror of
https://github.com/brain-hackers/linux-brain.git
synced 2024-06-09 15:26:21 +09:00
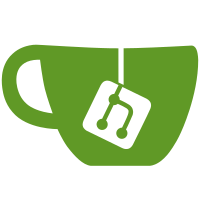
Based on 1 normalized pattern(s): this program is free software you can redistribute it and or modify it under the terms of the gnu general public license as published by the free software foundation either version 2 of the license or at your option any later version this program is distributed in the hope that it will be useful but without any warranty without even the implied warranty of merchantability or fitness for a particular purpose see the gnu general public license for more details you should have received a copy of the gnu general public license along with this program if not write to the free software foundation inc 675 mass ave cambridge ma 02139 usa extracted by the scancode license scanner the SPDX license identifier GPL-2.0-or-later has been chosen to replace the boilerplate/reference in 441 file(s). Signed-off-by: Thomas Gleixner <tglx@linutronix.de> Reviewed-by: Michael Ellerman <mpe@ellerman.id.au> (powerpc) Reviewed-by: Richard Fontana <rfontana@redhat.com> Reviewed-by: Allison Randal <allison@lohutok.net> Reviewed-by: Kate Stewart <kstewart@linuxfoundation.org> Cc: linux-spdx@vger.kernel.org Link: https://lkml.kernel.org/r/20190520071858.739733335@linutronix.de Signed-off-by: Greg Kroah-Hartman <gregkh@linuxfoundation.org>
110 lines
3.3 KiB
C
110 lines
3.3 KiB
C
/* SPDX-License-Identifier: GPL-2.0-or-later */
|
|
/*
|
|
NetWinder Floating Point Emulator
|
|
(c) Rebel.com, 1998-1999
|
|
|
|
Direct questions, comments to Scott Bambrough <scottb@netwinder.org>
|
|
|
|
*/
|
|
|
|
#ifndef __FPA11_H__
|
|
#define __FPA11_H__
|
|
|
|
#define GET_FPA11() ((FPA11 *)(¤t_thread_info()->fpstate))
|
|
|
|
/*
|
|
* The processes registers are always at the very top of the 8K
|
|
* stack+task struct. Use the same method as 'current' uses to
|
|
* reach them.
|
|
*/
|
|
#define GET_USERREG() ((struct pt_regs *)(THREAD_START_SP + (unsigned long)current_thread_info()) - 1)
|
|
|
|
#include <linux/thread_info.h>
|
|
|
|
/* includes */
|
|
#include "fpsr.h" /* FP control and status register definitions */
|
|
#include "milieu.h"
|
|
|
|
struct roundingData {
|
|
int8 mode;
|
|
int8 precision;
|
|
signed char exception;
|
|
};
|
|
|
|
#include "softfloat.h"
|
|
|
|
#define typeNone 0x00
|
|
#define typeSingle 0x01
|
|
#define typeDouble 0x02
|
|
#define typeExtended 0x03
|
|
|
|
/*
|
|
* This must be no more and no less than 12 bytes.
|
|
*/
|
|
typedef union tagFPREG {
|
|
float32 fSingle;
|
|
float64 fDouble;
|
|
#ifdef CONFIG_FPE_NWFPE_XP
|
|
floatx80 fExtended;
|
|
#else
|
|
u32 padding[3];
|
|
#endif
|
|
} __attribute__ ((packed,aligned(4))) FPREG;
|
|
|
|
/*
|
|
* FPA11 device model.
|
|
*
|
|
* This structure is exported to user space. Do not re-order.
|
|
* Only add new stuff to the end, and do not change the size of
|
|
* any element. Elements of this structure are used by user
|
|
* space, and must match struct user_fp in <asm/user.h>.
|
|
* We include the byte offsets below for documentation purposes.
|
|
*
|
|
* The size of this structure and FPREG are checked by fpmodule.c
|
|
* on initialisation. If the rules have been broken, NWFPE will
|
|
* not initialise.
|
|
*/
|
|
typedef struct tagFPA11 {
|
|
/* 0 */ FPREG fpreg[8]; /* 8 floating point registers */
|
|
/* 96 */ FPSR fpsr; /* floating point status register */
|
|
/* 100 */ FPCR fpcr; /* floating point control register */
|
|
/* 104 */ unsigned char fType[8]; /* type of floating point value held in
|
|
floating point registers. One of
|
|
none, single, double or extended. */
|
|
/* 112 */ int initflag; /* this is special. The kernel guarantees
|
|
to set it to 0 when a thread is launched,
|
|
so we can use it to detect whether this
|
|
instance of the emulator needs to be
|
|
initialised. */
|
|
} __attribute__ ((packed,aligned(4))) FPA11;
|
|
|
|
extern int8 SetRoundingMode(const unsigned int);
|
|
extern int8 SetRoundingPrecision(const unsigned int);
|
|
extern void nwfpe_init_fpa(union fp_state *fp);
|
|
|
|
extern unsigned int EmulateAll(unsigned int opcode);
|
|
|
|
extern unsigned int EmulateCPDT(const unsigned int opcode);
|
|
extern unsigned int EmulateCPDO(const unsigned int opcode);
|
|
extern unsigned int EmulateCPRT(const unsigned int opcode);
|
|
|
|
/* fpa11_cpdt.c */
|
|
extern unsigned int PerformLDF(const unsigned int opcode);
|
|
extern unsigned int PerformSTF(const unsigned int opcode);
|
|
extern unsigned int PerformLFM(const unsigned int opcode);
|
|
extern unsigned int PerformSFM(const unsigned int opcode);
|
|
|
|
/* single_cpdo.c */
|
|
|
|
extern unsigned int SingleCPDO(struct roundingData *roundData,
|
|
const unsigned int opcode, FPREG * rFd);
|
|
/* double_cpdo.c */
|
|
extern unsigned int DoubleCPDO(struct roundingData *roundData,
|
|
const unsigned int opcode, FPREG * rFd);
|
|
|
|
/* extneded_cpdo.c */
|
|
extern unsigned int ExtendedCPDO(struct roundingData *roundData,
|
|
const unsigned int opcode, FPREG * rFd);
|
|
|
|
#endif
|