mirror of
https://github.com/brain-hackers/linux-brain.git
synced 2024-06-09 07:16:21 +09:00
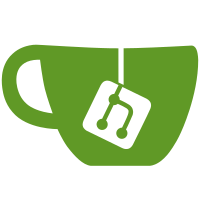
ARMv6 and greater introduced a new instruction ("bx") which can be used to return from function calls. Recent CPUs perform better when the "bx lr" instruction is used rather than the "mov pc, lr" instruction, and this sequence is strongly recommended to be used by the ARM architecture manual (section A.4.1.1). We provide a new macro "ret" with all its variants for the condition code which will resolve to the appropriate instruction. Rather than doing this piecemeal, and miss some instances, change all the "mov pc" instances to use the new macro, with the exception of the "movs" instruction and the kprobes code. This allows us to detect the "mov pc, lr" case and fix it up - and also gives us the possibility of deploying this for other registers depending on the CPU selection. Reported-by: Will Deacon <will.deacon@arm.com> Tested-by: Stephen Warren <swarren@nvidia.com> # Tegra Jetson TK1 Tested-by: Robert Jarzmik <robert.jarzmik@free.fr> # mioa701_bootresume.S Tested-by: Andrew Lunn <andrew@lunn.ch> # Kirkwood Tested-by: Shawn Guo <shawn.guo@freescale.com> Tested-by: Tony Lindgren <tony@atomide.com> # OMAPs Tested-by: Gregory CLEMENT <gregory.clement@free-electrons.com> # Armada XP, 375, 385 Acked-by: Sekhar Nori <nsekhar@ti.com> # DaVinci Acked-by: Christoffer Dall <christoffer.dall@linaro.org> # kvm/hyp Acked-by: Haojian Zhuang <haojian.zhuang@gmail.com> # PXA3xx Acked-by: Stefano Stabellini <stefano.stabellini@eu.citrix.com> # Xen Tested-by: Uwe Kleine-König <u.kleine-koenig@pengutronix.de> # ARMv7M Tested-by: Simon Horman <horms+renesas@verge.net.au> # Shmobile Signed-off-by: Russell King <rmk+kernel@arm.linux.org.uk>
50 lines
1.2 KiB
ArmAsm
50 lines
1.2 KiB
ArmAsm
/*
|
|
* linux/arch/arm/kernel/fiqasm.S
|
|
*
|
|
* Derived from code originally in linux/arch/arm/kernel/fiq.c:
|
|
*
|
|
* Copyright (C) 1998 Russell King
|
|
* Copyright (C) 1998, 1999 Phil Blundell
|
|
* Copyright (C) 2011, Linaro Limited
|
|
*
|
|
* FIQ support written by Philip Blundell <philb@gnu.org>, 1998.
|
|
*
|
|
* FIQ support re-written by Russell King to be more generic
|
|
*
|
|
* v7/Thumb-2 compatibility modifications by Linaro Limited, 2011.
|
|
*/
|
|
|
|
#include <linux/linkage.h>
|
|
#include <asm/assembler.h>
|
|
|
|
/*
|
|
* Taking an interrupt in FIQ mode is death, so both these functions
|
|
* disable irqs for the duration.
|
|
*/
|
|
|
|
ENTRY(__set_fiq_regs)
|
|
mov r2, #PSR_I_BIT | PSR_F_BIT | FIQ_MODE
|
|
mrs r1, cpsr
|
|
msr cpsr_c, r2 @ select FIQ mode
|
|
mov r0, r0 @ avoid hazard prior to ARMv4
|
|
ldmia r0!, {r8 - r12}
|
|
ldr sp, [r0], #4
|
|
ldr lr, [r0]
|
|
msr cpsr_c, r1 @ return to SVC mode
|
|
mov r0, r0 @ avoid hazard prior to ARMv4
|
|
ret lr
|
|
ENDPROC(__set_fiq_regs)
|
|
|
|
ENTRY(__get_fiq_regs)
|
|
mov r2, #PSR_I_BIT | PSR_F_BIT | FIQ_MODE
|
|
mrs r1, cpsr
|
|
msr cpsr_c, r2 @ select FIQ mode
|
|
mov r0, r0 @ avoid hazard prior to ARMv4
|
|
stmia r0!, {r8 - r12}
|
|
str sp, [r0], #4
|
|
str lr, [r0]
|
|
msr cpsr_c, r1 @ return to SVC mode
|
|
mov r0, r0 @ avoid hazard prior to ARMv4
|
|
ret lr
|
|
ENDPROC(__get_fiq_regs)
|