mirror of
https://github.com/brain-hackers/linux-brain.git
synced 2024-06-09 07:16:21 +09:00
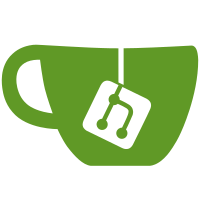
Simplify the timerqueue code by using cached rbtrees and rely on the tree leftmost node semantics to get the timer with earliest expiration time. This is a drop in conversion, and therefore semantics remain untouched. The runtime overhead of cached rbtrees is be pretty much the same as the current head->next method, noting that when removing the leftmost node, a common operation for the timerqueue, the rb_next(leftmost) is O(1) as well, so the next timer will either be the right node or its parent. Therefore no extra pointer chasing. Finally, the size of the struct timerqueue_head remains the same. Passes several hours of rcutorture. Signed-off-by: Davidlohr Bueso <dbueso@suse.de> Signed-off-by: Thomas Gleixner <tglx@linutronix.de> Link: https://lkml.kernel.org/r/20190724152323.bojciei3muvfxalm@linux-r8p5
95 lines
2.5 KiB
C
95 lines
2.5 KiB
C
// SPDX-License-Identifier: GPL-2.0-or-later
|
|
/*
|
|
* Generic Timer-queue
|
|
*
|
|
* Manages a simple queue of timers, ordered by expiration time.
|
|
* Uses rbtrees for quick list adds and expiration.
|
|
*
|
|
* NOTE: All of the following functions need to be serialized
|
|
* to avoid races. No locking is done by this library code.
|
|
*/
|
|
|
|
#include <linux/bug.h>
|
|
#include <linux/timerqueue.h>
|
|
#include <linux/rbtree.h>
|
|
#include <linux/export.h>
|
|
|
|
/**
|
|
* timerqueue_add - Adds timer to timerqueue.
|
|
*
|
|
* @head: head of timerqueue
|
|
* @node: timer node to be added
|
|
*
|
|
* Adds the timer node to the timerqueue, sorted by the node's expires
|
|
* value. Returns true if the newly added timer is the first expiring timer in
|
|
* the queue.
|
|
*/
|
|
bool timerqueue_add(struct timerqueue_head *head, struct timerqueue_node *node)
|
|
{
|
|
struct rb_node **p = &head->rb_root.rb_root.rb_node;
|
|
struct rb_node *parent = NULL;
|
|
struct timerqueue_node *ptr;
|
|
bool leftmost = true;
|
|
|
|
/* Make sure we don't add nodes that are already added */
|
|
WARN_ON_ONCE(!RB_EMPTY_NODE(&node->node));
|
|
|
|
while (*p) {
|
|
parent = *p;
|
|
ptr = rb_entry(parent, struct timerqueue_node, node);
|
|
if (node->expires < ptr->expires) {
|
|
p = &(*p)->rb_left;
|
|
} else {
|
|
p = &(*p)->rb_right;
|
|
leftmost = false;
|
|
}
|
|
}
|
|
rb_link_node(&node->node, parent, p);
|
|
rb_insert_color_cached(&node->node, &head->rb_root, leftmost);
|
|
|
|
return leftmost;
|
|
}
|
|
EXPORT_SYMBOL_GPL(timerqueue_add);
|
|
|
|
/**
|
|
* timerqueue_del - Removes a timer from the timerqueue.
|
|
*
|
|
* @head: head of timerqueue
|
|
* @node: timer node to be removed
|
|
*
|
|
* Removes the timer node from the timerqueue. Returns true if the queue is
|
|
* not empty after the remove.
|
|
*/
|
|
bool timerqueue_del(struct timerqueue_head *head, struct timerqueue_node *node)
|
|
{
|
|
WARN_ON_ONCE(RB_EMPTY_NODE(&node->node));
|
|
|
|
rb_erase_cached(&node->node, &head->rb_root);
|
|
RB_CLEAR_NODE(&node->node);
|
|
|
|
return !RB_EMPTY_ROOT(&head->rb_root.rb_root);
|
|
}
|
|
EXPORT_SYMBOL_GPL(timerqueue_del);
|
|
|
|
/**
|
|
* timerqueue_iterate_next - Returns the timer after the provided timer
|
|
*
|
|
* @node: Pointer to a timer.
|
|
*
|
|
* Provides the timer that is after the given node. This is used, when
|
|
* necessary, to iterate through the list of timers in a timer list
|
|
* without modifying the list.
|
|
*/
|
|
struct timerqueue_node *timerqueue_iterate_next(struct timerqueue_node *node)
|
|
{
|
|
struct rb_node *next;
|
|
|
|
if (!node)
|
|
return NULL;
|
|
next = rb_next(&node->node);
|
|
if (!next)
|
|
return NULL;
|
|
return container_of(next, struct timerqueue_node, node);
|
|
}
|
|
EXPORT_SYMBOL_GPL(timerqueue_iterate_next);
|