mirror of
https://github.com/brain-hackers/linux-brain.git
synced 2024-06-09 15:26:21 +09:00
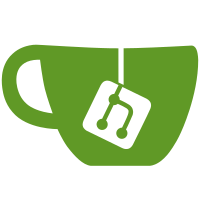
The hrtimer code is interested whether the added timer is the first one to expire and whether the removed timer was the last one in the tree. The add/del routines have that information already. So we can return it right away instead of reevaluating it at the call site. Signed-off-by: Thomas Gleixner <tglx@linutronix.de> Acked-by: Peter Zijlstra <peterz@infradead.org> Cc: Preeti U Murthy <preeti@linux.vnet.ibm.com> Cc: Viresh Kumar <viresh.kumar@linaro.org> Cc: Marcelo Tosatti <mtosatti@redhat.com> Cc: Frederic Weisbecker <fweisbec@gmail.com> Cc: John Stultz <john.stultz@linaro.org> Link: http://lkml.kernel.org/r/20150414203501.579063647@linutronix.de Signed-off-by: Thomas Gleixner <tglx@linutronix.de>
113 lines
3.2 KiB
C
113 lines
3.2 KiB
C
/*
|
|
* Generic Timer-queue
|
|
*
|
|
* Manages a simple queue of timers, ordered by expiration time.
|
|
* Uses rbtrees for quick list adds and expiration.
|
|
*
|
|
* NOTE: All of the following functions need to be serialized
|
|
* to avoid races. No locking is done by this library code.
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, write to the Free Software
|
|
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
|
|
*/
|
|
|
|
#include <linux/bug.h>
|
|
#include <linux/timerqueue.h>
|
|
#include <linux/rbtree.h>
|
|
#include <linux/export.h>
|
|
|
|
/**
|
|
* timerqueue_add - Adds timer to timerqueue.
|
|
*
|
|
* @head: head of timerqueue
|
|
* @node: timer node to be added
|
|
*
|
|
* Adds the timer node to the timerqueue, sorted by the
|
|
* node's expires value.
|
|
*/
|
|
bool timerqueue_add(struct timerqueue_head *head, struct timerqueue_node *node)
|
|
{
|
|
struct rb_node **p = &head->head.rb_node;
|
|
struct rb_node *parent = NULL;
|
|
struct timerqueue_node *ptr;
|
|
|
|
/* Make sure we don't add nodes that are already added */
|
|
WARN_ON_ONCE(!RB_EMPTY_NODE(&node->node));
|
|
|
|
while (*p) {
|
|
parent = *p;
|
|
ptr = rb_entry(parent, struct timerqueue_node, node);
|
|
if (node->expires.tv64 < ptr->expires.tv64)
|
|
p = &(*p)->rb_left;
|
|
else
|
|
p = &(*p)->rb_right;
|
|
}
|
|
rb_link_node(&node->node, parent, p);
|
|
rb_insert_color(&node->node, &head->head);
|
|
|
|
if (!head->next || node->expires.tv64 < head->next->expires.tv64) {
|
|
head->next = node;
|
|
return true;
|
|
}
|
|
return false;
|
|
}
|
|
EXPORT_SYMBOL_GPL(timerqueue_add);
|
|
|
|
/**
|
|
* timerqueue_del - Removes a timer from the timerqueue.
|
|
*
|
|
* @head: head of timerqueue
|
|
* @node: timer node to be removed
|
|
*
|
|
* Removes the timer node from the timerqueue.
|
|
*/
|
|
bool timerqueue_del(struct timerqueue_head *head, struct timerqueue_node *node)
|
|
{
|
|
WARN_ON_ONCE(RB_EMPTY_NODE(&node->node));
|
|
|
|
/* update next pointer */
|
|
if (head->next == node) {
|
|
struct rb_node *rbn = rb_next(&node->node);
|
|
|
|
head->next = rbn ?
|
|
rb_entry(rbn, struct timerqueue_node, node) : NULL;
|
|
}
|
|
rb_erase(&node->node, &head->head);
|
|
RB_CLEAR_NODE(&node->node);
|
|
return head->next != NULL;
|
|
}
|
|
EXPORT_SYMBOL_GPL(timerqueue_del);
|
|
|
|
/**
|
|
* timerqueue_iterate_next - Returns the timer after the provided timer
|
|
*
|
|
* @node: Pointer to a timer.
|
|
*
|
|
* Provides the timer that is after the given node. This is used, when
|
|
* necessary, to iterate through the list of timers in a timer list
|
|
* without modifying the list.
|
|
*/
|
|
struct timerqueue_node *timerqueue_iterate_next(struct timerqueue_node *node)
|
|
{
|
|
struct rb_node *next;
|
|
|
|
if (!node)
|
|
return NULL;
|
|
next = rb_next(&node->node);
|
|
if (!next)
|
|
return NULL;
|
|
return container_of(next, struct timerqueue_node, node);
|
|
}
|
|
EXPORT_SYMBOL_GPL(timerqueue_iterate_next);
|